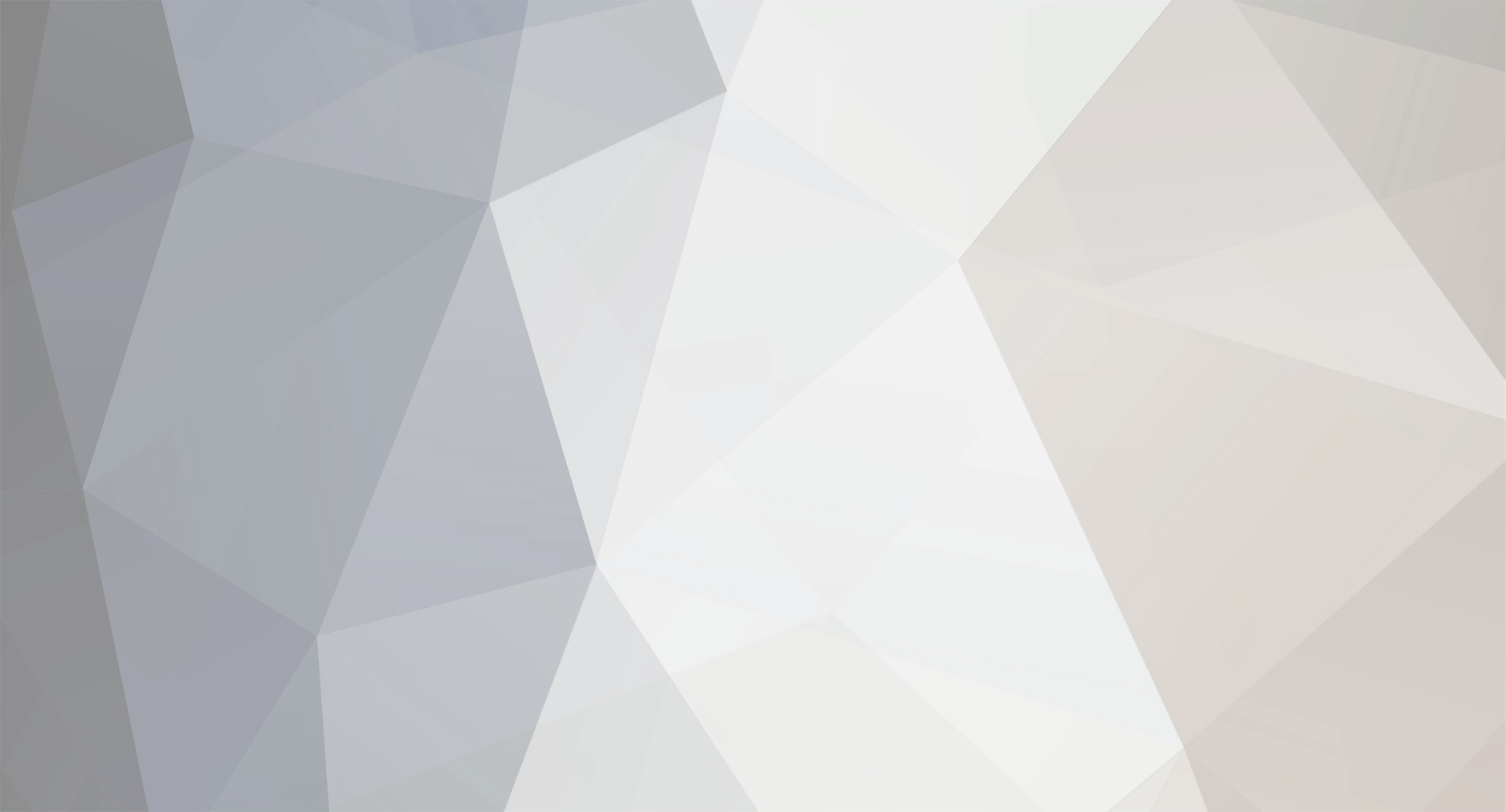
Jacky2611
Members-
Posts
499 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Jacky2611
-
I am trying to get meta items working in 1.12. Right now I have a basic meta item setup and use the following code to register my item render: public static void registerItemRenderer() { for(ItemBasic item : ItemRegistry.items) { register(item); } } public static void register(ItemBasic item) { //normal items if(!item.getHasSubtypes()) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher() .register(item, 0, new ModelResourceLocation(item.getRegistryName(), "inventory")); } else { NonNullList<ItemStack> list = NonNullList.create(); item.getSubItems(null, list); for(ItemStack stack : list) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher() .register(item, stack.getItemDamage(), new ModelResourceLocation(item.getRegistryName(), "inventory")); } } } which makes sure that my items have the same texture my main item had. The problem is that I have no idea how I can get variants working inside an items json file.
-
There is a warp command? What does it do? Or are you playing on a bukkit server? Cause some bukkit servers don't allow clients with forge to join.
-
If you are an admin on the server you could also just install a server side only mod. Clients could still connect to your server without the mod and you could do a lot more stuff without having to go through vanilla commands. Kinda like bukkit but better and more awesome.
-
Fun things: one of the things at the top of my possible projects list is a model json maker. But writing a really good ui and figuring out the eclipse api would be so boring...
-
Nah, we are perfectly happy helping you as long as the best answer gets a like. And it's not like I have anything better to do. My own mods usually run out of ideas or I get stuck in some really boring part of development. (like writing jsons. ugh)
-
What exactly is this supposed to do, where is it, and could you please rename posNumber to pos? Show us more code. Preferably a GitHub repo.
-
One of the inits. Not sure which one though. Edit: preinit And thx, your video just gave me an idea on how to solve one of my own problems. (Without overloading the server) And do you need something to teleport you to your dimension?
-
OK, I just realized that days don't have to be 24000 ticks long in all dimensions. (I am way too tired for this) Which means that my code above is mostly useless should I ever want to use it somewhere else since there is no way to figure out how many ticks are in another dimension sun cycle. What I could do instead is to trigger a new day whenever the isDaytime boolean for a world changes. If I combine that with my current approach (in order to notice should someone just skip an entire day/night) my system should be (mostly) foolproof. EDIT: Even /time set day sets it back down to 0. And users don't even have to use commands. As soon as another mod starts messing around with time I am screwed. And as I already said above, I can't rely on the tick count because not all dimension have to have the same day cycle. What I did now is to rely on the world#isDaytime boolean to catch normal day changes while also triggering a change should someone mess around with the server time. This is what my sleep deprived mind came up with. public void updateCurrentTime(long currentTime, boolean currentDay) { //check if someone used a command to reset time or if the night/day switched to day if((currentTime<this.lastTime) || ((this.isDay!=currentDay))&¤tDay) this.addDay(); this.lastTime=currentTime; this.isDay = currentDay; } And did I write my reply above in camel case o.O?
-
Well, that's the funny thing about it. I also thought that it would be in the range of 0-23999 ticks, but apparently it keeps counting up in most cases. I have to get the long back into the range on my own to make my own code work. here is what I wrote to get a proper day count for my worlds. I call updateCurrentTime all 200 ticks from inside a world tick event with the worlds current time.
-
Interesting. It looks like sleepingDoesntResetTheTimeCounter. But as soon as someone does use a command we are back to 0. Looks like I have to write my own solution.
-
Are you sure @Draco18s? I just tried that in a world and whenever I changed the time of day it had absolutely no effect on getTotalWorldTime.
-
Wait, that does account for sleeping and stuff like that? I thought that was the real time spent in the world.
-
Quick question, is there any way to get a worlds age in days? I know that I can use getTotalWorldTime() to get the real time the world has existed, but that totally ignores sleeping and cheating admins. Right now I am thinking about using the world tick event to check all 100 ticks if the world time is smaller than it was the last time I checked, and if yes increase the worlds day counter by one. Is there any better solution? Andwould the overworlds time keep running if all my players are in a different dimension?
-
Fun thing, I tried to do the exact same thing two years ago. Just generate a random new dimension with the same WorldGen and seed the overworld has and you should be (mostly) fine. Has been quite a struggle to find any good reference material back then. And I have no idea how much things have changed. But why would you even want to create a copy of the overworld? If you just want to reset everything to how it has been when the world was generated you could just delete the chunk and let MC regenerate it. Way faster and uses a lot less disk space.
-
Show us your code. And any log you might have. What exactly happens?
-
Resource pack sounds like a really bad idea. As soon as someone adds a new block it will no longer be affected by you resource pack. What I would instead try to do is try to implement a custom shader. (Like the super secret settings.) But I have never seen anyone do this in forge before. I would be very interested if you somehow manage to pull this of.
-
Not entirely true. It is possible to use damage to load different textures. Some adventure maps are doing that to create their custom items. (You will have to make your item unbreakable to keep it from mutating into different swords though) But that's more a resource pack question than a forge question.
-
Never heard of subworlds before. This sounds very, very interesting. Can you give me some examples of it or a documentation? @WOLFI3654 Use google. took me like 2 minutes. If you feel up to it you can use JDGUI and have a look at Archimedes Ships Plus. Not sure how good collision is working though.
-
You will have to turn your blocks into an entity (mob).
-
@Choonster Damn, I knew it. The ForgeRegistries class was the first place I looked. Could it be that I need a forge version newer than 14.21.1.2387(recommended) if I want to get the registries from inside the ForgeRegistries class?
-
Not sure if you can still get registered biomes from the BiomeDictionary, but older versions had a getBiomesForType(Type.Forest) that could be useful (I seriously doubt you really want it to be in absolutely every biome. e.g. ocean and nether). Another way I found is to wait for the RegistryEvent.Register<Biome> event and then just do something like this: final IForgeRegistry<Biome> registry = event.getRegistry(); for(Biome b :registry) { System.out.println(b.getBiomeName()); } But I am sure that there is a better way to get access to the ForgeRegistry. My example above is an absolute nightmare for way too many reasons. Could one of the code gods please descend and help us?
-
And what is while (world.isAirBlock(pos.down()) && pos.getY() > 55) { pos.subtract(new Vec3i(0, 1, 0)); } if (!world.isAirBlock(pos)) return false; supposed to do? I am guessing that you plan to go down from the highest block until you find on that is not air. The problem is that your pos y value is 0 at the start of the generate function. Replace it with something like: pos = new BlockPos(pos.getX(), world.getTopSolidOrLiquidBlock(pos).getY(), pos.getZ());
-
[1.12] [Solved] Inventory textures not loading
Jacky2611 replied to WARDOGSK93's topic in Modder Support
How the hell do you always know when someone mentions SideOnly @diesieben07? I haven't seen a single thread that talks about it without you appearing. -
Sry, had to finish something else first. I added an item that tells me the biomes position when I rightclick something with it and found this in just a few minutes. (changed the top_block of your biome to diamond to make it easier to see) The problem definitely are your trees. You can probably fix it if you check if the chunk has already been generated before you place any blocks in it. I tried to reupload the entire project (with a newer forge version, a fixed file structure and my (terrible) BiomeFinder) but it looks like github is down. I will do it as soon as possible.
-
Give me a moment, I am pulling it now... Damn, you are running a outdated forge version. I am currently updating everything to the recommended version for 1.8