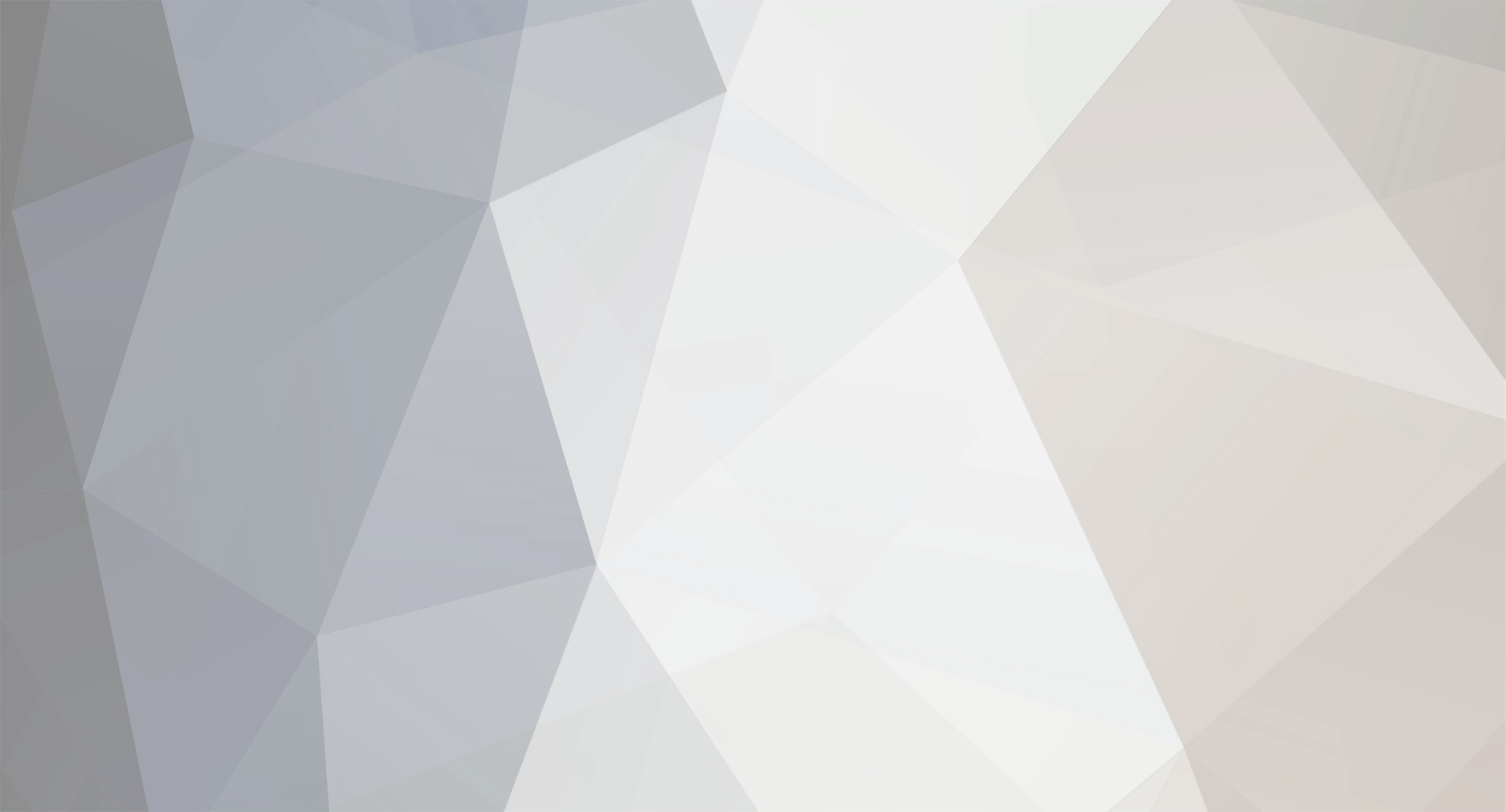
Alexiy
Forge Modder-
Posts
198 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Alexiy
-
What is there to dissect? The source code is available. If you mean to edit the minecraft code directly, then you'll have to set up a custom environment - the code is located in gradle's cache directory as a Jar.
-
I also tried using JavaFx, but it seems that it is not being loaded / is excluded from the libraries shipped with the game. You will have to design you gui elements yourself.
-
You know many mods use this event to draw custom things on screen - strings, images/textures, OGL objects... It can result in HUD having these things overlapped, because I don't see a way to detect position of drawn elements from the event. Would it be possible to make a system for providing info at what screen coordinates the element is drawn, so other mods can query them and adjust their elements accordingly to avoid overlapping? Like, make an interface with getXposition, getYposition, getWidth, getHeight, so a modder can implement it and put the implementation into a list which would be in the RenderGameOverLayEvent. This way we can query this list, get positions and size of all added elements and add our element considering their coordinates.
-
I can see a method 'isValidArmor' in the Item.class. I haven't used it, but it seems the right one, so here is example: @Override public boolean isValidArmor(ItemStack stack, EntityEquipmentSlot armorType, Entity entity) { return armorType!=EntityEquipmentSlot.OFFHAND; }
-
Yes, you will need to put Baubles into the 'mods' folder, just like in regular environment. To add a library from repository, you will need to specify the repository url and a dependency. Example for Just Enough Items: repositories{ //the repository that contains the libraries maven{ url "http://dvs1.progwml6.com/files/maven" } } dependencies{ //the dependency compile 'mezz.jei:jei_1.10.2:3.14.5.406:api' }
-
It depends how the API is being provided. If it's plain source, like Baubles or RF API, then you just download their source, extract it and put it into the 'api' module of the project. If it's provided as a library from a repository, like JEI or Refined Storage, then you add appropriate entries to your build.gradle file.
-
If your tile entity is processing stuff on the server world, you only need to send packet to those clients that are able to see the progress. You can make a boolean in tile entity that will indicate whether it should send the progress. Then in the container class, you pass the tile entity to its constructor and set this boolean flag to true. Then in tile's update() method, you check this flag and send a packet containing the progress value to the players that can see the progress. When container is closed, you set the flag to false, so there are no unneeded packets sent.
-
[1.10.2] How do I get started with Forge Energy?
Alexiy replied to Willbl3pic's topic in Modder Support
What Shadows said, and for start you might want to extend default EnergyStorage to implement energy extractors and energy providers. Then store these objects in your tile entities and provide them in mentioned methods. -
[1.10.2] Gui doesn't update progress or RF storage[RESOLVED]
Alexiy replied to RealTheUnderTaker11's topic in Modder Support
Did you know that there is an energy capability? net.minecraftforge.energy.CapabilityEnergy - I suggest using that. Also you can read about capabilities here http://mcforge.readthedocs.io/en/latest/datastorage/capabilities/, although it's not explanatory enough. If you have any questions about them, do ask, because I recently refactored my mod to use item and energy capabilities and dropped IInventory interfaces. -
Method for finding client instance of server entity?
Alexiy replied to Stephen_789's topic in Modder Support
Use net.minecraft.entity.Entity#getEntityId. It should be same. If it doesn't help, show the synchronisation code. -
Example: I have a block implementation with connections. So, for each side there is a boolean property which indicates whether there is a same block at that side. If true, they are rendered with a 'pipe' between them. This check is performed in getActualState and the blockstate file applies the appropriate model for rendering.
-
Isn't it 'reobfJar' ?
-
You also need to override following methods to make synchronisation automatic between server and client: @Override public SPacketUpdateTileEntity getUpdatePacket() { BlockPos blockPos=getPos(); return new SPacketUpdateTileEntity(blockPos,0,getUpdateTag()); } @Override public void onDataPacket(NetworkManager net, SPacketUpdateTileEntity pkt) { NBTTagCompound nbtTagCompound=pkt.getNbtCompound(); super.readFromNBT(nbtTagCompound); readFromNBT(nbtTagCompound); } @Override public NBTTagCompound getUpdateTag() { NBTTagCompound supertag=super.getUpdateTag(); writeToNBT(supertag); return supertag; } @Override public void handleUpdateTag(NBTTagCompound tag) { super.handleUpdateTag(tag); readFromNBT(tag); }
-
[1.10.2]GuiContainer: draw texture in foreground of an item in slot
Alexiy replied to Mark136's topic in Modder Support
Yes, look at the GuiContainerCreative#handleMouseInput method, this is where scrolling is handled. -
[1.10] custom thin block is causing 'x-ray' effects.
Alexiy replied to needoriginalname's topic in Modder Support
Try overriding public boolean isFullCube(IBlockState state) { return false; } -
Thanks, plus to you.
-
Is it in the form of source jar? If so, extract it and put result into 'api' source set.
-
All right, I refactored it, now it looks like this: public class TileStrongChest extends GeneralTileEntity { final static public String CHESTTYPE = "Chest type", ITEMSTACKS = "Item stacks"; public int type; public ItemStackHandler itemStackHandler; public TileStrongChest() {super();} public TileStrongChest(int metadata) { super(); type = metadata; itemStackHandler=new ItemStackHandler(metadata==0 ? 54 : metadata==1 ? 81 : 108); } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { if (capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) return true; return super.hasCapability(capability, facing); } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if (capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) return (T) itemStackHandler; return super.getCapability(capability, facing); } @Nullable @Override public ITextComponent getDisplayName() { int stacks=0; for (int i = 0; i < itemStackHandler.getSlots(); i++) { if(itemStackHandler.getStackInSlot(i)!=null)stacks++; } return new TextComponentString(stacks+"/"+ itemStackHandler.getSlots()); } @Override public NBTTagCompound writeToNBT(NBTTagCompound compound) { NBTTagCompound nbtTagCompound=super.writeToNBT(compound); NBTTagCompound handlernbt= itemStackHandler.serializeNBT(); nbtTagCompound.setTag(ITEMSTACKS,handlernbt); nbtTagCompound.setShort(CHESTTYPE, (short) type); return nbtTagCompound; } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); type=compound.getShort(CHESTTYPE); itemStackHandler=new ItemStackHandler(); itemStackHandler.deserializeNBT(compound.getCompoundTag(ITEMSTACKS)); } } Seems to be working. Although I have read the docs few times, I didn't understand it right away. I assert that it needs to show an example, or a link to one.
-
Here is my first attempt at using Capability and IItemHandler. The tile seems to work fine, but I would like someone's review to verify that I did this right or if something can be improved. public class TileStrongChest extends GeneralTileEntity implements IItemHandlerModifiable { final static public String CHESTTYPE="Chest type",ITEMSTACK="Item stack"; public int type; ItemStack[] storage; static public Capability<IItemHandler> iItemHandler=CapabilityItemHandler.ITEM_HANDLER_CAPABILITY; public ItemStackHandler itemStackHandler; public TileStrongChest(){super();} public TileStrongChest(int metadata) { super(); type=metadata; storage=new ItemStack[metadata==0 ? 54 : metadata==1 ? 81 : 108]; itemStackHandler=new ItemStackHandler(storage); } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { if(capability==iItemHandler) return true; return super.hasCapability(capability, facing); } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if(capability==iItemHandler) return (T) this; return super.getCapability(capability, facing); } @Nullable @Override public ITextComponent getDisplayName() { int stacks=0; for (int i = 0; i < getSlots(); i++) { if(storage[i]!=null)stacks++; } return new TextComponentString(stacks+"/"+ getSlots()); } @Override public int getSlots() { return storage.length; } @Override public ItemStack getStackInSlot(int slot) { return storage[slot]; } @Override public ItemStack insertItem(int slot, ItemStack stack, boolean simulate) { return itemStackHandler.insertItem(slot,stack,simulate); } @Override public ItemStack extractItem(int slot, int amount, boolean simulate) { return itemStackHandler.extractItem(slot,amount,simulate); } @Override public void setStackInSlot(int slot, ItemStack stack) { storage[slot]=stack; } @Override public NBTTagCompound writeToNBT(NBTTagCompound compound) { NBTTagCompound nbtTagCompound=super.writeToNBT(compound); int number=0; for(ItemStack itemStack: storage) { if(itemStack!=null) { NBTTagCompound item=itemStack.writeToNBT(new NBTTagCompound()); compound.setTag(ITEMSTACK+number,item); } number++; } nbtTagCompound.setShort(CHESTTYPE, (short) type); return nbtTagCompound; } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); type=compound.getShort(CHESTTYPE); switch (type) { case 0: storage=new ItemStack[54];break; case 1: storage=new ItemStack[81];break; case 2: storage=new ItemStack[108];break; } for (int i = 0; i < storage.length; i++) { ItemStack itemStack=ItemStack.loadItemStackFromNBT(compound.getCompoundTag(ITEMSTACK+i)); if(itemStack!=null)storage[i]=itemStack; } itemStackHandler=new ItemStackHandler(storage); } }
-
The buttons are stored in field 'buttonList' in class GuiScreen. It has protected access, so you will have to make a subclass of GuiScreen. From the subclass you will be able to delete/add buttons. Look into the source.
-
[1.7.10] How to update item when right clicking a block?
Alexiy replied to DaBananaboat's topic in Modder Support
You don't need "if(!world.isRemote)" piece. -
Press that "Download ZIP" button on the right and copy everything from "src/main" from archive.
-
[1.7.10]Crashing when opening custom Container Gui
Alexiy replied to _gjkf_'s topic in Modder Support
The x,y,z are the coordinates you need to pass: player.openGui(Main.instance, References.GUI_CORE_ID, world, x,y,z); They are the coordinates of block you are clicking. -
No, it's just a tip for modders.
-
There are some mods that have tools to perform operations such as rotating, transforming blocks. The outcome is shortage of inventory space. What would be a good solution is to use empty hand as a tool. Combined with sneak+right click you get another "tool".