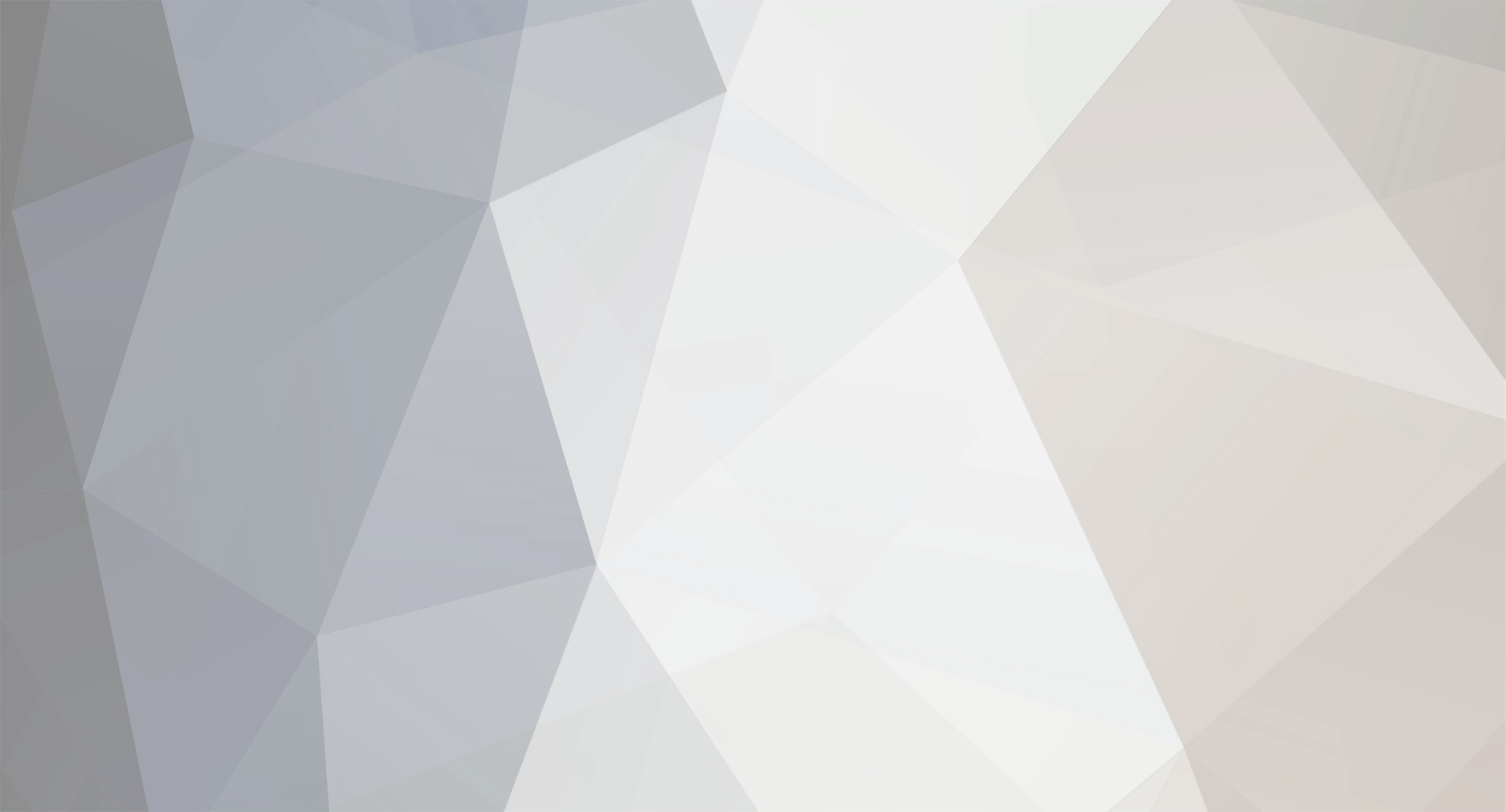
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
He said this is singleplayer. That would still only give you the client player, and would crash the mod if played on a server. There is absolutely no reason at all to use Minecraft.getMinecraft() in this case, single player or not.
-
Well, lucky for you then that you don't even need to make your own: ((EntityPlayerMP) player).playerNetServerHandler.sendPacket(new S39PacketPlayerAbilities(player.capabilities));
-
That's because the client side version of the player doesn't know he can fly - it wouldn't work in multiplayer, either. Send a packet to update the client player's capabilities when you change them (there is a vanilla packet for this).
-
Exactly the same. Single player has the integrated server which uses EntityPlayerMP just like the dedicated server. Nowadays the vast majority of code does not need to change whether in single or multi player.
-
Here is the constructor I created for my slingshot that has scattering ability. With that, when you want to spawn your entities, just use a for loop with that constructor and spawn each into the world as it is created.
-
That's because PlayerTickEvent happens on BOTH sides - always type check before casting: if (event.player instanceof EntityPlayerMP) { EntityPlayerMP playerMP = (EntityPlayerMP) event.player; // rest of code relying on playerMP still within condition }
-
Unfortunately, you can't get much more instantaneous than every single tick in Minecraft. Tick events have a Phase.START and Phase.END, so they happen twice per tick. Use the START phase to change movement speed so it affects the player's movement in that particular update tick, or during the END phase to change it for the next tick. To make the code nice, you could make a Map of Block Material type to movement speed modifier, then add and remove AttributeModifiers for SharedMonsterAttribute.movementSpeed to the player: public static final UUID blockMovementModifierUUID = UUID.fromString("PutSomethingInHere: see http://createguid.com/"); // every tick during Phase.START: IAttributeInstance movement = player.getEntityAttribute(SharedMonsterAttributes.movementSpeed); // remove old: AttributeModifier old = getModifier(blockMovementModifierUUID); if (old != null) { movement.removeModifier(old); } // Add new: double value = yourMap.get(currentBlock.getMaterial()); // one should check if map contains value first // Attribute Modifiers have 3 [url=http://minecraft.gamepedia.com/Attributes#Operations]operations[/url]: 0 - additive, 1 - multiplicative, 2 - 1+value multiplicative AttributeModifier modifier = (new AttributeModifier(blockMovementModifierUUID, "Block Movement Modifier", value, 1)).setSaved(false); movement.applyModifier(modifier);
-
Run 'gradlew clean', refresh your workspace, then try again. Sometimes the workspace does not register changes you make if you make them in Windows Explorer - for example, if I am browsing a folder and change a texture name, Eclipse still thinks it's the old name until I tell it to refresh. I've never had it affect the actual build, though... worth a try I suppose.
-
Sounds like you may have a problem with how you are storing the player. At any rate, I have an item in one of my mods that increases the player's step height - I do so only on the client side and it works fine. You should try toggling the step height directly on the client (you don't need any update event to mess with this): @SubscribeEvent public void onKeyInput(KeyInputEvent event) { Minecraft mc = Minecraft.getMinecraft(); // or store the instance as a class field if (Keyboard.getEventKeyState() && Keyboard.getEventKey() == mc.gameSettings.keyBindWhatever.getKeyCode()) { mc.thePlayer.stepHeight += (yourToggleFlag ? 1.0F : -1.0F); } }
-
Your build.gradle looks totally fine. Have you tried using an earlier version of Forge 1.8? I've been using 1.8-11.14.0.1281-1.8 with those mappings and the jar builds and runs with no issues.
-
[Solved][1.7.10]Why is this check for armor always returning true?
coolAlias replied to jabelar's topic in Modder Support
LOL!!! Nice one. Well, I DID copy / paste your code to make sure I could replicate the problem, and... <smacks forehead>. Personally, I usually only use a newline before a brace in my class declarations; everywhere else they get inlined. -
[1.7.2]About Swing speed/ Attack Speed/AttackDelay
coolAlias replied to aguinmox's topic in Modder Support
See EntityLivingBase#getArmSwingAnimationEnd(). It's easy to find if you highlight the digSlowdown Potion and open its Call Hierarchy (Ctrl + Alt + H in Eclipse). -
[Solved][1.7.10]Why is this check for armor always returning true?
coolAlias replied to jabelar's topic in Modder Support
It works when wearing foot gear, but when the stack is null, there is inexplicably still console output despite the 'if (stack != null)' condition. It even prints that the stack is still null within the condition!!! I'm truly stumped on this one :\ -
[Solved][1.7.10]Why is this check for armor always returning true?
coolAlias replied to jabelar's topic in Modder Support
That's pretty bizarre. What do you get if you put 'System.out.println("CurrentArmor(0): " + thePlayer.getCurrentArmor(0));' ? EDIT: Just tried it myself, and it is completely inexplicable: if (!event.entityLiving.worldObj.isRemote && event.entityLiving instanceof EntityPlayer) { EntityPlayer thePlayer = (EntityPlayer) event.entityLiving; ItemStack stack = thePlayer.getCurrentArmor(0); System.out.println("Stack = " + stack); if (stack != null && stack.getItem() == ZSSItems.bootsHeavy); { // DEBUG System.out.println("LivingFallEvent handled due to having safe falling armor equipped: " + stack); event.distance = 0.0F ; } } Output: [14:59:58] [server thread/INFO] [sTDOUT]: [zeldaswordskills.handler.ZSSEntityEvents:onFall:78]: Stack = null [14:59:58] [server thread/INFO] [sTDOUT]: [zeldaswordskills.handler.ZSSEntityEvents:onFall:82]: LivingFallEvent handled due to having safe falling armor equipped: null -
[Solved][1.7.10]Why is this check for armor always returning true?
coolAlias replied to jabelar's topic in Modder Support
You should never need to check if an ItemStack's getItem() method returns null - if the stack itself is not null, it is guaranteed to have a valid Item. Can you show your full LivingFallEvent code? (another btw, LivingFallEvent is not posted in creative mode - for that, you must also subscribe to PlayerFlyableFallEvent). -
[Solved][1.7.10]Why is this check for armor always returning true?
coolAlias replied to jabelar's topic in Modder Support
For Items, it is much more efficient to check identity: ItemStack stack = thePlayer.getCurrentArmor(0); if (stack != null && stack.getItem() == MagicBeans.bootsOfSafeFalling) { System.out.println(stack.getDisplayName() + " equipped."); } If I may ask, where are you getting 'thePlayer' from, and in what context is your code being run (e.g. Item#onUpdate, packet, etc.) ? -
It really depends. If you have your own IInventory implementation like you showed below, then the case of items changing is already handled and you shouldn't need to loop at all. Each time a slot changes, you call equip or unequip for the item which modifies values in the player's extended properties (much better than writing to NBT each time), then you just get the value from the extended properties rather than using getEntityData().getWhateverValue("something"). That would be about as efficient as you could get, not involving any loops or disk I/O, at least for managing the player's current buffs/debuffs. Btw, in your setInventorySlotContents implementation, you should check first if the current stack in the slot is not null and call onUnequipped for it, otherwise you could easily end up with permanent buffs/debuffs.
-
PlayerLoggedInEvent happens for every player that logs in to the server, though, so if you do a direct assignation, you would only ever be able to return one player out of all the players online. Might explain why you are having trouble getting it to work multiplayer, though I thought that the player's step height only mattered on the client.
-
If your TileEntity implements IInventory, then that is your custom inventory class.
-
It's always false because you only set its value on the server side (or possibly getAttackTarget is always null on the client). Anyway, you don't want to use static as you already discovered; instead, you need to send a packet to the client with the current state of the 'isShooting' field for the given entity. Alternatively, you can store the boolean value in DataWatcher instead, which will handle the packet bit for you.
-
No, your GuiHandler looks fine, and the proxies shouldn't have anything to do with it. Are you doing anything out of the ordinary in the Gui class? For reference, this is all you need for a functional container-based gui class. How about your custom IInventory class, anything funky in there? Otherwise, nothing really comes to mind. You do have a lot of unused and unnecessary code in your Container, stuff like: // using loops for one iteration: for(int i = 0; i < 1; i++) { for(int j = 0; j < 1; j++) { } } // unused variables: Slot inSlot = (Slot) this.inventorySlots.get(0); Slot outSlot = (Slot) this.inventorySlots.get(1);
-
[1.7.10] Adding Custom Villager trades to a new Villager
coolAlias replied to xchow's topic in Modder Support
Your entity is a vanilla EntityVillager with a specific profession ID. So spawn a villager and set its profession. -
[1.7.10] Adding Custom Villager trades to a new Villager
coolAlias replied to xchow's topic in Modder Support
If you don't want to add a custom structure to the village, an alternate (and pretty hacky) way of doing it is to hitch a ride on the regular villagers using EntityJoinWorldEvent. Check if the event.entity.getClass().isAssignableFrom(EntityVillager.class) to make sure you only target vanilla villagers (and not every subclass thereof), then you could cast to EntityVillager and make sure the profession was vanilla as well, if that's important to you. Then, so you don't spawn with every single villager, do a rand check like "if (event.entity.worldObj.rand.nextFloat() < 0.1F)" for 1 of your villagers per 10 regular, on average, and spawn one in at the same location as the other villager. -
A common source of ghost itemstacks is not returning the appropriate server-side gui element in your IGuiHandler. Can you show us that class?
-
[1.8] SimpleNetworkWrapper & io.netty.handler.codec.DecoderException
coolAlias replied to techstack's topic in Modder Support
Interesting. I haven't tried using sendToAllAround in a multiplayer environment yet, so that's good to know. Thanks for reporting your results, and hopefully it will get fixed soon.