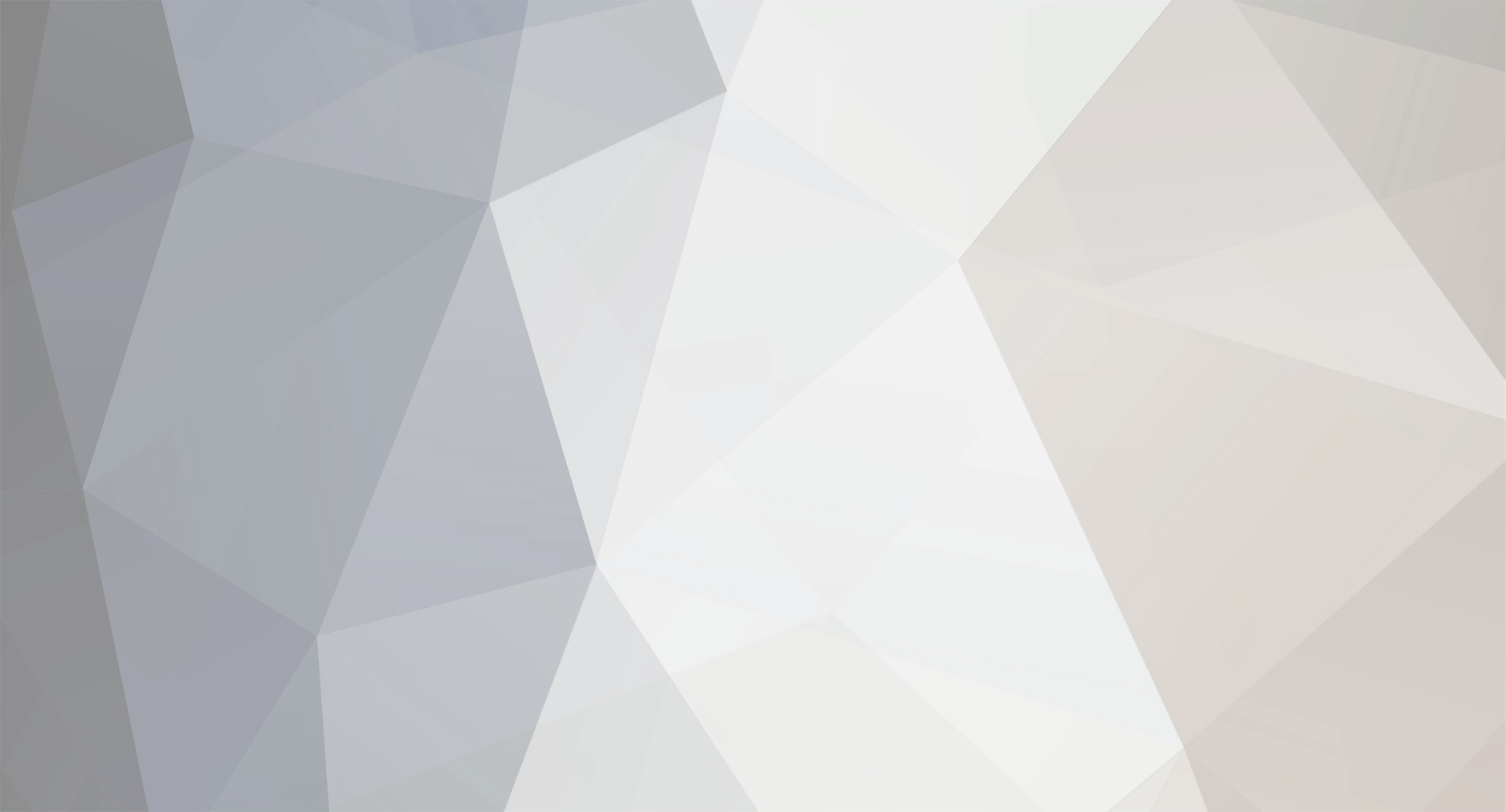
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[1.7.10] Consume item in hand instead of just any item?
coolAlias replied to dude22072's topic in Modder Support
You can also use player.getHeldItem() to get the currently held item, reduce the stack size as diesieben mentioned, and if it's null, use player.setCurrentItemOrArmor(0, null), because that always sets the currently held item to whatever the second parameter is. Or you could play with player.inventory.currentItem, but I find the above more expedient -
That does seem odd, but at the same time it is very common for random number generators to generate the same number multiple times in a row (up to 7 times in a row, actually, according to some article I read somewhere...) - it's a common misconception that random means every number is different. Especially with such a small range (0-4), you are bound to end up with lots of repeats, and Random will always output the same sequence of numbers given the same seed. Whether that means you don't have an issue or not, I don't know, but it is very much within the realm of randomness to get numbers that don't seem quite random.
-
This is exactly why you should ALWAYS use the @Override annotation on any inherited method - it will tell you right away in your IDE that the method does not exist if the signature is incorrect, which you fix not by removing @Override, but by finding the correct method signature
-
Multiple things happening when space is pressed
coolAlias replied to Asweez's topic in Modder Support
Why not just use the regular vanilla jump key? You can handle that in a KeyInputEvent just like you would a custom key binding, with the advantage of being very intuitive for players (jump key jumps for everything) and still customizable. Another alternative you may consider is that you could simply let the player jump as normal while riding your vehicle, and then have the vehicle y motion match the player's, then you wouldn't need any key handling at all. I doubt that's how horses / minecarts work, but depending on what your vehicle is (e.g. a pogo-stick ), it could be an option. -
You can also check KeyBinding#isPressed() to see if the key was pressed - this will decrement the pressed value (which should only have been at 1 since it was just pressed), then unset it with setKeyBindState as you were doing. Another thing to keep in mind is that the KeyInputEvent fires TWICE for each key press: once when the key is pressed (that's the one you want to intercept), and once again when it is released. Use Keyboard#getEventKeyState to check which state (press or release) the key is in. The trouble, I think, may be that KeyInputEvent, since it is not designed to be cancelable, fires too late to stop the client's action from sending a packet to the server, and when that packet is received, it no longer cares about the state of any client-side input. You may have to take some more drastic measures and attempt to intercept this packet, or just call it 'good enough' to expect most users to be using the LMB (mouse) to attack, and cancel the MouseEvent when appropriate. At least that way, you can move on to other things, then come back to this issue if users of your mod complain about it (very few people don't use the mouse, but they do exist...).
-
Oh yeah, you're right - KeyInputEvent does NOT fire for mouse keys, but there was a PR I saw that was going to change that. Anyway, you need to listen for both KeyInputEvent AND MouseEvent, whichever one receives the keyBindAttack will need to handle preventing the interaction; MouseEvent you can cancel directly and that will 100% for sure work, but I'm not sure how unpressing the key from KeyInputEvent will work in the event that someone is using a non-mouse key to attack.
-
... Clearly you can see from the code that it uses the owner's UUID for saving and loading... so I'm not sure what your point is.
-
If your item is still not rendering, try first using RenderSnowball class to render your rock item when thrown. Then at least you can rule out problems with your main classes, registrations, etc. and know that the problem is in your rendering class. For the arm not swinging, try TrashCaster's suggestion above.
-
It sounds like you just want to know if your player is wearing ALL of the pieces at once to then do something, no? In that case, whenever you want to do that special thing, simply check each piece of worn equipment on the player: ItemStack helm = player.getCurrentArmor(3); // etc. for each piece, then check each: if (helm != null && helm.getItem() == YourMod.yourCustomHelm && // etc., all the other pieces) { // do your special thing here } If, for whatever reason, you actually need to know which specific parts of your custom armor are being worn at all times without manually checking the player's armor slots all the time (which isn't really that big of a deal), then I recommend storing that information in IExtendedEntityProperties, but you really have to ask yoursef: "Why do I need this information, and why is the current method of getting that information not sufficient for my needs?" In 99% of cases, you will find that the current methods (see above) are more than sufficient.
-
He's gone over this before: it does not work because PlayerInteractEvent is not called on the client when left-clicking a block, so on the client it still looks like the block breaks for a split second and then reappears after the server re-updates the client saying 'hey, that didn't actually break.' Jabelar's solution may work, provided that the BreakEvent is fired on the client as well (I haven't checked) and BEFORE the block disappears from the world. Otherwise, the only way to stop the visual glitchiness is to intercept the actual attack key press on the client. @OP The KeyInputEvent will work, as I said, but if the attack key bind is set to the mouse, you have to intercept it from MouseEvent first and cancel that if your conditions are met, because as you found out unsetting the key pressed value is not fast enough to stop the mouse from clicking through.
-
That depends - is your entity using index 17 for anything else? Are any of its super classes using index 17? If you can answer NO to all of those, then yes, you can use it.
-
... you do realize that each index in Datawatcher can only contain one value, right? You need a separate index per value you wish to store.
-
Seems like you're having the same issue as Thornack, and the solution will be the same: when you have a valid owner entity, store the display name in another one of your entity's DataWatcher slots (assuming you need it on the client); then be sure to save that value to your entity's NBT tag when it saves, and load it back when it loads.
-
The situation seems simple enough: if you need the nickname while not logged in, then save/load it via NBT like you do the UUID and store it in another DataWatcher slot; whenever setOwner or the like is called, simply have it update the nickname field as well. Unless you are doing something funky behind the scenes, that's really all it takes: two data watcher fields, one each for the UUID and nickname, and saving / loading each of those to NBT. Just like EntityWolf/Tameable.
-
An entity's NBT data is persistent - that's the whole point of saving to and loading from NBT. You should only need to store the UUID of the owner in NBT, nowhere else, just like in EntityTameable. If you want the player's nickname, you can always get that later as you need it based on the current owner.
-
I realize what your TalentHelper is for, my point is why use it to directly modify the returned speed value when you could instead add an attribute modifier to speed each time your talent changes? This way, you don't have to do ANY calculations at all when returning speed - it is all handled automatically by the SharedMonsterAttribute / AttributeModifiers, which should also automatically be known by the client so you can display the appropriate speed value.
-
You cannot cancel KeyInputEvent, but you CAN set the key state to not pressed. Whether that will happen before the MouseEvent / mouse input is processed, I can't tell you for certain, but you can certainly try it. int kb = Keyboard.getEventKey(); if (kb == mc.gameSettings.keyBindAttack.getKeyCode()) { // Set attack key to not pressed KeyBinding.setKeyBindState(kb, false); } Then, if that doesn't unset the key press in time, you can still listen for the MouseEvent and cancel it if the mouse button pressed is the same as keyBindAttack and your other conditions are met. More economically, make callKeyInputFunctionThatChecksYourConditions() that you can use both in the KeyInputEvent and to setCanceled(resultOfThatMethod) from MouseEvent when clicking the attack key.
-
Instead of TalentHelper and manually adding adjustments for being evolved, why not add speed modifier as part of the talent / when the entity evolves? Then the entity's speed will be adjusted automatically. Otherwise, you will need to send all of your talents and evolved statuses to the client for it to display the bonuses properly.
-
Oh, I remember running into problems like that. I found that using 'event.useBlock = Result.DENY;' works to prevent the block from getting destroyed, whereas canceling the event didn't work as well. Anyway, if you're having issues with the client side, MouseEvent is definitely the way to go except that people can change their keybindings / use a gamepad - a better alternative may be to listen for KeyInputEvent and check for mc.gameSettings.keyBindAttack.
-
Instead of returning false for isCollidable, return null for the bounding box: // 1.7.10 method signature, but probably something similar in 1.8 @Override public AxisAlignedBB getCollisionBoundingBoxFromPool(World world, int x, int y, int z) { return null; } Then your block can still respond to onBlockClicked / onBlockActivated, and you don't need any events at all. I have a fire block that does just that, yet players and other entities can walk right on through it.
-
[1.7.10] TileEntity Mimicing Changes on All Instances
coolAlias replied to Izzy Axel's topic in Modder Support
(looking through the Block class) Hm, what's this? public int getLightValue(IBlockAccess world, int x, int y, int z) Looks like you can store the light level in your TileEntity and retrieve the TileEntity's value with that lovely method from Forge