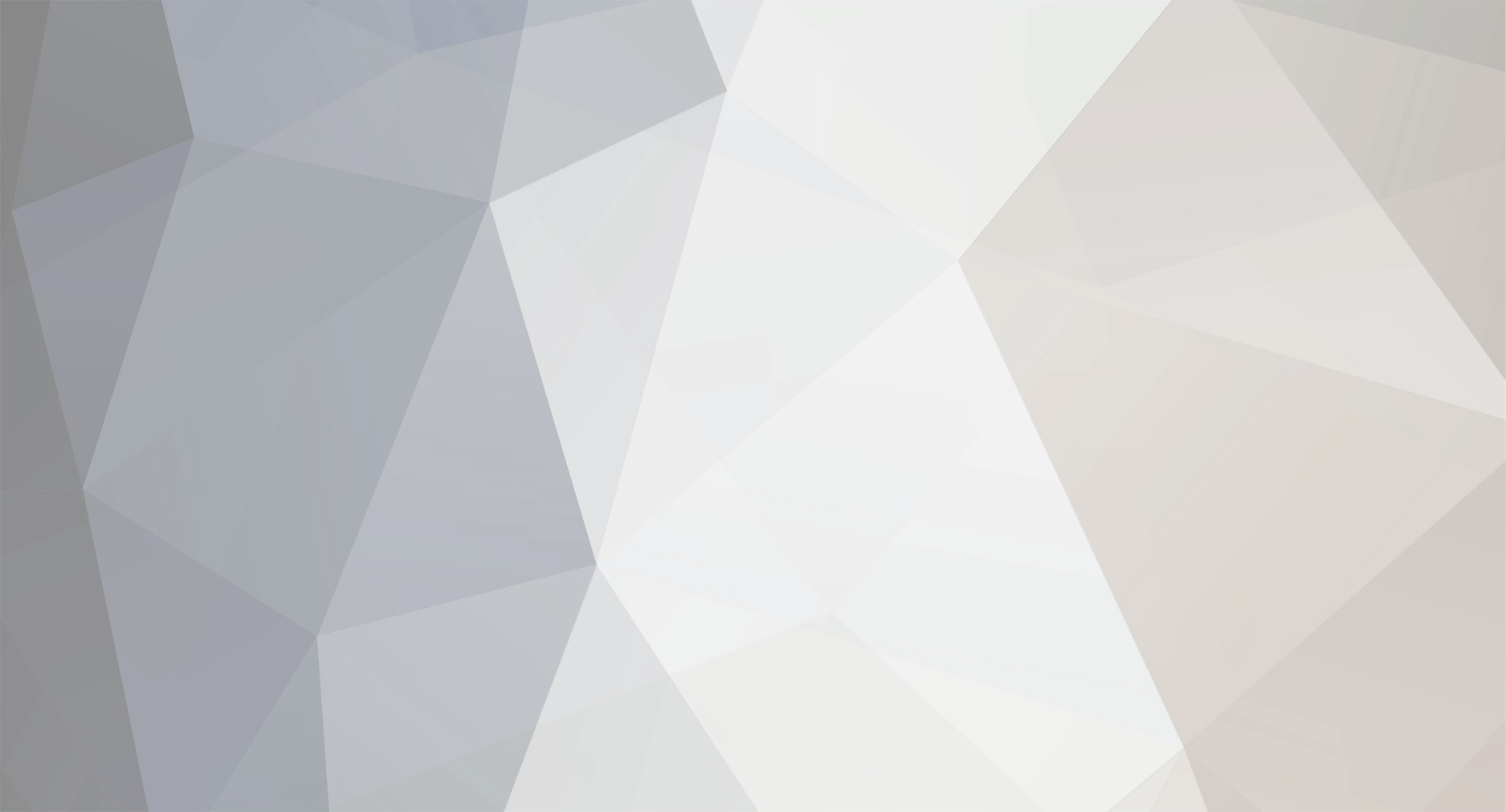
larsgerrits
Members-
Posts
3462 -
Joined
-
Last visited
-
Days Won
17
Everything posted by larsgerrits
-
Just use: t#(YOUR_VARIABLE_NAME/YOUR_METHOD_NAME
-
Try installing the scala eclipse plugin.
-
Gah! x..X Don't use static variables like that. While it's not messing up when executed, its generally not good practice. Those are prime examples of values that should be passed to the various functions that use them. Ok, I'll change that after it renders. I have a picture of the bounding boxes that work, and use the same class. Maybe it has something to with that the boundingbox method passes the IBlockAccess variable to the ConnectingIds class, but the TileEntitySpecialRenderer class a worldObj. [spoiler=Image]
-
Serieusly, i can't find it. Here's some more code: [spoiler=ConnectingIds] package larsg310.mod.techcraft.lib; import larsg310.mod.techcraft.block.TCBlocks; import net.minecraft.world.IBlockAccess; import net.minecraft.world.World; public class ConnectingIds { private static IBlockAccess world; private static int x; private static int y; private static int z; public static boolean getCableConnectings(IBlockAccess world1, int x1, int y1, int z1, String direction) { world = world1; x = x1; y = y1; z = z1; return isConnecting(direction); } public static boolean getCableConnectings(World world1, int x1, int y1, int z1, String direction) { world = world1; x = x1; y = y1; z = z1; return isConnecting(direction); } public static int getNorthBlock() { return world.getBlockId(x-1, y, z); } public static int getSouthBlock() { return world.getBlockId(x+1, y, z); } public static int getEastBlock() { return world.getBlockId(x, y, z-1); } public static int getWestBlock() { return world.getBlockId(x, y, z+1); } public static int getUpBlock() { return world.getBlockId(x, y-1, z); } public static int getDownBlock() { return world.getBlockId(x, y+1, z); } public static boolean isConnecting(String direction) { boolean north = false; boolean east = false; boolean south = false; boolean west = false; boolean up = false; boolean down = false; if(direction == "north") { if(getNorthBlock() == TCBlocks.redstonePowerCable.blockID) north = true; else if(getNorthBlock() == TCBlocks.redstonePowerGenerator.blockID) north = true; return north; } if(direction == "south") { if(getSouthBlock() == TCBlocks.redstonePowerCable.blockID) south = true; else if(getSouthBlock() == TCBlocks.redstonePowerGenerator.blockID) south = true; return south; } if(direction == "east") { if(getEastBlock() == TCBlocks.redstonePowerCable.blockID) east = true; else if(getEastBlock() == TCBlocks.redstonePowerGenerator.blockID) east = true; return east; } if(direction == "west") { if(getWestBlock() == TCBlocks.redstonePowerCable.blockID) west = true; else if(getWestBlock() == TCBlocks.redstonePowerGenerator.blockID) west = true; return west; } if(direction == "up") { if(getUpBlock() == TCBlocks.redstonePowerCable.blockID) up = true; else if(getUpBlock() == TCBlocks.redstonePowerGenerator.blockID) up = true; return up; } if(direction == "down") { if(getDownBlock() == TCBlocks.redstonePowerCable.blockID) down = true; else if(getDownBlock() == TCBlocks.redstonePowerGenerator.blockID) down = true; return down; } return false; } }
-
Do you have scala installed?
-
Hello, I made a cable-like block with a custom model etc. and the middle part renders always as i want to, but it doesn't render other parts based on the cables next to it. [spoiler=TileEntitySpecialRenderer] package larsg310.mod.techcraft.renderer.tileentity; import larsg310.mod.techcraft.lib.ConnectingIds; import larsg310.mod.techcraft.lib.Reference; import larsg310.mod.techcraft.model.ModelRedstonePowerCable; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.ResourceLocation; import net.minecraft.world.World; import org.lwjgl.opengl.GL11; public class TileEntityRedstonePowerCableRenderer extends TileEntitySpecialRenderer { private ModelRedstonePowerCable model; public ResourceLocation REDSTONE_POWER_CABLE_TEXTURE = new ResourceLocation(Reference.MOD_ID, "textures/model/ModelRedstonePowerCable.png"); public TileEntityRedstonePowerCableRenderer() { this.model = new ModelRedstonePowerCable(); } public void renderTileEntityAt(TileEntity tileentity, double x, double y, double z, float f) { World world = tileentity.worldObj; GL11.glPushMatrix(); GL11.glTranslatef((float)x + 0.5F, (float)y + 1.5F, (float)z + 0.5F); GL11.glRotatef(180, 0, 0, 1); this.bindTexture(REDSTONE_POWER_CABLE_TEXTURE); GL11.glPushMatrix(); model.renderAll(world, (int)x, (int)y, (int)z); GL11.glPopMatrix(); GL11.glPopMatrix(); } } [spoiler=Model Class] package larsg310.mod.techcraft.model; import larsg310.mod.techcraft.lib.ConnectingIds; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; import net.minecraft.world.World; public class ModelRedstonePowerCable extends ModelBase { ModelRenderer Middle; ModelRenderer ExtensionNorth; ModelRenderer ExtensionEast; ModelRenderer ExtensionSouth; ModelRenderer ExtensionWest; ModelRenderer ExtensionDown; ModelRenderer ExtensionUp; public ModelRedstonePowerCable() { textureWidth = 64; textureHeight = 64; Middle = new ModelRenderer(this, 0, 0); Middle.addBox(0F, 0F, 0F, 4, 4, 4); Middle.setRotationPoint(-2F, 14F, -2F); Middle.setTextureSize(64, 64); Middle.mirror = true; setRotation(Middle, 0F, 0F, 0F); ExtensionNorth = new ModelRenderer(this, 0, 9); ExtensionNorth.addBox(0F, 0F, 0F, 4, 4, 6); ExtensionNorth.setRotationPoint(-2F, 14F, 2F); ExtensionNorth.setTextureSize(64, 64); ExtensionNorth.mirror = true; setRotation(ExtensionNorth, 0F, 0F, 0F); ExtensionEast = new ModelRenderer(this, 0, 20); ExtensionEast.addBox(0F, 0F, 0F, 6, 4, 4); ExtensionEast.setRotationPoint(2F, 14F, -2F); ExtensionEast.setTextureSize(64, 64); ExtensionEast.mirror = true; setRotation(ExtensionEast, 0F, 0F, 0F); ExtensionSouth = new ModelRenderer(this, 0, 9); ExtensionSouth.addBox(0F, 0F, 0F, 4, 4, 6); ExtensionSouth.setRotationPoint(-2F, 14F, -8F); ExtensionSouth.setTextureSize(64, 64); ExtensionSouth.mirror = true; setRotation(ExtensionSouth, 0F, 0F, 0F); ExtensionWest = new ModelRenderer(this, 0, 20); ExtensionWest.addBox(0F, 0F, 0F, 6, 4, 4); ExtensionWest.setRotationPoint(-8F, 14F, -2F); ExtensionWest.setTextureSize(64, 64); ExtensionWest.mirror = true; setRotation(ExtensionWest, 0F, 0F, 0F); ExtensionDown = new ModelRenderer(this, 0, 29); ExtensionDown.addBox(0F, 0F, 0F, 4, 6, 4); ExtensionDown.setRotationPoint(-2F, 18F, -2F); ExtensionDown.setTextureSize(64, 64); ExtensionDown.mirror = true; setRotation(ExtensionDown, 0F, 0F, 0F); ExtensionUp = new ModelRenderer(this, 0, 29); ExtensionUp.addBox(0F, 0F, 0F, 4, 6, 4); ExtensionUp.setRotationPoint(-2F, 8F, -2F); ExtensionUp.setTextureSize(64, 64); ExtensionUp.mirror = true; setRotation(ExtensionUp, 0F, 0F, 0F); } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { super.render(entity, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, entity); Middle.render(f5); ExtensionNorth.render(f5); ExtensionEast.render(f5); ExtensionSouth.render(f5); ExtensionWest.render(f5); ExtensionDown.render(f5); ExtensionUp.render(f5); } public void renderAll(World world, int x, int y, int z) { Middle.render(0.0625F); if(ConnectingIds.getCableConnectings(world, x, y, z, "north")) ExtensionNorth.render(0.0625F); if(ConnectingIds.getCableConnectings(world, x, y, z, "east")) ExtensionEast.render(0.0625F); if(ConnectingIds.getCableConnectings(world, x, y, z, "south")) ExtensionSouth.render(0.0625F); if(ConnectingIds.getCableConnectings(world, x, y, z, "west")) ExtensionWest.render(0.0625F); if(ConnectingIds.getCableConnectings(world, x, y, z, "up")) ExtensionDown.render(0.0625F); if(ConnectingIds.getCableConnectings(world, x, y, z, "down")) ExtensionUp.render(0.0625F); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5, Entity entity) { super.setRotationAngles(f, f1, f2, f3, f4, f5, entity); } } I'm not putting in the ConnectingIds class, because i know it works because i'm using it to make the block bounds in the block class. If you need mor classes i'll put them here.
-
Try changing the side == 3 in your getIcon method to side == meta that should fix the rotating. For the second one, the vanilla furnace uses 2 blocks, one with active and one with the idle texture. Try that way.
-
That looks even impressiver than without smooth lightning.
-
[SOLVED] Item Overriding? Very confused - Forge 1.6.2
larsgerrits replied to squealy_squigs's topic in Modder Support
You aren't setting a value to IDs.adminiumPickaxe_actual. -
If the project is finished, can you upload some screenshots and maybe make API of it, so other mods can use it too. BTW, your project looks amazing!
-
Hey, while i was updating my workspace to 1.6.4, i get a crash. Here it is: [spoiler=Error]okt 03, 2013 7:43:53 PM net.minecraft.launchwrapper.LogWrapper log INFO: Loading tweak class name cpw.mods.fml.common.launcher.FMLTweaker okt 03, 2013 7:43:53 PM net.minecraft.launchwrapper.LogWrapper log INFO: Using primary tweak class name cpw.mods.fml.common.launcher.FMLTweaker okt 03, 2013 7:43:53 PM net.minecraft.launchwrapper.LogWrapper log INFO: Calling tweak class cpw.mods.fml.common.launcher.FMLTweaker 2013-10-03 19:43:54 [iNFO] [ForgeModLoader] Forge Mod Loader version 6.4.20.916 for Minecraft 1.6.4 loading 2013-10-03 19:43:54 [iNFO] [ForgeModLoader] Java is Java HotSpot Client VM, version 1.7.0_25, running on Windows 8:x86:6.2, installed at C:\Program Files (x86)\Java\jre7 2013-10-03 19:43:54 [iNFO] [ForgeModLoader] Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation 2013-10-03 19:43:55 [iNFO] [sTDOUT] Loaded 40 rules from AccessTransformer config file fml_at.cfg 2013-10-03 19:43:55 [iNFO] [sTDOUT] Loaded 109 rules from AccessTransformer config file forge_at.cfg 2013-10-03 19:43:55 [sEVERE] [ForgeModLoader] The binary patch set is missing. Either you are in a development environment, or things are not going to work! 2013-10-03 19:43:57 [iNFO] [ForgeModLoader] Loading tweak class name cpw.mods.fml.common.launcher.FMLDeobfTweaker 2013-10-03 19:43:57 [iNFO] [ForgeModLoader] Calling tweak class cpw.mods.fml.common.launcher.FMLDeobfTweaker 2013-10-03 19:43:58 [iNFO] [ForgeModLoader] Launching wrapped minecraft {net.minecraft.client.main.Main} 2013-10-03 19:43:59 [sEVERE] [ForgeModLoader] Unable to launch java.lang.reflect.InvocationTargetException at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at net.minecraft.launchwrapper.Launch.launch(Launch.java:131) at net.minecraft.launchwrapper.Launch.main(Launch.java:27) Caused by: java.lang.UnsatisfiedLinkError: no lwjgl in java.library.path at java.lang.ClassLoader.loadLibrary(Unknown Source) at java.lang.Runtime.loadLibrary0(Unknown Source) at java.lang.System.loadLibrary(Unknown Source) at org.lwjgl.Sys$1.run(Sys.java:73) at java.security.AccessController.doPrivileged(Native Method) at org.lwjgl.Sys.doLoadLibrary(Sys.java:66) at org.lwjgl.Sys.loadLibrary(Sys.java:95) at org.lwjgl.Sys.<clinit>(Sys.java:112) at net.minecraft.client.Minecraft.getSystemTime(Minecraft.java:2457) at net.minecraft.client.main.Main.main(Main.java:37) ... 6 more It seems like to me that it couldn't find lwjgl in java, but in 1.6.2 it worked? Anyone know how this can be solved? [spoiler=Imported Libraries]
-
BTW use reobfuscate_srg
-
In the class where you register your blocks, add MinecraftForge.setBlockHarvestLevel(par1, "par2", par3 And replace par1 with your block, replace par2 with pickaxe, axe or shovel, and replace par3 with the harvest level, look in EnmuToolMaterial to find the harvest level for the tools
-
Try using FMLPreInitializationEvent
-
Forge installer just gives a new version in the profile options - I want to create the actual profile, the thing you can select from a dropdown list on the launcher itself (not inside the options) Then i don't know how to do it...
-
You mean like the Forge installer does? Try and find(maybe ask) the source code for it.
-
Yeah that's it. If you don't have that, add that and test if it works. If it doesn't maybe you could put everything after the first if statement in a else statement.
-
Thanks for calling me mr genius, but now back to the topic Did you register the class with the LivingHurtEntity event like so: MinecraftForge.EVENT_BUS.register(new YOUR_CLASS_WITH_LIVING_HURT_ENTITY_EVENT
-
It crashes on this line: EntityDamageSource dmgSource = (EntityDamageSource) event.source; Maybe the source isn't a EntitiDamageSource? (never worked with damage etc.)
-
I think it means, that something in your code, probably a severe thingy, closed minecraft. Correct me if i'm wrong.
-
Minecraft crashing in eclipse after making config file
larsgerrits replied to RosarioMokaChan's topic in Modder Support
Code? -
What i think is happening, is that your tile entity is desynchronized between client and server.