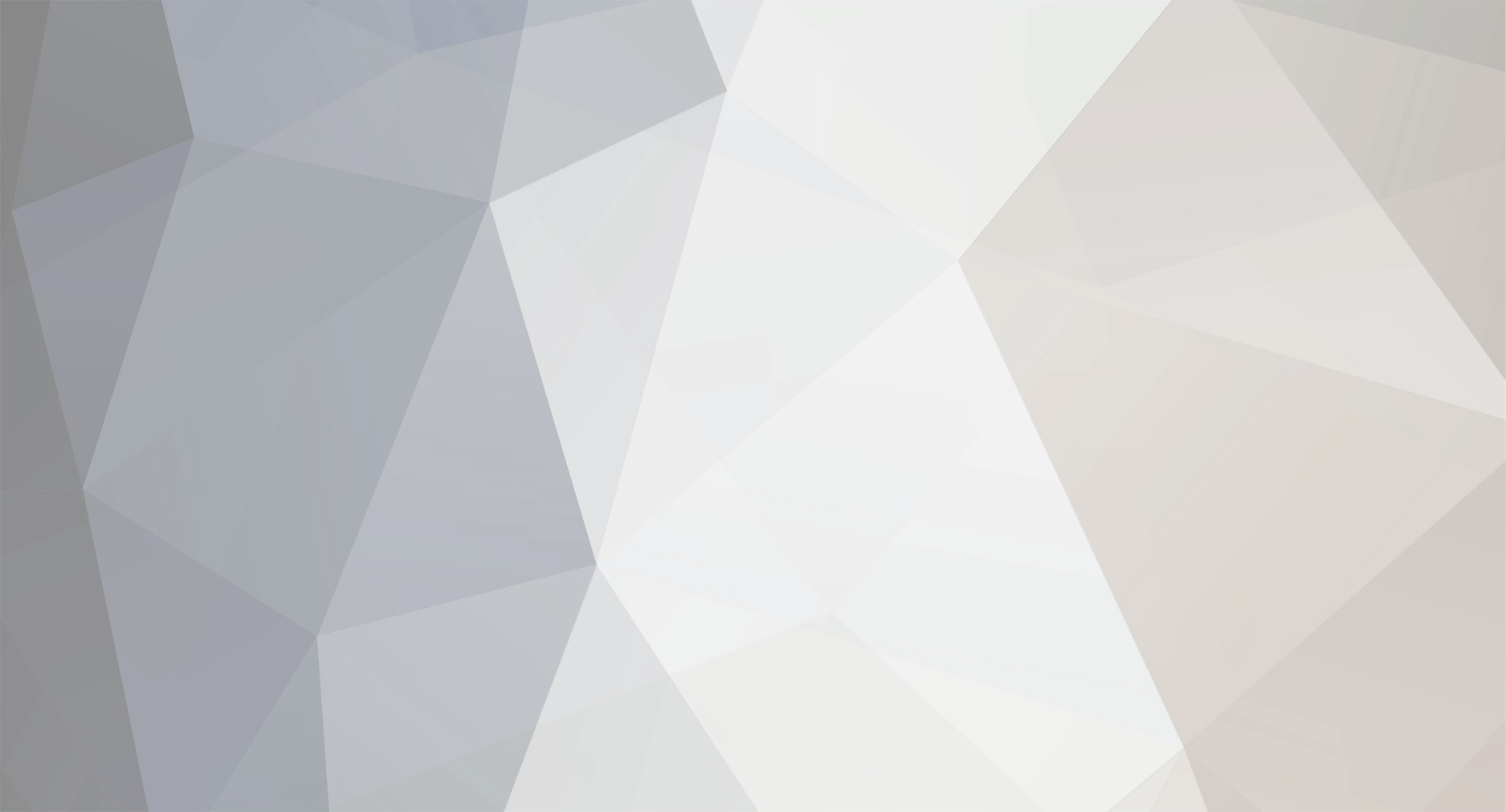
Alexiy
Forge Modder-
Posts
198 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Alexiy
-
Why is NetherBrick's harvestTools equal to null?
Alexiy replied to Insane96MCP's topic in Modder Support
Javadoc of getHarvestTool() says: "Queries the class of tool required to harvest this block, if null is returned we assume that anything can harvest this block." Null doesn't mean that tool is not effective, efficiency and harvestability are separate properties in minecraft. Take a look at net.minecraft.item.Item#getHarvestLevel() and net.minecraft.item.Item#getStrVsBlock(). You should probably use those. -
There might be other mods interfering in rendering, setting/unsetting certain GL switches.
-
give a target player potion effect onItemRightClick.
Alexiy replied to mar_uku's topic in Modder Support
Indeed, it's just an example. Helpful or not, it is for asker to decide. -
give a target player potion effect onItemRightClick.
Alexiy replied to mar_uku's topic in Modder Support
player.addPotionEffect(new PotionEffect(MobEffects.NAUSEA,60)); -
Can you show screenshot of the model in inventory?
-
I guess it's for sorting the event listeners - those who have higher priority will be notified of event before those having the lower.
-
If you want to know how to implement pipes, the common approach is create a pipe block and a tile entity with fluid handling capability. The pipe's tile entity should fill adjacent fluid-capable blocks, and you might want to prevent presence of more than one fluid in a pipe network. This can be done by binding the pipe to a fluid's name or fluid stack when a fluid enters it the first time. I don't know how to render liquids in pipe, though - never attempted it.
-
So, fence block has these boolean properties: /** Whether this fence connects in the northern direction */ public static final PropertyBool NORTH = PropertyBool.create("north"); /** Whether this fence connects in the eastern direction */ public static final PropertyBool EAST = PropertyBool.create("east"); /** Whether this fence connects in the southern direction */ public static final PropertyBool SOUTH = PropertyBool.create("south"); /** Whether this fence connects in the western direction */ public static final PropertyBool WEST = PropertyBool.create("west"); And in public IBlockState getActualState(IBlockState state, IBlockAccess worldIn, BlockPos pos) { return state.withProperty(NORTH, canFenceConnectTo(worldIn, pos, EnumFacing.NORTH)) .withProperty(EAST, canFenceConnectTo(worldIn, pos, EnumFacing.EAST)) .withProperty(SOUTH, canFenceConnectTo(worldIn, pos, EnumFacing.SOUTH)) .withProperty(WEST, canFenceConnectTo(worldIn, pos, EnumFacing.WEST)); } "canFenceConnectTo()" returns a boolean which is passed to "withProperty()" method and appropriate bounding boxes are added or removed when a neighbor block changes.
-
1. I believe you must do stuff by adding a scheduled task to server world: @Override public void execute(MinecraftServer server, ICommandSender sender, String[] args) throws CommandException { WorldServer world= (WorldServer) sender.getEntityWorld(); world.addScheduledTask(() -> { EntityPlayer player= (EntityPlayer) sender.getCommandSenderEntity(); player.addVelocity(0,3,0); //the value 10 is too much, players will be sent into sky with it }); } 2. You act only on the player who ran the command. If you want to launch all players, retrieve them from world.playerEntities or from bounding box.
-
If you want to add trades to existing villager professions, you need: 1. Implement EntityVillager.ITradeList 2. Get the profession from ForgeRegistries.VILLAGER_PROFESSIONS 3. Get career from the profession 4. add the ITradeList to the career - #addTrade. Example below: Implementation which adds 60 emeralds -> 1 Wither skull trade: public class Trades implements EntityVillager.ITradeList { @Override public void addMerchantRecipe(IMerchant merchant, MerchantRecipeList recipeList, Random random) { recipeList.add(new MerchantRecipe(new ItemStack(Items.EMERALD,60),new ItemStack(Items.SKULL,1,1))); } } Then getting a specific profession - nitwit in this case: VillagerRegistry.VillagerProfession nitwit=ForgeRegistries.VILLAGER_PROFESSIONS.getValue(new ResourceLocation("minecraft:nitwit")); And adding the trade to career with id "5": nitwit.getCareer(5).addTrade(1,new Trades()); Figure out desired career and profession by inspecting vanilla classes.
-
Preventing Cascading Worldgen with Large, Randomized Structures
Alexiy replied to TheXFactor117's topic in Modder Support
Have you looked at mineshaft code? Might give some hints, too. It's kind of difficult to abstractly imagine how your algorithm would work, so just do it and we'll see how it goes. -
Preventing Cascading Worldgen with Large, Randomized Structures
Alexiy replied to TheXFactor117's topic in Modder Support
In theory, when an event is fired to which your generator is subscribed to, you should be able to get chunk's X an Y. Then while you generate the structure, you have to continuously check whether the next generated block will trigger generation of new chunk. If the blockpos is within current chunk, proceed generation; if not, then store this blockpos and maybe chunkpos in a map, perhaps. Then on next event you check whether the new chunkpos corresponds to any of the stored chunk positions and proceed generation if it does. You can also find a way to track current chunkpos of player and based on it, pick chunks from the loaded ones and do your thing more safely - still, will have to start checking once the chunk positions are close to the border. -
I'm not an expert on particles, but I know that you can spawn them by World#spawnParticle.
-
Why is it uncommon to see overriden 'equals' method in minecraft classes? Instead different method(s) are provided, for example, ItemStack - 7 methods. They could override its 'equals' as well, so stacks are not so painful to use in collections. Or is it a common 'practice' in Java programming?
-
Let's say I have a server command which starts a Thread @Override void execute(MinecraftServer server, ICommandSender sender, String[] args) throws CommandException { WorldServer world=sender.entityWorld as WorldServer if(args) { if(args.size()==1) { String action=args[0] ChunkPos chunkPos=new ChunkPos(sender.position) int chunkx=chunkPos.chunkXPos int chunkz=chunkPos.chunkZPos if(action==STOP_GENERATION) { if(landGenerator && landGenerator.isAlive()){ landGenerator.interrupt() sender.sendMessage(new TextComponentString('Sent stop signal')) } } } else if(args.size()==2) { BlockPos startposition=sender.position String action=String.valueOf(args[0]) if(action==START_GENERATION) { int range = parseInt(args[1]) landGenerator = new LandGenerator(world, range, startposition,sender) world.addScheduledTask(landGenerator) } } else if (args.size()==3) { String s=String.valueOf(args[0]) int cx=parseInt(args[1]) int cz=parseInt(args[2]) } } } This is a thread which generates world chunks (don't ask me why) class LandGenerator extends Thread{ WorldServer serverWorld int range BlockPos centerPosititon int x1=0,x2=0,z1=0,z2=0 ICommandSender commander Runtime runtime=Runtime.getRuntime() LandGenerator(WorldServer worldServer, int radius,BlockPos center,ICommandSender sender) { super("Land generator") Chunk centerchunk=worldServer.getChunkFromBlockCoords(center) ChunkPos chunkPos=centerchunk.getPos() serverWorld=worldServer range=radius centerPosititon=center int x=chunkPos.chunkXPos int z= chunkPos.chunkZPos x1=x-range z1=z-range x2=x+range z2=z+range commander=sender } @Override void run() { commander.sendMessage(new TextComponentString("Started generation in $range chunk range")) IChunkGenerator chunkGenerator= serverWorld.getChunkProvider().chunkGenerator int chunkamount=0; ChunkPos chunkPos=new ChunkPos(centerPosititon) d: for (int xx = x1; xx < x2; xx++) { for (int zz = z1; zz <z2 ; zz++) { ChunkPos curpos=new ChunkPos(xx,zz) boolean generated= serverWorld.isChunkGeneratedAt(xx,zz) if(!generated){ Chunk chunk=chunkGenerator.provideChunk(xx,zz) serverWorld.chunkProvider.chunkLoader.saveChunk(serverWorld,chunk) generated=serverWorld.isChunkGeneratedAt(xx,zz) if(generated) { println("Generated chunk at $xx, $zz") chunkamount++; long freememory=runtime.maxMemory()-(runtime.totalMemory()-runtime.freeMemory()) float freeMBs=freememory/1024/1024 println(freeMBs+" MB") if(freeMBs<300){ commander.sendMessage(new TextComponentString("Generation terminated due to low free memory")) break d } } } } } commander.sendMessage(new TextComponentString("Generated $chunkamount chunks")) } @Override void interrupt() { super.interrupt() commander.sendMessage(new TextComponentString('Generation interrupted')) } } While the thread is working, I can't execute any other commands. Is there a solution to this?
-
1.10.2 How do I attach spawn my structure in a cave system;
Alexiy replied to TheRPGAdventurer's topic in Modder Support
Take a look at ChunkProviderOverworld. There are several events fired, which you can subscribe to and generate your stuff in their context. -
Just a reminder for JSON texture domains...
Alexiy replied to Winseven4lyf's topic in Modder Support
There is a minecraft section in StackOverflow? Why there? -
I'm using the annotation config. Is it possible to define the type and range for map's values?
-
All my 1.10.2 Mods on the same workspace - Is it possible?
Alexiy replied to American2050's topic in Modder Support
I'm using Intellij and I hate eclipse. -
All my 1.10.2 Mods on the same workspace - Is it possible?
Alexiy replied to American2050's topic in Modder Support
I have this layout too, although there is a slight problem. Whenever I build inner mod, it messes up something in root project, so it becomes unusable in the game (getting abstract method errors and such), so I must build it from terminal (./gradlew :build) to make it usable again. I haven't investigated the issue much, ain't nobody got time for that. -
Regarding Minecraft 1.12, And policy changes.
Alexiy replied to LexManos's topic in Site News (non-forge)
Don't you think that this will also cause an increase of coremods, because more people will learn how to use ASM? I would add another recommendation for coremod makers - just provide configs for coremods with options to disable them or some of their features. -
[1.10+] Is it necessary to put modid in unlocalized name?
Alexiy replied to MrBendelScrolls's topic in Modder Support
It only matters when some other mod happens to have same translation key as in your mod. Chance of this depends on an amount of mods played (still low chance in my opinion). -
So I should add 8 to x and z of provided position? I could subclass Worldgenminable, but I can only override its #generate() method and still will have to copy original generation code and adapt it.
-
[1.10.2] Exporting a library with the mod's jar
Alexiy replied to JimiIT92's topic in Modder Support
If you want to try this out, then I suggest instead of bundling it, put it into mods folder. Forge should load it too, as long as it is Jar. -
I've added vanilla ore generation to the Nether (via DecorateBiomeEvent.Post) , but initially I have been getting the cascading worldgen message. So I found out that one has to apply offsets to the passed in block position. I looked at WorldGenMinable#generate, but it is quite obscure. I copied some parts of it and set the amount of blocks generated to 1. Here is what I currently have: @SubscribeEvent public void generateOresInNether(DecorateBiomeEvent.Post decorateBiomeEvent) { World world=decorateBiomeEvent.getWorld(); if(world.provider instanceof WorldProviderHell) { int numberOfBlocks=7;//FT.defaultGenerationSettings.get; Random random=decorateBiomeEvent.getRand(); BlockPos someposition=decorateBiomeEvent.getPos(); someposition=someposition.add(random.nextInt(16),random.nextInt(108)+10,random.nextInt(16)); float f = random.nextFloat() * (float)Math.PI; double d0 = (double)((float)(someposition.getX() + 8) + MathHelper.sin(f) * (float)numberOfBlocks / 8.0F); double d1 = (double)((float)(someposition.getX() + 8) - MathHelper.sin(f) * (float)numberOfBlocks / 8.0F); double d2 = (double)((float)(someposition.getZ() + 8) + MathHelper.cos(f) * (float)numberOfBlocks / 8.0F); double d3 = (double)((float)(someposition.getZ() + 8) - MathHelper.cos(f) * (float)numberOfBlocks / 8.0F); double d4 = (double)(someposition.getY() + random.nextInt(3) - 2); double d5 = (double)(someposition.getY() + random.nextInt(3) - 2); float f1 = (float) 6 / (float)numberOfBlocks; double d6 = d0 + (d1 - d0) * (double)f1; double d7 = d4 + (d5 - d4) * (double)f1; double d8 = d2 + (d3 - d2) * (double)f1; double d9 = random.nextDouble() * (double)numberOfBlocks / 16.0D; double d10 = (double)(MathHelper.sin((float)Math.PI * f1) + 1.0F) * d9 + 1.0D; double d11 = (double)(MathHelper.sin((float)Math.PI * f1) + 1.0F) * d9 + 1.0D; int j = MathHelper.floor(d6 - d10 / 2.0D); int k = MathHelper.floor(d7 - d11 / 2.0D); int l = MathHelper.floor(d8 - d10 / 2.0D); int i1 = MathHelper.floor(d6 + d10 / 2.0D); int j1 = MathHelper.floor(d7 + d11 / 2.0D); int k1 = MathHelper.floor(d8 + d10 / 2.0D); BlockPos setPosition=new BlockPos(i1,j1,k1); IBlockState blockState=world.getBlockState(setPosition); while(blockState==Blocks.AIR.getDefaultState() || blockState==Blocks.LAVA) { setPosition=setPosition.down(); if(setPosition.getY()<3) break; } world.setBlockState(setPosition,blocks.get(random.nextInt(blocks.size()-1)).getDefaultState()); } } How should I offset given position to be able to generate ore clusters without triggering unneeded generation?