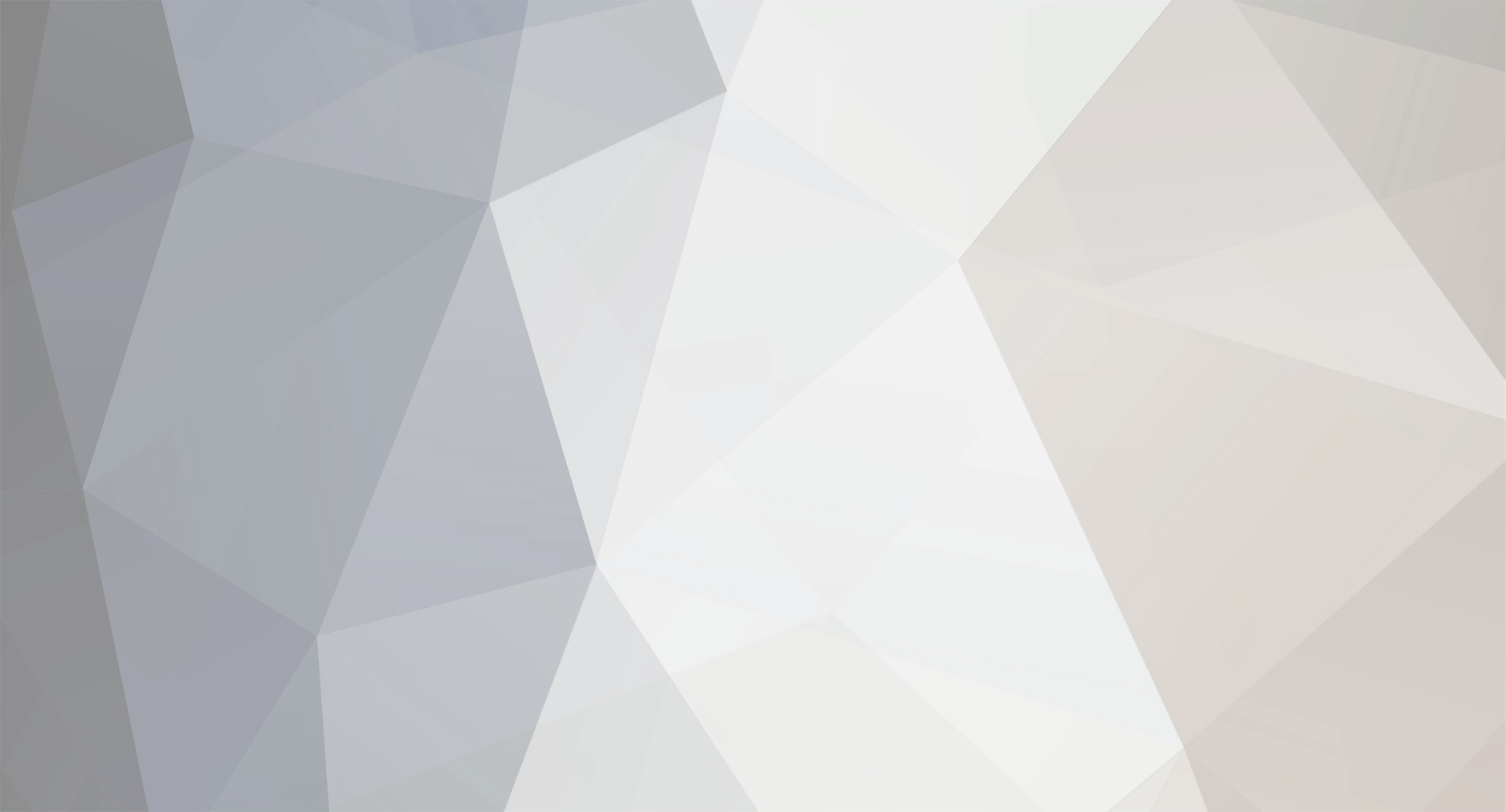
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
Odd, I've never had any problems initializing the mod instance like that. Surely your error was caused by something else, but at least it's solved. I wasn't aware that we're not supposed to initialize them, as I have been since I started modding with no ill effects, but if it's just doing the same work twice, well, no need for that
-
You could just extend ItemFood to take advantage of the automated handling when the item use count reaches the max use duration... otherwise you will have to code it yourself probably using the Item#onUpdate method.
-
[1.7][SOLVED]Trouble getting block names to work.
coolAlias replied to robustus's topic in Modder Support
Check that your run configuration is set to use the correct working directory; if you followed Lex's advice on setting up your workspace, you would point it to the "${workspace_loc}\run" folder, otherwise it would probably be "${workspace_loc\eclipse}" or something like that. Another thing that I've found is quirky in Eclipse, whenever I add or change resources (languages, sounds, textures, etc.), I often have to right-click the resources folder and select "Refresh" for them to show up in the game. -
You need to define the behavior in onPlayerStoppedUsing.
-
[1.7.2] world.destroyBlock not working [Solved]
coolAlias replied to thomassu's topic in Modder Support
It's not nearly that difficult or different. I was under the same impression going in, but it's really quite a simple changeover and most of the stuff is exactly the same, perhaps with a new name (Icon -> IIcon, etc.). Depending on how complicated your mod is, it will probably only take a couple hours to completely convert it and work out most of the bugs, since 1.7.2 tends to act differently in certain situations. For example, in my mod I rely quite heavily on key pressed mechanics for activating my skills, and some of that didn't work doing a straight port to 1.7, but pretty much everything else did. Anyway, give it a go and see for yourself -
[1.7.2] world.destroyBlock not working [Solved]
coolAlias replied to thomassu's topic in Modder Support
Honestly, the majority of methods and fields that are commonly used are already deobfuscated, with the occasional random exception such as destroyBlock. But with a little looking, it's usually not too difficult to find what you need. -
Netty Packet Handling. If you'd like to see a basic example of it in use, you can check my 1.7.2 tutorial code.
-
The Minecraft version and type of Block are irrelevant. You need to create a custom ItemBlock (the block that is actually in your inventory is an ItemBlock, not a Block) and set the max stack size to 1 in the constructor.
-
diesieben07 already covered the most important ways that timing is handled in Minecraft. If you want the player's view to rotate smoothly, you should probably implement a tick handler for RenderTicks (RenderTickEvent in 1.7). From there, you can simply adjust the player's rotation yaw by some increment each tick while your command or whatever is active.
-
There is no processing of slots on the gui image that you or I need to worry about; it's done automatically by the GuiContainer / Container. The coordinates for the slots are where the slots appear, which is where the items render. Just try it and see if it works. If not, we can try something else.
-
[1.7.2] world.destroyBlock not working [Solved]
coolAlias replied to thomassu's topic in Modder Support
It is there, it's just not deobfuscated yet: World#func_147480_a(int x, int y, int z, boolean drops) is destroyBlock. -
There shouldn't be any packets involved at all, at least not that you need to worry about. IInventory-Container-GuiContainer are a slick package that handle everything you need internally, for the most part. Have you tried using the standard gui positions for the player inventory? Your slot positions are way off, probably off the screen: // Main, yours: 133 + k * 18, 122 + j * 18 // Main, mine: 8 + k * 18, 84 + j * 18 // Action bar, yours: 133 + j * 18, 179 // Action bar, mine: 8 + j * 18, 142
-
Part of your problem is that the slot indices you are using for your chest inventory are messed up; they should start at zero and increment by 1 each iteration, just like the vanilla player inventory. Take a look: for (int chestRow = 0; chestRow < 2; chestRow++) { // iterates from 0 to 1, we'll label this 'a' for (int chestCol = 0; chestCol < 3; chestCol++) { // iterates from 0 to 2, we'll label this 'b' // values of your indices (b + a * 2) for a = 0, b = (0 to 2) are 0, 1, 2 // values of your indices (b + a * 2) for a = 1, b = (0 to 2) are 2, 3, 4 addSlotToContainer(new Slot(chestInventory, chestCol + chestRow * 2, 12 + chestCol * 18, 8 + chestRow * 18)); } } for (int y = 0; y < rows; y++) { for (int x = 0; x < cols; x++) { // these start at 9 (0 + 1 * 9), go up to 17 (8 + 1 * 9), move to the next row at 18 (0 + 2 * 9), etc. // meaning the player inventory slots are indices 9-35 (inclusive, so 27 slots) addSlotToContainer(new Slot(playerInventory, x + (y + 1) * cols, 133 + x * 18, 122 + y * 18)); } } // why does it start at 9? the action bar takes slots 0-8: for (int x = 0; x < cols; x++) { addSlotToContainer(new Slot(playerInventory, x, 133 + x * 18, 179)); } EDIT: Furthermore, looking at your TileEntity, you never use markDirty() when the inventory changes. I use it in the following two methods for my TileEntity-based inventories: @Override public ItemStack decrStackSize(int slot, int amount) { ItemStack stack = getStackInSlot(slot); if (stack != null) { if(stack.stackSize > amount) { stack = stack.splitStack(amount); markDirty(); } else { setInventorySlotContents(slot, null); } } return stack; } @Override public void setInventorySlotContents(int slot, ItemStack itemstack) { inventory[slot] = itemstack; if (itemstack != null && itemstack.stackSize > getInventoryStackLimit()) { itemstack.stackSize = getInventoryStackLimit(); } markDirty(); }
-
ALL events MUST be "public void", NOT static, not private. Look at yours: public static void onPlayerLogin(PlayerEvent.PlayerLoggedInEvent event) {}
-
[1.6.2] Custom armor doesn't renders correctly
coolAlias replied to SackCastellon's topic in Modder Support
In getArmorTexture, slot 2 is for legs, all other slots are for the other armor pieces, but you are only checking for slot 0 or 1, then passing 2 and 3 off in the else statement. 3 is for your boots, mate, getting lumped in with the leggings overlay. Btw, you misspelled leggings as "leggins". -
By doesn't work anymore, surely you must mean never worked. Look at the import for your PlayerLoggedInEvent, and you will see it's in the cpw.mods.fml.common.eventhandler.Event package, NOT MinecraftForge. You need to register it to the FML bus: FMLCommonHandler.instance().bus().register(new YourFMLEventHandler());
-
Sorry, I still haven't been able to find out why the ModAPITransformer isn't stripping the interface when loading my built mod in the normal Minecraft launcher. Am I just using it incorrectly, or is it not working properly? In the meantime, I'm back to using two classes that are identical except that one implements the other mod's api interface and is only used when that mod is loaded, while the other gets used otherwise. While that works just fine, I'd prefer a more sophisticated approach. If anyone can point me in the right direction, I'd be very grateful.
-
That's because you are using the Minecraft display height and width directly; you need to create and use a Scaled Resolution: ScaledResolution resolution = new ScaledResolution(mc.gameSettings, mc.displayWidth, mc.displayHeight); resolution.getScaledWidth() // there is also a double version, if needed resolution.getScaledHeight() // there is also a double version, if needed
-
Determining whether or not the player has the chat window open
coolAlias replied to Athire's topic in Modder Support
This should work for all Minecraft versions: if (Minecraft.getMinecraft().currentScreen == null) { } -
You can start by creating an item that stores an inventory. Then you can subscribe to the Forge ItemPickupEvent, check if your player has your backpack item, and store the item in there instead of the regular inventory. For crafting, you'd probably have to implement that in the Item class onUpdate method for your Backpack Item, checking its inventory every now and again to see if there is anything it can craft.
-
ChestGenHooks is for loot, DungeonHooks is for mobs (though it used to be for loot, so is deprecated in that sense)
-
The Item class has two built-in tick handlers, one for all items and one specific to armor. Look for the method onArmorTick (in 1.6.x it was called onArmorTickUpdate). It's a much better solution than using the TickEvents in this case.
-
That sounds like the packet data that you are reading is larger than the packet data you wrote, or maybe the other way around, though I could be completely wrong. Why not show us your encodeInto and decodeInto code?