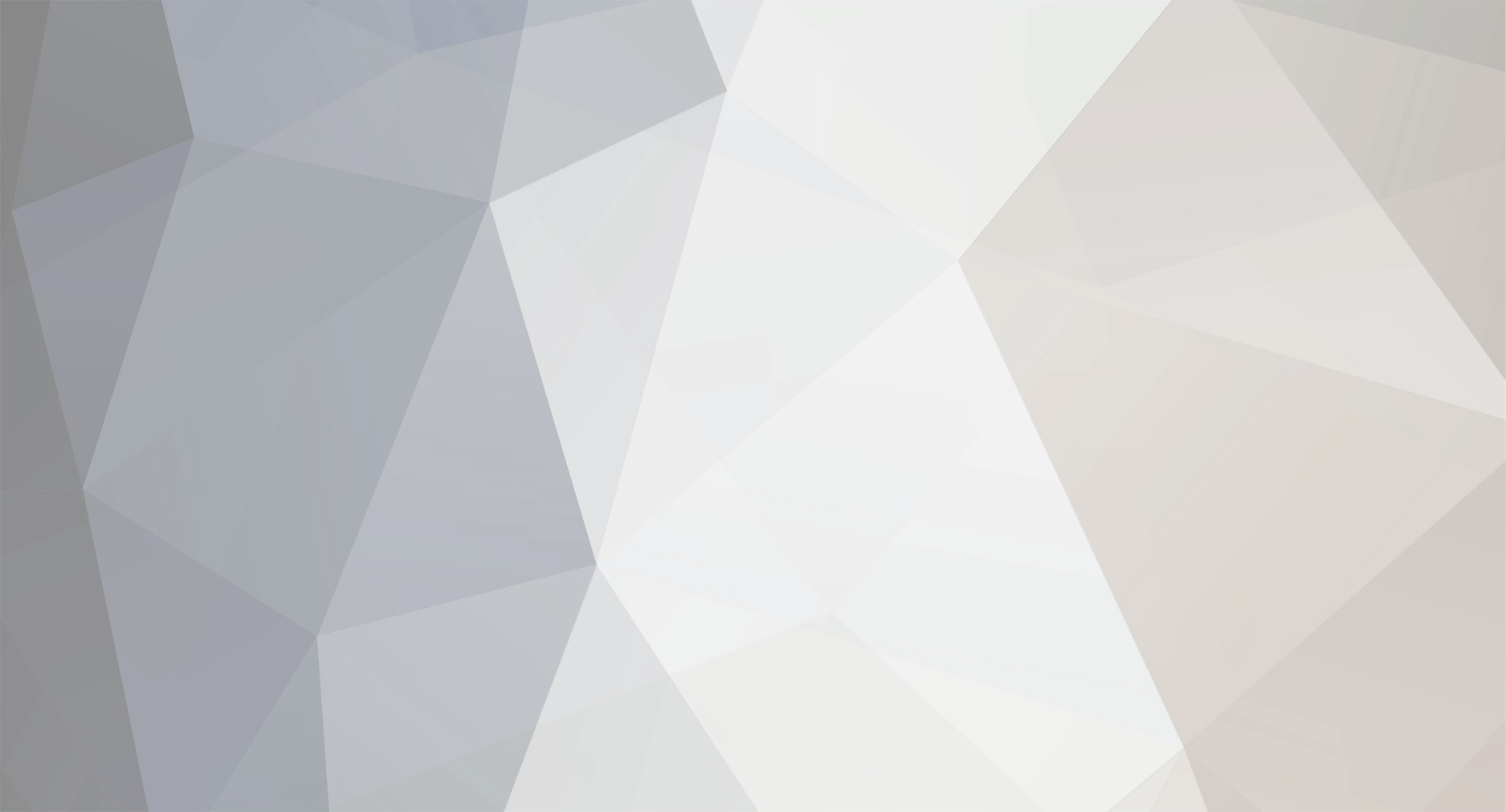
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
You can store Blocks by id: Block.getIdFromBlock(block). For your TileEntity, you can write it to NBT and append the tag to the ItemStack's NBT tag compound when the block is broken and the Block ItemStack is created, then when the stack is placed as a block, get the TileEntity at that position and read from the ItemStack's NBT. I've found the Block methods 'breakBlock' and 'onBlockPlacedBy' to be most useful for this.
-
[1.7] [SOLVED] Problem with item spawning TileEntity
coolAlias replied to Meastroman's topic in Modder Support
BlockTurret turretblock = new BlockTurret(); ... Never create new instances of Items or Blocks - each one should only be instantiated one single time when your mod starts, and every other 'instance' should refer to that instance. This is because Items and Blocks are all declared as static fields. -
Have you tried changing the zLevel at which you render? If your render code is affecting the vanilla rendering, you need to make sure that you disable/re-enable whatever settings you changed in openGL; for example, if you enable BLEND, be sure to disable it when you finish rendering.
-
Yes, you can, but if you want your attribute to be included in vanilla mobs, you will have to add it either via ASM or during EntityJoinWorldEvent, at which point you can add it to the attribute map with whatever value you want.
-
Couldn't you just set "values.put("mana", 200)" in the constructor? I'm a bit confused with your code, to be honest; you seem to be making it much more complicated than you need to - do you really need hashmaps, rather than simply a couple integer fields? Why don't you save the max value as well, does it not ever change?
-
The ItemStack may also be null, and if it's not, then itemstack.getItem() will not ever be null. "if (itemstack != null && itemstack.getItem() instanceof SomeItem)" is safe from null exceptions.
-
Is the 2D entity ONLY in the GUI? If so, then you shouldn't need to sync anything. If not, could you just fake it, as in render the 2D entity based on some random variables you have on the client, or does it absolutely have to be synced to something that is processing on the server?
-
It's pretty straightforward: // I create a local index to make it easy on myself; just increment away int modEntityIndex = 0; // the numbers are a) tracking range, b) frequency of tracking updates; true to send tracking updates // see [url=https://docs.google.com/spreadsheet/pub?key=0Ap8gssssFFPAdFRXREZGSzZRY3k1WE8wcUE4S09xWXc&single=true&gid=0&output=html]this chart[/url] for typical vanilla values EntityRegistry.registerModEntity(SomeEntity.class, "entity1name", ++modEntityIndex, YourMod.instance, 64, 10, true); EntityRegistry.registerModEntity(AnotherEntity.class, "entity2name", ++modEntityIndex, YourMod.instance, 64, 10, true); Keep in mind that doing it this way you will not be able to use the vanilla spawn eggs or summon command for you custom entity (thus the appeal of the global entity id, which I still don't understand why it has not been 'fixed' to allow for more than 256, such as using a String "mod:entityName" for spawn eggs rather than an integer, but I digress), so you will have to create your own spawn egg item(s) and command if you want them.
-
Get a mob to have random texture when spawned?
coolAlias replied to HappleAcks's topic in Modder Support
@OP The render class' getEntityTexture method has an Entity parameter, which is your entity - you can cast it to your custom entity class to access your entity class methods: protected ResourceLocation getEntityTexture(Entity entity) { // cast to your entity YourEntityClass blah = (YourEntityClass) entity; // now you can access class methods, such as my hypothetical getType() which // returns the integer value from datawatcher used to store the mob's type, i.e. texture switch(blah.getType()) { case 1: return texture1; case 2: return texture2; etc; } } DataWatcher is just for storing a single value, in this case an integer, that is synchronized between server and client - you set the value on the server, and all of the player clients will see the same value, in this case texture. @delpi DataWatcher ranges vary from Entity to Entity - players use the most of any, and I tend to start mine at around 21 or 22 just from habit, but if you really need to know exactly which indices are still available, the most sure method of finding out is to open up all the parent classes of the entity in question and look at the constructors and entityInit methods to see which values are used. This page lists some of them, but it may be outdated. @OP - you may want to check out that link as well, it's a tutorial on DataWatcher, though I think SanAndreasP pretty much covered it all -
[1.7.2] Add other mods to build path (Eclipse)
coolAlias replied to xypista's topic in Modder Support
Each mod should be its own separate folder, completely independent of your other mods. See Lex's on multi-project workspaces. If you are trying to use a second mod as a resource in your first mod, as an add-on or something, then you don't need to set it up at all - simply place the mod jar in your /eclipse/mods/ folder, then add it as a referenced library; you will then be able to import the mod's classes and such, though to build your project you will need to edit the build.gradle file to include the referenced mod files. -
Get a mob to have random texture when spawned?
coolAlias replied to HappleAcks's topic in Modder Support
Make sure your texture field is not static, and also that the field is visible / updated on the client. Personally, I use DataWatcher to store an integer that I can retrieve using a method such as 'entity.getType()', and place the switch in my render class as 'switch(((MyEntity) parEntity).getType())' -
Did you try cpw's PlayerLoggedOutEvent? It's a sub-class of PlayerEvent, registered to the FML event bus, just like the PlayerLoggedInEvent.
-
1.7.2 NEED HELP with entities and events
coolAlias replied to zacharyjaiden's topic in Modder Support
Zachary, Most of the time when someone around here encourages you to learn more about Java, we are doing so in an effort to help you, and getting upset about it is not very conducive to learning. As we've discussed before, learning Java is a process, and claiming that you already 'know basic Java', while it certainly contains some truth, is also far enough from reality that believing this is simply going to prevent you from taking the steps necessary to expand your knowledge. I've been coding in Java for almost a year now, and while I have a pretty good grasp of most of the fundamentals, I am still always learning new things, even things that most Java programmers would consider extremely basic (such as the difference between 'instanceof' and 'isAssignableFrom(Class)', which I learned about only a month or two ago). So when you ask a question and someone responds with 'please learn basic Java, what you want is {fill in the blank}', instead of getting upset at that person, take the opportunity to do a search for the Java documentation of whatever they said you needed (e.g. instanceof), and you will not only have shown yourself to be a mature and proactive individual, but you will also have learned valuable information that will make modding much easier, especially if you make it a habit to look things up. On the other hand, if you don't care about learning Java except just enough to make a mod, then you can hardly expect people to take you seriously on a forum such as this. Sorry for the rant, but please understand that help comes in many forms, and the best kind of help is the kind that helps you help yourself. As the saying goes, 'Give a man a fish, you feed him for a day; teach a man to fish, you feed him for a lifetime.' Regards, coolAlias -
The exception is occurring in a custom packet 250; do you send any packets in your mod? If so, you may want to check that your packets and handling thereof. I don't currently have Eclipse open, so this is just speculation, but if I remember correctly, cpw's Player class is used when sending a packet from the server to the client and is only ever cast from the server player entity (EntityPlayerMP), but somehow you have a packet that is trying to cast from the client player, as though you are trying to send a packet to the client from the client.
-
In the Minecraft launcher, select your profile -> edit -> launcher visibility: select "always visible" or whatever option that is, then you can view the same output that you would get in your IDE console in the launcher's "development console" tab. Of course, the usefulness thereof depends on how much information you output to the console from your mod.
-
Make sure you ran "setupDecompWorkspace"; code is located under "Referenced Libraries" in a "forge"-prefixed package.
-
See this post.
-
[1.7.2][UnSolved]Custom Furnace not outputing items
coolAlias replied to dude22072's topic in Modder Support
It looks like your problem is this: if(this.cookTime == this.furnaceSpeed) { this.cookTime = 0; this.smeltItem(); flag1 = true; } else { // RIGHT HERE this.cookTime = 0; } Basically, your code says, "if it's not completely cooked, set the cook time to zero"... rather than letting it increment all the way up to the furnace speed, you set the cook time to zero every single tick. -
Here you go. It's updated for 1.7.2, but there are only two changes you need to make to back-port it to 1.6.4, both related to Item: 1. Add an (int id) to your item constructor 2. Change IIconRegister back to IconRegister (remove the leading 'I') Everything else should work exactly the same.
-
How to make Bow render as bow, and shoot faster.
coolAlias replied to JohnBeGood's topic in Modder Support
You need to use the FOVUpdateEvent, check if the item in use is your custom bow, and calculate an appropriate field of view modifier based on the number of ticks in use. To see the vanilla calculations, look in EntityPlayerSP#getFOVMultiplier(). -
[1.6.4]How can I know when a player leaves a world?
coolAlias replied to Abastro's topic in Modder Support
It was replaced entirely with events -> see cpw.mods.fml.common.gameevent.PlayerEvent (these events need to be registered to the FML bus, not regular EVENT_BUS) -
How to make a weapon [gun] fire faster than default speed?
coolAlias replied to HappleAcks's topic in Modder Support
The reason you don't do that is because that variable will be the same for every single instance of the Item, making it incompatible in multiplayer for sure, and possibly in single player as well if you have two or more of the item in question. @OP Your code is close, but when you right click, you forgot to set the time at which it was fired, meaning that every click will allow it to fire as world time > 0 is always true Add this to your right-click method after determining that you should fire a shot: stack.getTagCompound().setLong("lastShotTime", world.getWorldTime() + delay); // where 'delay' is an integer equal to the number of ticks you want to delay between shots That should now prevent you from spamming right click to fire quickly, while still allowing you to hold right-click and fire continuously while the item is in use. -
How to make Bow render as bow, and shoot faster.
coolAlias replied to JohnBeGood's topic in Modder Support
setFull3D() to make the item be held upright and larger (like swords, tools, bows, etc.). The length of time required to reach full charge is determined by the equation in onPlayerStoppedUsing: // the denominator is how long it takes, in ticks, to reach maximum strength // 20.0F means you need to charge for 20 ticks (1 second) float f = (float) j / 20.0F; f = (f * f + f * 2.0F) / 3.0F; Of course, you will want to change your icons to match whatever timing you decide upon. -
The event is simply a convenient way of getting your world gen class to be called, not a replacement for it.