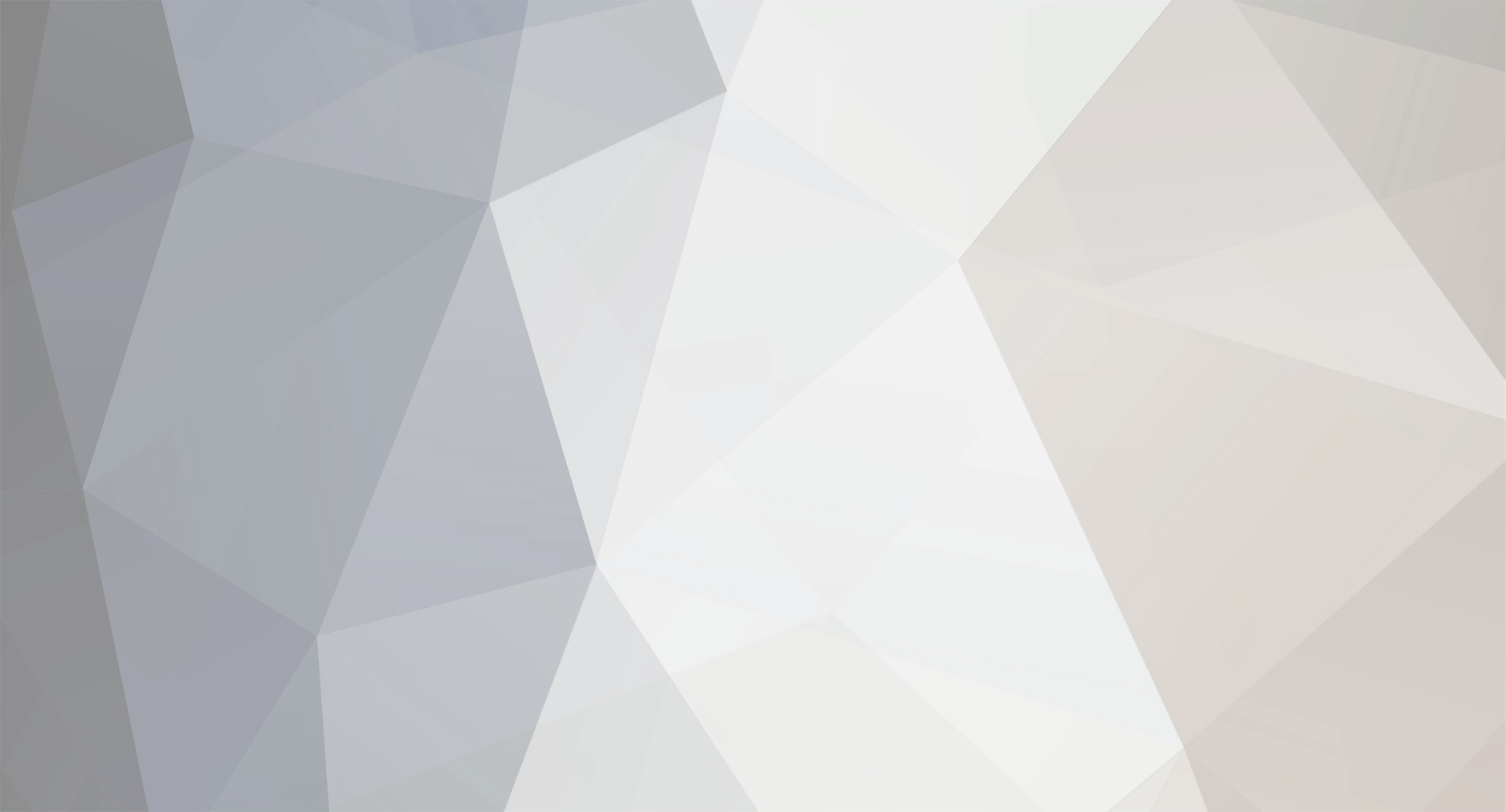
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[1.8] Custom entity attacking when attacked
coolAlias replied to expert700's topic in Modder Support
Just FYI, null checks are automatically accounted for when using instanceof: if (source.getEntity() instanceof EntityPlayer) { // The entity was an EntityPlayer instance, i.e. both not null and an EntityPlayer type } else { // entity was either null or some other type that could not be cast to EntityPlayer } -
[1.8][SOLVED]Issues with item that spawns entities
coolAlias replied to The_Fireplace's topic in Modder Support
Have you tried using your IDE's debugger? The problem is likely simply one of logic, i.e. a value is not what you expect it to be. Furthermore, simplifying / rewriting your logic would likely help make things clearer. I don't really see the point of all those conditions in your while loop, for example: // Is it really necessary to store the ammo type in ExtendedPlayer? Why can't you just fetch it each time you right click? EnumAmmo ammo = ExtendedPlayer.get(playerIn).getAmmoType(); // can this ever return null? // This loop already changes the item each time, making all those conditionals redundant and messy // Also, you have an EnumAmmo instance on hand, so why make static methods when you can use the instance itself? // E.g. ammo.getItem() instead of EnumAmmo.getItem(ammo) while (!playerIn.inventory.hasItem(ammo.getItem())) { // no need to involve player here, just fetch the next ammo type and see if the player has it ammo = ammo.getNext()); // You will need, however some way to stop the loop, e.g. if ammo loops back around to its original value, set it to null and break } if (ammo == null) { return itemStackIn; } // set the ExtendedPlayer ammo value at this point, if you still want to do that ExtendedPlayer.get(player).setAmmo(ammo); // You've already checked that the player has the item needed, right? Don't need to check again, then. YourCoalEntity entity = ammo.getCoalEntity(worldIn, playerIn); // make your Enum do some work for you if (entity != null && !worldIn.isRemote) { worldIn.spawnEntityInWorld(entity); // ammo already knows the item, right? So why hard code them all? playerIn.inventory.consumeInventoryItem(ammo.getItem()); } return itemStackIn; You could probably even consolidate all of those different entities into one class, depending on how different they are, simply by setting different class attributes based on the ammo type: setDamage(based on ammo type), setVelocity(based on ammo type), etc. Sorry I kind of went off on a tangent, there, but I think it would be worth your while to do some refactoring here. In the meantime, you should be able to pinpoint your issue by using the debugger. -
Well, I can at least say I've been making progress in understanding the model system. Hilariously in an embarrassing sort of way, for whatever reason it took me a while to realize that I could return 'this' from ISmartItemModel#handleItemState or ISmartBlockModel#handleBlockState. I kept wondering why my methods weren't working properly, and it was because I was returning the model used to construct the smart model, rather than the smart model itself. Derp. Don't know what the hell was going on, but definitely feeling retarded While I don't feel quite ready to tackle the bow + arrow thing yet, I did get my shields working: First person: Third person: I registered two .json models for each shield, a standard one and one for when it is in use, both of which are swapped out to use the same ISmartItemModel class. In there, all I do is swap the last quad from the default model with the face quad for the 'back' model (which does have a .json for the texture, but none of its transformations / rotations are used). @Override public List<BakedQuad> getGeneralQuads() { List<BakedQuad> quads = shieldFront.getGeneralQuads(); quads.set(quads.size() - 1, (BakedQuad) shieldBack.getGeneralQuads().get(1)); return quads; } Pretty simple, really, though it took me quite a bit of time to get the .json rotations correct for the blocking position due to the vanilla rotations throwing everything out of whack. Turned out pretty nicely, though, and not nearly as verbose as I was expecting. Btw, TGG, your camera transform tool was extremely handy while working on this, even though it was unable to dynamically adjust the shield models. The json used for the 'blocking' version didn't even register when pressing 'reset', so I had to enter it in manually each time with a sword or some other item I could block with. Still, very very helpful, so thanks for making that. Is there any way to adjust rotations by increments of 1 or less while using it? Or just type the value in directly? That would be handy if you feel like doing some more work
-
That's not generics, that's simple inheritance (which should be fine for your purposes), but you CAN NOT cast to the specific tile entity class. That defeats the whole point. If you need a container that has access to methods / fields specific to your TileEntityElectrolyser class, make a container specifically for that class - the container can extend your super container, and take the specific TileEntityElectrolyser as a constructor parameter.
-
Use generics, or just use your 'super tile entity' class as the parameter type. Keep in mind that you will only be able to use methods / fields from that type, so if any of your tile entity classes have special methods or fields, they will not be accessible without casting to that type. What you're trying is really only useful if there is a lot of common behavior and you don't need it to do anything specific based on the actual class involved.
-
I think Elix's answer covered that already: If you are having trouble using it, post the code that you have tried. You will need to put that method in your SWORD class, btw, to return a SHIELD icon when in use (if (player.isUsingItem())
-
When you're ready to attempt the advanced version, I do actually have a couple of tutorials that will get you well on your way: 1. IExtendedEntityProperties 2. Custom player inventory (e.g. shield slot) 3. Packets 4. Example of all of the above That should be enough to get you a shield slot (or wait for 1.9? ), then it's up to you to figure out when the player has a shield equipped in that slot and handle any rendering that needs to be done. Good luck!
-
You can't check directly, but you can check if (player.isUsingItem()) which is effectively the same thing.
-
It tries to load the class that failed and, when it undoubtedly fails again, prints a usable stack trace in the crash log.
-
So even using GlStateManager for the OpenGL calls uses the fixed pipeline? I would have expected it to queue them up along with the rest of Minecraft's rendering calls. I do use the current system for the vast majority of my items and blocks, but those few cases that require special handling are really causing me a headache, which is why I end up resorting to hacks like the one above from time to time. Your comments about the fixed pipeline are causing me to question some other things that I thought were perfectly fine, e.g.: Should we not be using GlStateManager at all? If that's the case, I'm in for a very rough time. Me + rendering != happiness. Never has for me, and some parts have only gotten much more complicated with this update; others, though, like changing scale, rotation, and translation for a model, have become deliciously trivial thanks to the JSON formats. I really hope they don't change to that for entities, though...
-
To expand a little on what Ernio said, DamageSource contains information about the entity that the damage was caused by. source.getEntity() is always (in vanilla implementations, at least) the entity that is ultimately responsible for the damage, such as the player entity who released the arrow entity that hit you. source.getSourceOfDamage() returns the entity directly responsible for the damage; in the example above, it would give you the arrow entity. I've seen the difference between those two trip quite a few people up.
-
Ah, I see. Well, that should work if you're already writing to the containing ItemStack's tag compound. At this point, I suggest comparing your code to mine method by method and seeing what is different. Sorry I can't point out specifically what is wrong, but my guess is it has something to do with your TagStaff object.
-
Post the crash log, too. Is it a NullPointerException? Make sure you initialize your 'color' field.
-
To expand upon diesieben's comment, you need to compare the Item and possibly the damage value and maybe even the NBT tag of the ItemStacks in question in order to determine equality. Usually, you don't care if the ItemStacks are actually equal, but rather that the Item it contains is the same. In your case, you want to know if the ItemStack contains an ItemShield item (assuming that's the name of your shield item class), so you'd have to first check if the stack is null, and then check if stack.getItem() is an instanceof your ItemShield class, or you could compare it directly to YourItems.shield: ItemStack stack = player.getHeldItem(); if (stack != null && stack.getItem() instanceof ItemShield) { // Alternatively: if (stack != null && stack.getItem() == ItemHandler.shield) { Using instanceof is more flexible, as it will handle ALL shield items with one check, whereas the latter you have to check specifically for each item you want to handle, making it a pain if you (or another modder using your mod) ever add more shields.
-
Minecraft uses OpenGL under the hood to do all of its rendering, so how is my code any slower or deprecated? What else are we supposed to use, such as for animation frames in TESR where there might not be a model, or if we have a model but want to move / rotate it? My code is most certainly hard-coded, and I will change it (eventually) to use the model system, but even then I would be dynamically generating a new model each frame, because each arrow item could theoretically have a different model, so I couldn't bake every possible combination ahead of time, could I? Why would this be any faster than using GLStateWrapper directly on a pre-baked model? I admit that my solution is not perfect and abstraction layers are certainly useful, but only if people can figure out how to use them; too many layers / too many pieces that must all be used in just the right combination at just the right time and registered in just the right way without any documentation makes it awfully difficult for people to get all their ducks in a row, especially when you step outside the standard model format. Sorry, it's just a very unintuitive and frustrating system for me, and I've already spent more hours than I care to admit wrestling with it and getting nowhere. Grammar Nazi alert: gets, not get's. Apostrophe + s is used for contractions and possessive nouns, not as a verb ending. Just FYI, in case you care
-
After looking through the panorama of classes involved for over an hour, that does seem to be like the way, but my lord, that is complicated, or at least appears that way when trying to figure it out for the first time. For example, this is all it takes for me to have it working right now: Simple and effective. Converting that into a proper model format would likely take me hours of frustration (it already has, actually, and in the end I didn't get anywhere with it). I think I'll stick with my hack for now, and give it another go when I have more time / patience. Perhaps by then TGG will have released a comprehensive guide on IModel. Re: components vs quads - I realize it's about quads, I was just referencing them by the component models because that's where I'd be getting the quads from, and all of the quads within that component would undergo the same transformations. Anyway, thanks for your efforts here. One of these days, I really will try to tackle this problem the correct way @Ernio That looks awesome, nice work!
-
Hm, I'm running into a couple of issues, and hopefully I'm just proving mentally deficient. 1) Item#getModel needs to return a ModelResourceLocation only, so it cannot (that I can figure out) return a combined baked model 2) If I do make a combined model, how can I return the correct texture atlas sprite for each component? It seems that I can only return one. 3) In the combined model, which method is suitable to go about rotating / translating a specific component? In the meantime, I'm going to try rendering the arrow stack directly from within Item#getModel. I know that's terrible form, but at this point, I just want to get the damn thing working. EDIT: Holy hell, it actually worked! Hahaha. Now I just have to get the rotations right, and f-you model system!!! Direct rendering, ftw! In all seriousness, though, if anyone knows a proper way to accomplish this, please let me know. But at least I can move on to other things for now, and leave my hack as fodder for the code police.
-
You can iterate through the GameRegistry and check for blocks to add to your inventory - I would use a regular Container / IInventory / GuiContainer triplet to handle it, then you won't need to worry about rendering at all. However, if you do choose to go with direct rendering, it is still possible - check out RenderSnowball. It uses a RenderItem instance (which you can get from Minecraft class) and then you pretty much just call 'renderItem.renderItemModel(ItemStack)'. Of course you'll want to mess around with it some to make it look nice in the GUI if it doesn't naturally, in which case I'd recommend you to check out GuiContainer.
-
Thanks TGG, that's what I was going for. Still, it makes my head ache just looking at your code :\ I'll see what I can come up with this weekend.
-
The side that sends the packet knows about the golem entity, but the side that receives it is a new instance of your message class and doesn't know about anything until it reads in the packet data. For an entity like that, you need to send the entity Id (i.e. entity.getEntityId()) with the packet as well, then read that integer in during read, and retrieve the entity from the world when the packet processes. Entity entity = player.worldObj.getEntityById(this.entityId); if (entity instanceof EntityGolem) { // continue processing } else { // log error message } Get the player from the current context via your proxy classes: see here and the ClientProxy implementation as well. That said, if all you want to do is set the attack timer on the client side for your golem entity, use worldObj.setEntityState(customFlag) and handleHealthUpdate(byte flag) - they work in tandem to send byte flags to the client side, allowing the entity to do different things like display heart particles when in love or set the attack timer for the arm-swing animation like golems. You can see how the vanilla golems do it just like that, and I prefer that to a packet when I can (though it sends a packet in the background) because it keeps everything encapsulated in the class.
-
[1.8] Problem loading models for items with metadata
coolAlias replied to zlotnleo's topic in Modder Support
You also need to register all of your item variants to ModelBakery.addVariantName(yourItem, String[]{variant1, variant2, etc.}). Each variant should be the model name qualified by your mod id, e.g. 'yourmodid:your_item_variant_1'. -
Sorry, but I don't see how that allows me to return two models at a time - perhaps I just am not understanding the interface. I need both the bow AND the arrow model to render together, not just one or the other. Or is there some way to dynamically merge the two models together and return them as one? I'm trying to make the bow compatible with possibly unknown (beforehand) arrow items, otherwise I could just make each of the 4 bow textures per arrow and return that model from getModel. Not very elegant, but it would work. I understand the new model formats are there to allow greater flexibility for resource pack makers, but trying to do any sort of customization has been an extremely frustrating experience. It's getting to the point that I may just resort to hackery and see if I can render the arrow before returning the model from Item#getModel. Thanks for the replies so far, and apologies again that I just don't seem to get it - rendering-related code has never been my strong suit.
-
Sorry, the Minecraft Forum editor seems to have garbled most of the tutorial. Here is the original, and the following is the most important part, updated for 1.8: /** * For inventories stored in ItemStacks, it is critical to implement this method * in order to write the inventory to the ItemStack's NBT whenever it changes. */ @Override public void markDirty() { for (int i = 0; i < getSizeInventory(); ++i) { if (getStackInSlot(i) != null && getStackInSlot(i).stackSize == 0) inventory[i] = null; } writeToNBT(invStack.getTagCompound()); } In your inventory class, you did not put anything at all in markDirty(), which is why your inventory is not saving.
-
There absolutely is a difference: onItemUse is only called when the player clicks on a block, not in the air; onItemRightClick is called for both. You CAN NOT open the gui on the server from a key press. Read my last reply, read the tutorial. Besides, why are you bothering with a key press when the player is already right-clicking with the item? Just open the gui onItemRightClick.