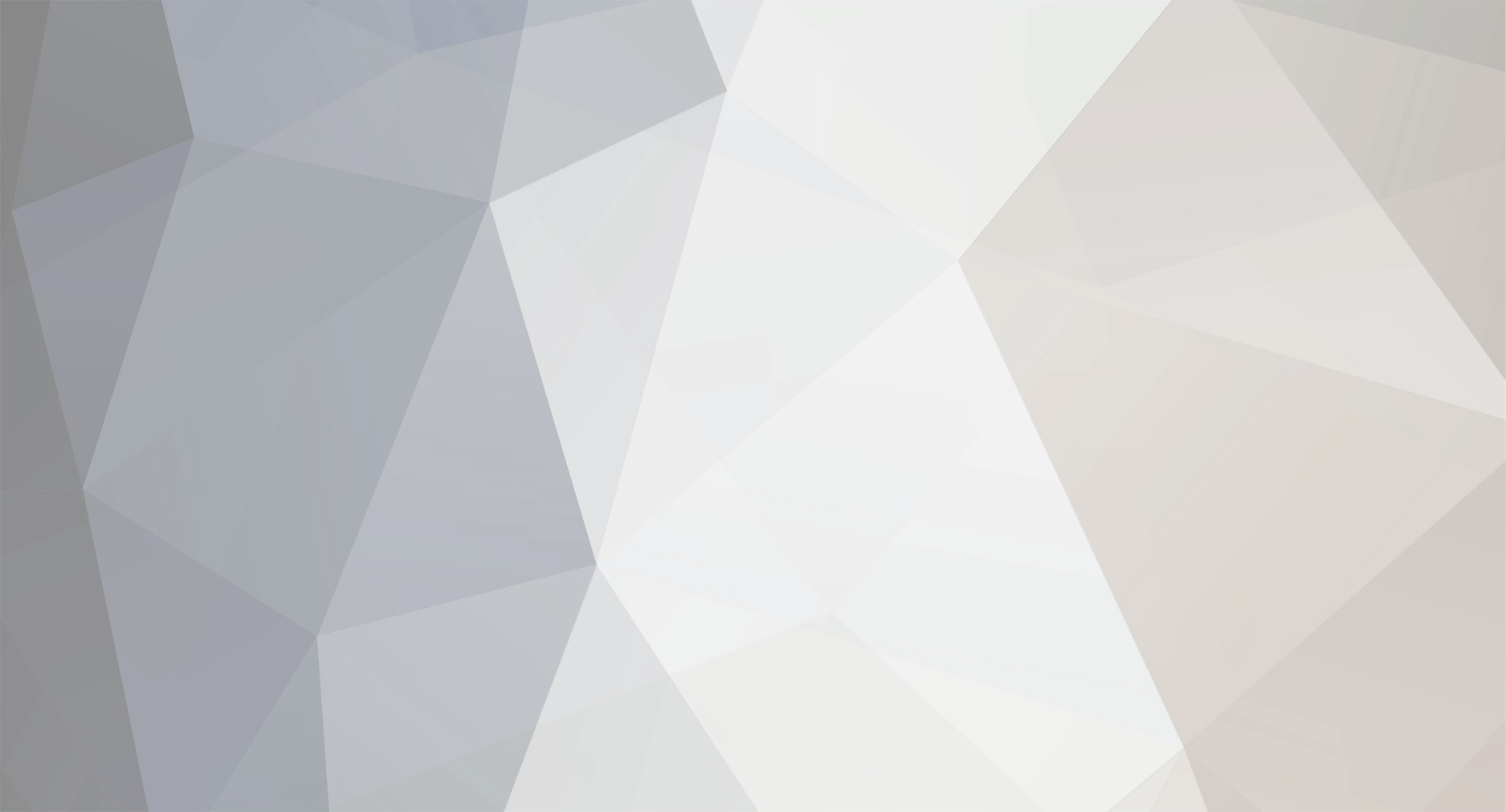
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
In 1.7, you can no longer close GUIs directly from the KeyBinding class, you have to do it in the GUI itself: @Override protected void keyTyped(char c, int keyCode) { super.keyTyped(c, keyCode); // 1 is the Esc key, and we made our keybinding array public and static so we can access it here if (c == 1 || keyCode == KeyHandler.keys[KeyHandler.CUSTOM_INV].getKeyCode()) { mc.thePlayer.closeScreen(); } }
-
Swap tools for an item when reaching 1 durability - Need Help.
coolAlias replied to Deadlyapples's topic in Modder Support
Alright, well first of all you can't store variables directly in your Item class and expect them to function normally, since Items are all declared as static. // No. boolean hitEntityTrue; boolean brokeBlockTrue; boolean itemIsDestroyed; Second of all, if you are putting println's in your methods and never seeing any console output in your Eclipse log (it's not a chat message), then your methods are incorrect or something else is going wrong. Add @Override above all inherited methods to make sure your method signatures are correct. Finally, storing ANY data about the ItemStack is fruitless; you need to get the data directly from the ItemStack EVERY single time a method is called with an ItemStack parameter. I gave you a perfectly valid example earlier and you completely butchered it... just follow the example as closely as possible and only change what is absolutely necessary - meaning only the names of the Items involved. -
Swap tools for an item when reaching 1 durability - Need Help.
coolAlias replied to Deadlyapples's topic in Modder Support
How about you post your item code and we'll take a peek? -
Good on you for trying to do it yourself. I cheated and copied the code from this tutorial. I'm sure you can find some answers in there, though having not built one myself I can't help directly. Good luck!
-
Note that doing so will make your mod incompatible with any other mod that attempts to modify the player's rendering. It is much better for compatibility's sake if you can manage to do what you want using the Forge RenderPlayerEvent hooks. I imagine it would be basically the same, using the post player render event to hook in at the end of rendering to modify the player's arm rotation like GoblinBob mentioned above.
-
Swap tools for an item when reaching 1 durability - Need Help.
coolAlias replied to Deadlyapples's topic in Modder Support
If it's your own Item class, you don't even need to fuss with Events, though that would work, too. It's much more efficient, however, to simply do it in your Item's hitEntity and onBlockDestroyed methods, since those only get called for your Item rather than every single Item that gets destroyed. You can see how I did it for some swords here. Just look for those two methods. If you're coding in 1.7 it's practically exactly the same, but onBlockDestroyed takes a Block instead of int {blockID} as the 3rd parameter. -
I'm just going to refer you to Lex's tutorials on setting up Forge - you no longer need an actual forge folder, set everything up in the directory that you will be using for the mod:
-
Run "gradlew setupDecompWorkspace" and you will get decompiled Minecraft code that you can view; it's in the "referenced libraries" section of your package explorer under "forgeSrc-{version}"
-
I thought that the big [CLIENT] in your title meant you were having troubles with the client side of things, not that you were making a client-side only mod. It's a bit ambiguous, see? The question is still there: why are you trying to render a player other than the one that is currently being rendered? Or is that not what you meant when you said "It seems that the client doesn't know if the other player on the server is on ground or not." ? By "other player" are you just referring to the server-side doppleganger of the client-side player, or actually to another player? Anyway, TGG is spot on, as usual - onGround isn't updated on the client, so you have to do it yourself. You could have just sent a packet from the server to the client with that information, but you are making a client-only mod so you will just have to do the position / block intersection calculations on the client.
-
You can check out my tutorial on custom projectiles, which I adapted and updated from the original Blaster Rifle tutorial. There is some stuff from the original tutorial that I don't cover which you may still find interesting and pertinent to your situation, but mine is up to date and will at least get your projectile all sorted out and rendering correctly.
-
(1.7.2) How many ticks does the world get each second?
coolAlias replied to TLHPoE's topic in Modder Support
Probably once on the client and once on the server: 40 / 2 = 20. -
[1.7.2] How to make a player invisible to mobs?
coolAlias replied to SackCastellon's topic in Modder Support
You could subscribe to LivingAttackEvent as well, checking if the player is invisible and getting the source of the source of damage (yes, you read that right), checking if that is an EntityLiving and then setting their current target to null. Obviously that won't work for everything - creepers, for instance - but it should cover most. Otherwise, you could get all of the living entities nearby the player when they turn invisible and set their targets to null at that time, if their current target is the player turning invisible. Probably simpler to just deal with some mobs still attacking. -
Even if you are trying to animate a player, only do so for the player that is currently available from the client side and then send an animation packet to all nearby clients to update them with the information about the player needing animating. Btw, if you just want to know if the player is in the air, try player.motionY != 0. Your best bet is to do all this stuff client side anyway, since it is just animating. I think you are overcomplicating it when you say you are trying to animate another player - you should never have to do that.
-
onGround should be in EntityPlayer, not the model class... I don't know of any way that you can access another player's client-side information (e.g. the other player's model's data) from another client. Your best bet is probably just to check if the other player's posX, Y - 1, and Z are a solid block (on the server), and then send a packet if you need to know on the client, but why you need to know if another player is on the ground or not to render yourself escapes me... perhaps I'm just not understanding what you mean.
-
Do you encounter this same problem if you create a new world?
-
One set of Armor overwrites the rest...
coolAlias replied to Bobblacksmith's topic in Modder Support
Is there any reason that you have a separate class for every single piece of armor in every single armor set? If you look at vanilla code or even Millenium3's bit of example code there, you can see that you can have all of your armors and pieces using the exact same class and just change the material... As for your IDs... well, you are certainly creating them, though why you would do it that way is beyond me since you make them all final there is no point; anyway, you are using them when you call your armor constructors, but something is happening in there that is not passing those IDs back to the original Item super constructor. Show us one of your many armor classes, if you wouldn't mind. -
One set of Armor overwrites the rest...
coolAlias replied to Bobblacksmith's topic in Modder Support
Your Item IDs are conflicting with vanilla items, so however you think you are getting the starting ID at 16000 obviously is not working. -
You could do that, but it's much cleaner (in my opinion) to take care of that inside of your projectile entity class, much like EntityThrowable etc. do. It's more difficult since EntityArrow's code is a mess, but if you break everything up into manageable pieces in your sub-class, you can avoid EntityArrow's onUpdate entirely and make your custom arrows extremely versatile. Check it out.
-
[1.7.2] How to make a player invisible to mobs?
coolAlias replied to SackCastellon's topic in Modder Support
What TGG said, though if you check properly the second post to the set living attack target event should process just fine. Btw, checking instanceof automatically null checks for you: if (event.target instanceof EntityPlayer) { ((EntityLiving) event.entity).setAttackTarget(null); } That will make the mobs mostly just stand around doing nothing, even if you attack them. If you want them to fight back, you should add the following check to the check for instanceof EntityPlayer: && event.entityLiving.func_94060_bK() != event.target) Note also that this only works on mobs that are using the AI system - spiders, for example, will still attack you. -
[1.7.2] Reference external library in MCP
coolAlias replied to apemanzilla's topic in Modder Support
MCP? Or Gradle? Are you not using Forge? If you are using Forge + Gradle, you need to specify them in your build.gradle file: dependencies { compile files ( "filePathRelativeToWorkingDirectory/completeNameOfReferencedLibrary.jar", "eclipse/mods/1.7.2-SomeReferencedMod-1.0.jar" // <-- example ) } Otherwise I have no idea :\ -
[1.7.2]Multi-Item only showing one in creative tab
coolAlias replied to The_Fireplace's topic in Modder Support
<cough> @Override <cough>