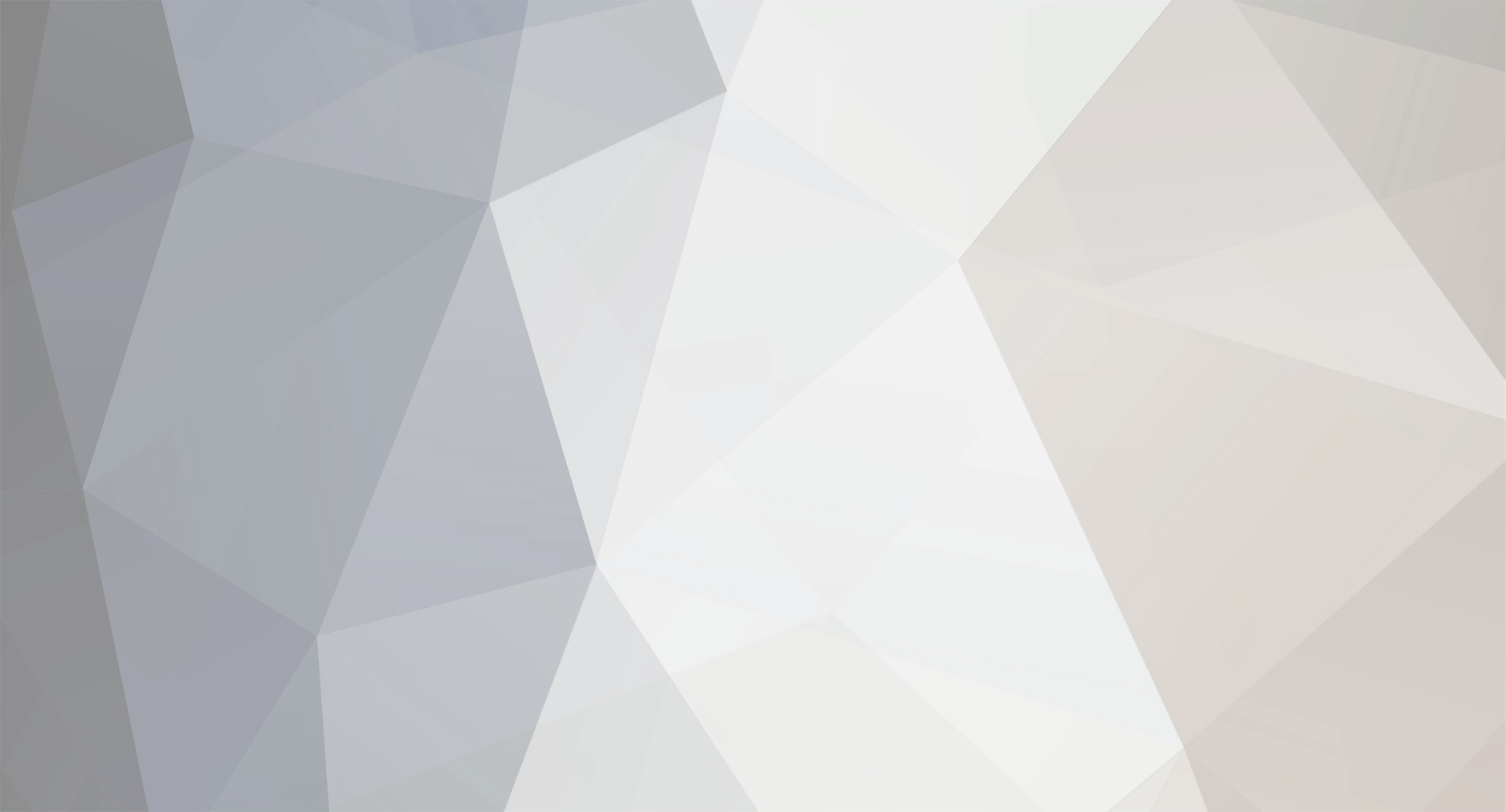
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[1.6.4] Client server issue regarding LAN
coolAlias replied to SenpaiSubaraki's topic in Modder Support
Why are you using the render bounding box instead of a regular bounding box? If this is in a client-only class, then try sending a packet to the server telling it to search for entities instead. -
The vanilla explosion class has a lot of hard-coded behaviors, for example, the maximum limit it will search for blocks is limited to 16, though increasing the radius should certainly affect how many blocks get destroyed. Try your explosion again without the offset position and see if you don't blast a giant hole in the ground. If not, you may need to write your own custom explosion code to get the behavior you need.
-
[1.6.4] add item to inventory if item is on top op the bl0ck
coolAlias replied to denbukki's topic in Modder Support
Do you have a TileEntity for your block? If so, you can check for EntityItems at the position above your block on the te's updateEntity tick. I would only check every 20-50 ticks or so unless you need it to be speedy for some reason. You can use World.getEntitiesWithinAABB(EntityItem.class, AxisAlignedBB) to get all the entity items within a certain bounding box (which you must define). Just look at the classes / methods involved and you will see how they work. -
Ah, man, you're right about that! Totally forgot about Float vs. float >.< Thanks. And I'll look into the EnumMap as well - good suggestion.
-
[1.6.4] How to make an entity follow the player?
coolAlias replied to osum4est's topic in Modder Support
All the code you need is in any of the passive mobs that can be tamed (all of which extend EntityTameable). As suggested, if you look in EntityWolf and EntityTameable, you can find the code you need. You will not be able to easily make the vanilla pig tameable, but you can make a class that extends EntityPig and replace the vanilla pig with your custom pig when you 'tame' the pig. -
I'll let the code do most of the talking on this one: http://pastebin.com/rERjziBM I'm trying to construct different EntityArrow types based on whatever arrow is nocked; currently I'm just using the vanilla arrow. As you can see in the code, I've done it before with mobs which works very well, using the same style code but the constructor with only a single World object argument. Using the three argument EntityArrow constructor, however, seems to always return null without throwing any exceptions, and the arrow id and class are correct according to my println statements. Thanks for any help.
-
[1.6.4] Client server issue regarding LAN
coolAlias replied to SenpaiSubaraki's topic in Modder Support
How is AABB client-side only? Neither the method World.getEntitiesWithinAABB nor the AxisAlignedAABB class itself are client-side only... what are you using? Perhaps posting the specific code would make it more clear. -
In your mob's class, override the getCanSpawnHere() method: @Override public boolean getCanSpawnHere() { return super.getCanSpawnHere() && yourMethodForIsEntityUnderground(); } You can either write your own method or look for a vanilla one to determine if the entity can see the sky (i.e. is underground or not). Since it is an entity method, you have access to 'this.worldObj' and 'this.posX/Y/Z'.
-
Witch. Where is calculated what kind of potion it attacks?
coolAlias replied to Kwasti's topic in Modder Support
Try checking the wiki. That only works for potions that actually exist in the game, though. For other potion effects such as blindness, you need to create a new PotionEffect with the correct id, duration, and amplifier and add that effect to the entity. You won't be able to throw a potion with that effect though, unless you create a custom potion. -
That would be because your mod's id, according to your console output, is "examplemod", but your folder is named "modid". They need to match.
-
Can I have the game detect if a player just spawned?
coolAlias replied to MINERGUY67880's topic in Modder Support
The reason he is suggesting using IPlayerTracker is because it is only called for players, whereas EntityJoinWorldEvent gets called for EVERY single entity, including thrown items, mobs, etc., so you are doing a lot of wasteful processing if you only care about instances of EntityPlayer. -
Can I have the game detect if a player just spawned?
coolAlias replied to MINERGUY67880's topic in Modder Support
Have you used Forge Events before? If not, this may be helpful. Look in net.minecraftforge.event and you will see all the different kinds of events available. The one you are looking for is called EntityJoinWorldEvent. You could also implement an IPlayerTracker, which has methods to handle login and spawn. -
Try this: // our entity class: public class EntityExperiment extends EntitySquid { public EntityExperiment(World world) { super(world); } } // no other methods, just plain old inheritance at work here // entity is registered normally // no render class is registered, as it uses RenderSquid automatically When spawning this sub-class of EntitySquid, it renders normally and has the regular tentacle behavior, but for some reason, and unlike the vanilla squids, it never changes its head direction, so the head is always pointing straight up making it look like a weird fishing bobber suddenly moving in various directions. Does anyone have any idea why this entity would fail to exhibit all of the regular squid's behavior? I've looked through all the parent classes for any references to 'squid' to no avail, nor did I find any in the various render classes.
-
You are declaring a new ItemStack in your eventhandler and checking if the item the player is using is that new ItemStack... what did you expect to happen? How could the player possibly be using an ItemStack stored in your EventHandler class??? EDIT: of course, using ItemStacks.areEqual method should still work for detecting if two itemstacks have the same item, but if your player's wooden axe is damaged at all, it will not be considered equal to the undamaged stack from your event handler. You need to check if the Item that the player is using is some kind of Axe and create a new thrown entity based off of, oh I don't know, the axe's material or something. // let me preface by saying that I don't have 1.7.2, so I am not sure exactly what the variables are called // but the logic will be the same // check if the Item is an Axe (if Item is really an ItemStack, then you'd use event.item.getItem()) if (event.item instanceof ItemAxe) { // assuming there is a player available from the event // and assuming you have an EntityThrowable that takes a world and player object in the constructor // then the following will set the position and heading automatically EntityThrowingAxe axe = new EntityThrowingAxe(event.player.worldObj, event.player); // now let's assume you created a method in your throwing axe entity to parse through items and get // the correct material, or you could just get the AttackDamage attribute modifier from the Item's // multimap and set the damage that way axe.setMaterial(event.item); // then spawn it into the world if (!event.player.worldObj.isRemote) { event.player.worldObj.spawnEntityInWorld(axe); } } Obviously the code will probably not work exactly as written, but that is what you need to do (logically speaking).
-
Just because the Item is final doesn't mean you can't change what the player is doing with it. You want right-click to throw, so when the UseEvent fires, check if the item is an axe and, if so, spawn a new EntityThrowingAxe or whatever into the world and set the player's currently held item slot to null. Make sure to cancel the event if possible so you don't subsequently throw a null-pointer exception.
-
I may be wrong as I haven't modded for 1.7 yet myself, but I believe they changed it to '@SubscribeEvent'.
-
[1.6.4] Creating new chest problem......texture {Solved}
coolAlias replied to ASHninja1997's topic in Modder Support
Without the full crash log, it's difficult to say, but I'm guessing that your TESR is trying to use something from the TileEntity, which of course will not be available when you are viewing the block in the inventory. That's why IItemRenderer and the like render Items, not TileEntities. I'm guessing you must not have looked at the link I posted earlier. Anyway, this is how I render my custom chest in the inventory, using the ISimpleBlockRenderingHandler. Of course you need to register it: RenderingRegistry.registerBlockHandler(new RenderChestLocked()); And in your Block class, return the appropriate render type: @Override public int getRenderType() { return RenderChestLocked.renderId; } That's it. This will not adversely affect your TESR either, which is responsible for rendering the block when it is actually in the world, so long as you set it up correctly in the first place. Here is my TESR for my custom chest. -
Yeah, that's me Glad you found those things helpful. What Draco suggested is also very useful, using raycasting or following the vector yourself onItemRightClick can get you the entity/block directly, rather than spawning an entity. Either way works, but I usually go with the raytracing in cases where I don't need anything rendering on the screen (e.g. when I don't have a projectile to render, trailing particles, that kind of thing).
-
If your block isn't using metadata for anything other than your rendering, why not just use the block's metadata value directly as stored in the world? world.setBlockMetatata(x, y, z, meta), then in your rendering class world.getBlockMetadata(x, y, z) done, you've got the index of the item responsible. If that doesn't work for you for whatever reason, store the value as a field in the tile entity (making sure to save it in the tile entity's write / read NBT methods) and override the getDescriptionPacket method. @Override public void writeToNBT(NBTTagCompound compound) { super.writeToNBT(compound); compound.setInteger("key", value); } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); yourField = compound.getInteger("key"); } // now this will automatically synchronize your TileEntity from server to client: @Override public Packet getDescriptionPacket() { NBTTagCompound tag = new NBTTagCompound(); this.writeToNBT(tag); return new Packet132TileEntityData(xCoord, yCoord, zCoord, 1, tag); } // combined with this: @Override public void onDataPacket(INetworkManager net, Packet132TileEntityData packet) { readFromNBT(packet.data); } From the sounds of it, you are simply missing the synchronization part.
-
No one ever does it because the NBT tag for tile entities is only used to store the data between quitting and restarting the world, unlike that for ItemStacks which is often modified and referenced during the course of the game. Once the tile entity loads, all the data should be stored in local fields, which you can print normally for debugging. If you really want to see what is in the NBT tag, then put your debugging messages in the read / write NBT methods.
-
[1.6.4] Creating new chest problem......texture {Solved}
coolAlias replied to ASHninja1997's topic in Modder Support
Yes, just use a TESR like this. That works just fine with the vanilla chest render id 22, except you will get a chest rendering in the inventory rather than your custom texture. Looks like you already took care of that though. Be sure to register your TESR on the client side: ClientRegistry.bindTileEntitySpecialRenderer(TileEntityChestLocked.class, new RenderTileEntityChestLocked()); -
Lol, oh man, that totally slipped by me! Sorry I just assumed you were using LivingHurtEvent... mybad
-
@diesieben07 True, but I've had (reported by users with other mods loaded in addition to mine, so...) class cast exceptions thrown when accessing tile entities like that, so I throw in that extra check for good measure.