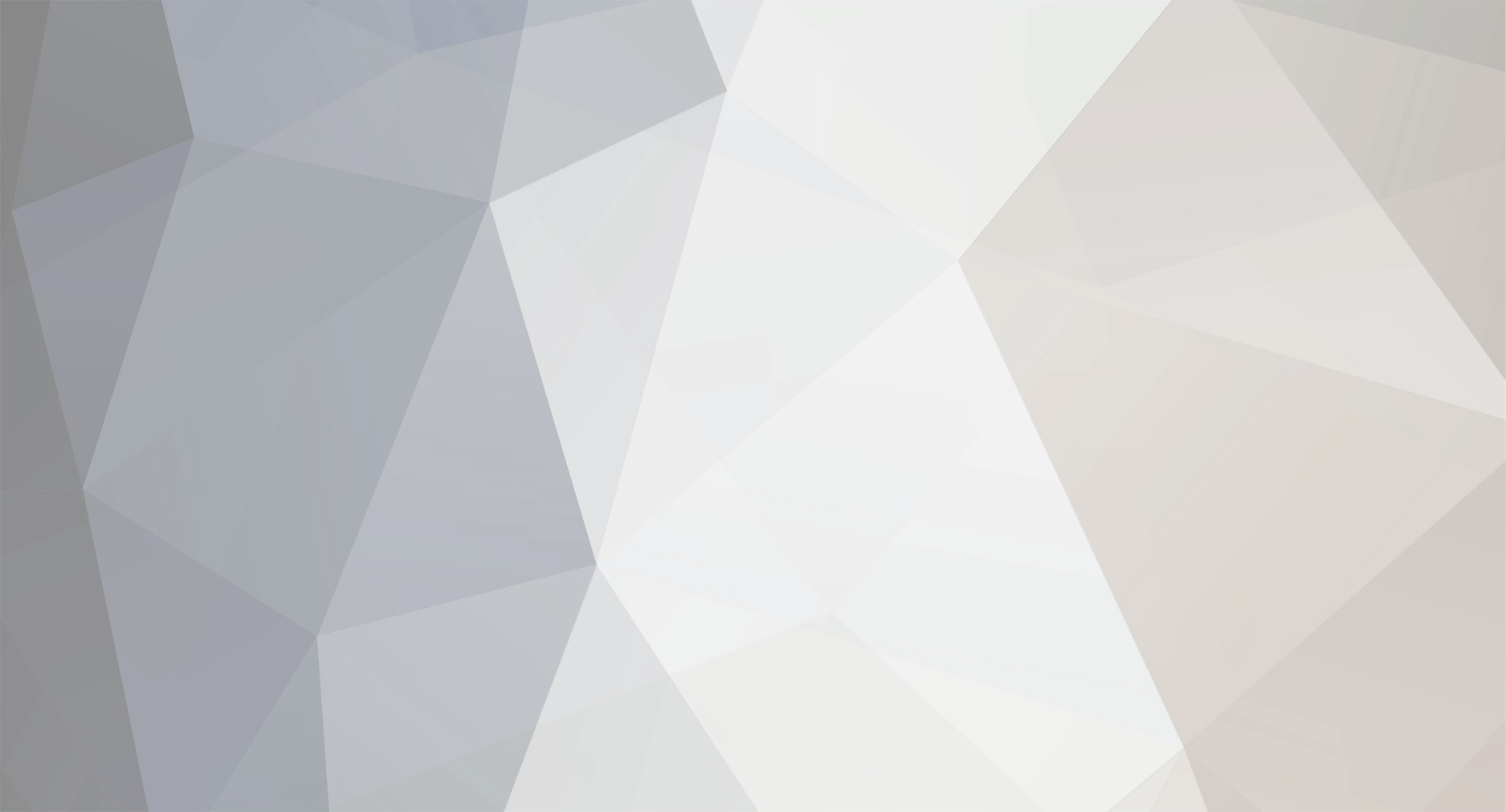
warjort
Members-
Posts
5420 -
Joined
-
Last visited
-
Days Won
175
Everything posted by warjort
-
These aren't forge/minecraft questions, these are java questions. We don't really answer those here. Knowing java is a prerequisite for minecraft modding. But since one of them is partly minecraft related I will answer them. Your main problem is you are not passing the correct parameter types or not passing parameters at all. (basic java). () -> new PlacedFeature(MY_KELP_CONFIGURED.getHolder().get())))); This constructor takes 2 parameters, you are missing the List of PlacementModifers which is the whole point of this class. You can see what is done for kelp in AquaticPlacements. event.getGeneration().addFeature(GenerationStep.Decoration.VEGETAL_DECORATION, MY_KELP_PLACED); This methods wants a PlacedFeature not a RegistryObject<PlacedFeature>, use MY_KELP_PLACED.get() - which gives you the real object.
-
The /give command is handled by you guessed it the GiveCommand class. ๐ You are still going to need the Block.use() method, except instead of dropping the item on the ground, you have to put in the player's inventory. Fortunately for you Forge has you covered. You don't need to copy the code from the GiveCommand, you can use ItemHandlerHelper.giveItemToPlayer(player, itemStack, 0);
-
I think you are new to java as well. ๐ * Your code only runs the client because calling super.use() returns PASS which means you don't want to do anything on the server. * Entity.spawnAtLocation is not a static method, your code won't even compile - you need an instance of an entity, e.g. player.spawnAtLocation() Look at CraftingTableBlock.use() - replace displaying the gui with your code Minecraft's interactions methods use an idiom where they are called twice, first on the client and then if you return SUCCESS it calls the same method on the server. Here's a link to the wiki: https://forge.gemwire.uk/wiki/Main_Page you should at least read about "Understanding sides"
-
You need a placed feature as well. Feature = the thing that places blocks ConfiguredFeature = Configuration(s) of that feature, e.g. how big an ore vein or which ore PlacedFeature = PlacementModifier(s) that decides where to put it, e.g. cactus goes on sand, diamonds generate at the bottom of the world But more importantly you need a Biome that uses it. To modify vanilla biomes see: https://forge.gemwire.uk/wiki/Biome_Modifiers or before 1.19 you use the BiomeLoadingEvent
-
ban a player who has reached the time limit played
warjort replied to 3mptysl's topic in Modder Support
look at the code in BanPlayerCommands.banPlayers() -
There are many different places in the minecraft code that does this (some more complicated than others). Perhaps the simplest is Containers.dropItemStack() which is used to drop items on the ground when you break a chest or furnace, etc. Another would be Entity.spawnAtLocation() which spawns an Item(Stack) at the entity's location. NOTE: You need do this on the logical server.
-
That's a feature correct? Continuing the theme of reinventing vanilla ๐ Something like (untested code): private static final DeferredRegister<Feature<?>> FEATURES = DeferredRegister.create(Registry.FEATURE_REGISTRY, ExampleMod.MODID); public static final RegistryObject<Feature<NoneFeatureConfiguration>> MY_KELP = FEATURES.register("my_kelp", () -> new KelpFeature(NoneFeatureConfiguration.CODEC)); public static final RegistryObject<ConfiguredFeature<?, ?>> MY_KELP_CONFIGURED = CONFIGURED_FEATURES.register("my_kelp", () -> new ConfiguredFeature<>(MY_KELP.get(), NoneFeatureConfiguration.INSTANCE));
-
Isn't that trivial? Maybe I don't understand your question. Why you would need your own configured feature for this (it is the same as the vanilla one) unless you intend to use your own/different Feature. public static final RegistryObject<ConfiguredFeature<?, ?>> MY_KELP_CONFIGURED = CONFIGURED_FEATURES.register("my_kelp", () -> new ConfiguredFeature<>(Feature.KELP, NoneFeatureConfiguration.INSTANCE));
-
[1.19.2] How to make your tree grow only on sand?
warjort replied to Pherment's topic in Modder Support
I am no expert on trees, but I know the basics of how this works. You don't show your PlacedFeature? PlacedFeatures have a list of PlacementModifiers. The vanilla trees use PlacementUtils.filteredBlockBySurvival() where the Block parameter is the sapling. This will call your sapling block's canSurvive(), see BushBlock.canSurvive() for the default implementation. Also, it is recommended to use DeferredRegister rather than the vanilla registration methods. https://forums.minecraftforge.net/topic/115928-1182-error-trying-to-register-a-custom-feature-based-on-kelpfeature/#comment-512366 -
Here's some similar bug reports on github, maybe one is relevant to you? https://github.com/search?q=Cannot+invoke+"net.minecraft.resources.ResourceLocation.toString()"+because+"p_130086_"+is+null&type=issues
-
The most likely ones will be the mods you have with fewest downloads. If this problem existed in any popular mods it would have been reported and fixed already. ๐
-
So we found the error message, but unfortunately it is one of those that doesn't identify the mod. All it says is that it can't send the recipes to the client because there is that null ResourceLocation [23Aug2022 21:52:30.530] [Netty Server IO #1/DEBUG] [net.minecraft.network.Connection/]: Failed to sent packet io.netty.handler.codec.EncoderException: java.lang.NullPointerException: Cannot invoke "net.minecraft.resources.ResourceLocation.toString()" because "p_130086_" is null at io.netty.handler.codec.MessageToMessageEncoder.write(MessageToMessageEncoder.java:104) ~[netty-codec-4.1.77.Final.jar%2380!/:4.1.77.Final] at io.netty.channel.AbstractChannelHandlerContext.invokeWrite0(AbstractChannelHandlerContext.java:717) ~[netty-transport-4.1.77.Final.jar%2384!/:4.1.77.Final] at io.netty.channel.AbstractChannelHandlerContext.invokeWriteAndFlush(AbstractChannelHandlerContext.java:764) ~[netty-transport-4.1.77.Final.jar%2384!/:4.1.77.Final] at io.netty.channel.AbstractChannelHandlerContext.write(AbstractChannelHandlerContext.java:790) ~[netty-transport-4.1.77.Final.jar%2384!/:4.1.77.Final] at io.netty.channel.AbstractChannelHandlerContext.writeAndFlush(AbstractChannelHandlerContext.java:758) ~[netty-transport-4.1.77.Final.jar%2384!/:4.1.77.Final] at io.netty.channel.AbstractChannelHandlerContext.writeAndFlush(AbstractChannelHandlerContext.java:808) ~[netty-transport-4.1.77.Final.jar%2384!/:4.1.77.Final] at io.netty.channel.DefaultChannelPipeline.writeAndFlush(DefaultChannelPipeline.java:1025) ~[netty-transport-4.1.77.Final.jar%2384!/:4.1.77.Final] at io.netty.channel.AbstractChannel.writeAndFlush(AbstractChannel.java:306) ~[netty-transport-4.1.77.Final.jar%2384!/:4.1.77.Final] at net.minecraft.network.Connection.m_243087_(Connection.java:209) ~[server-1.19.2-20220805.130853-srg.jar%23348!/:?] at net.minecraft.network.Connection.lambda$sendPacket$8(Connection.java:198) ~[server-1.19.2-20220805.130853-srg.jar%23348!/:?] at io.netty.util.concurrent.AbstractEventExecutor.runTask(AbstractEventExecutor.java:174) [netty-common-4.1.77.Final.jar%2381!/:4.1.77.Final] at io.netty.util.concurrent.AbstractEventExecutor.safeExecute(AbstractEventExecutor.java:167) [netty-common-4.1.77.Final.jar%2381!/:4.1.77.Final] at io.netty.util.concurrent.SingleThreadEventExecutor.runAllTasks(SingleThreadEventExecutor.java:470) [netty-common-4.1.77.Final.jar%2381!/:4.1.77.Final] at io.netty.channel.nio.NioEventLoop.run(NioEventLoop.java:503) [netty-transport-4.1.77.Final.jar%2384!/:4.1.77.Final] at io.netty.util.concurrent.SingleThreadEventExecutor$4.run(SingleThreadEventExecutor.java:995) [netty-common-4.1.77.Final.jar%2381!/:4.1.77.Final] at io.netty.util.internal.ThreadExecutorMap$2.run(ThreadExecutorMap.java:74) [netty-common-4.1.77.Final.jar%2381!/:4.1.77.Final] at java.lang.Thread.run(Thread.java:833) [?:?] Caused by: java.lang.NullPointerException: Cannot invoke "net.minecraft.resources.ResourceLocation.toString()" because "p_130086_" is null at net.minecraft.network.FriendlyByteBuf.m_130085_(FriendlyByteBuf.java:610) ~[server-1.19.2-20220805.130853-srg.jar%23348!/:?] at net.minecraft.network.protocol.game.ClientboundUpdateRecipesPacket.m_179469_(ClientboundUpdateRecipesPacket.java:50) ~[server-1.19.2-20220805.130853-srg.jar%23348!/:?] at net.minecraft.network.FriendlyByteBuf.m_236828_(FriendlyByteBuf.java:162) ~[server-1.19.2-20220805.130853-srg.jar%23348!/:?] at net.minecraft.network.protocol.game.ClientboundUpdateRecipesPacket.m_5779_(ClientboundUpdateRecipesPacket.java:27) ~[server-1.19.2-20220805.130853-srg.jar%23348!/:?] at net.minecraftforge.network.filters.VanillaPacketSplitter.appendPackets(VanillaPacketSplitter.java:68) ~[forge-1.19.2-43.1.2-universal.jar%23353!/:?] at net.minecraftforge.network.filters.ForgeConnectionNetworkFilter.splitPacket(ForgeConnectionNetworkFilter.java:60) ~[forge-1.19.2-43.1.2-universal.jar%23353!/:?] at net.minecraftforge.network.filters.VanillaPacketFilter.encode(VanillaPacketFilter.java:60) ~[forge-1.19.2-43.1.2-universal.jar%23353!/:?] at net.minecraftforge.network.filters.VanillaPacketFilter.encode(VanillaPacketFilter.java:23) ~[forge-1.19.2-43.1.2-universal.jar%23353!/:?] at io.netty.handler.codec.MessageToMessageEncoder.write(MessageToMessageEncoder.java:89) ~[netty-codec-4.1.77.Final.jar%2380!/:4.1.77.Final] ... 16 more The only way to find the problem mod will be remove mods until the problem stops happening.
-
Yes it looks to be related to the new chat system: https://feedback.minecraft.net/hc/en-us/articles/7525860212749-Minecraft-Java-Edition-1-19-1-Pre-Release-4
-
I don't know what that "[Not Secure]" is. Maybe it is related to new chat system in 1.19.2? Did you type "test" in the system console or something?
-
That file does show any error from what I can see. You can turn it back to "info" now. ๐ Can you try doing what I said above
-
That is an error on the server for a different mod. If the client log does not contain the error, the next step is to look at the server's debug.log with the -Dforge.logging.mojang.level=debug in your user_jvm_args.txt
-
Can you try changing this line <Root level="info"> to <Root level="debug">
-
It looks like their log4j configuration is here? -Dlog4j.configurationFile=C:\Users\david\AppData\Roaming\gdlauncher_next\datastore\assets\objects\bd\client-1.12.xml
-
You need to ask gdlauncher how to get the forge debug.log Or how to change their log file you posted before to show debug information.
-
That is not the logs/debug.log Your actual error message looks like something on the client is to trying to write null data into a network packet, but doesn't give anymore information than that. This leads to you getting disconnected. This is probably one of those places where Mojang doesn't put the error message in the log file properly. If you add the following to the jvm arguments of your client it might display this error, but then only if you have the proper debug.log -Dforge.logging.mojang.level=debug
-
The Game crashed whilst rendering overlay
warjort replied to ChrisNT's topic in Support & Bug Reports
[23ago.2022 11:42:27.250] [Render thread/ERROR] [net.minecraftforge.fml.javafmlmod.FMLModContainer/]: Exception caught during firing event: net/minecraftforge/client/IItemRenderProperties Index: 1 Listeners: 0: NORMAL 1: ASM: class com.legacy.mining_helmet.MiningHelmetRegistry onRegister(Lnet/minecraftforge/registries/RegisterEvent;)V java.lang.NoClassDefFoundError: net/minecraftforge/client/IItemRenderProperties This is an error in the mining helmet mod you have. I don't even see the version you have on curseforge? https://www.curseforge.com/minecraft/mc-mods/miners-helmet/files/all?filter-game-version=1738749986%3a73407 -
The Game crashed whilst rendering overlay
warjort replied to ChrisNT's topic in Support & Bug Reports
You need to post a link to your logs/debug.log so we can see what the real error is. -
The Game crashed whilst rendering overlay
warjort replied to ChrisNT's topic in Support & Bug Reports
The latest version of creative core says it supports 1.19, 1.19.1 and 1.19.2 It is also a library mod so the problem could be one of the mods that uses it. https://www.curseforge.com/minecraft/mc-mods/creativecore/relations/dependents?filter-related-dependents=3 If you can't get it to work, contact the mod author(s) -
The Game crashed whilst rendering overlay
warjort replied to ChrisNT's topic in Support & Bug Reports
This is the latest version for 1.19: https://www.curseforge.com/minecraft/mc-mods/jei/files/3903068 jei-1.19-forge-11.1.1.239.jar you have jei-1.19-forge-11.0.0.206.jar -
You have a "mods" folder correct? You will also have "logs" folder. In there is the debug.log If it is not there, you need to ask aternos.