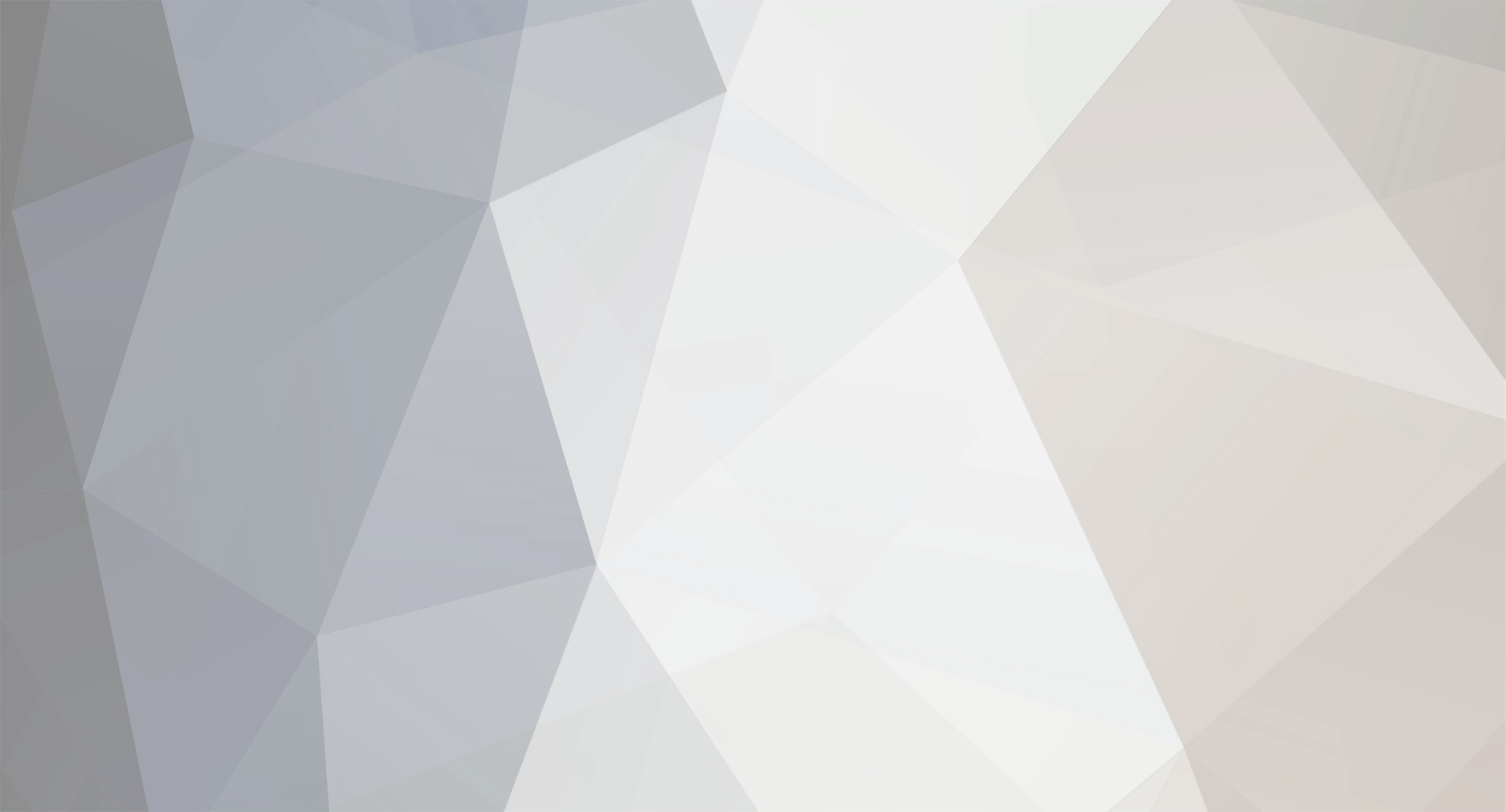
rich1051414
Forge Modder-
Posts
160 -
Joined
-
Last visited
Everything posted by rich1051414
-
How to make a block with 32*32 in its Texture?
rich1051414 replied to Wanghh's topic in General Discussion
You don't choose that, it depends on the texture file being used. Texture files have 256(16x16, not size, actually 16 tiles, by 16 tiles.) tiles, the size of which depends on the size of the actual texture file that contains it. A 256x256 texture file holds 16pixelx16pixel tiles. 512x512 holds 32pixelx32pixel tiles, and so on. -
Different block face textures while using infinite terrain
rich1051414 replied to HuskyBlueFire's topic in Modder Support
This is actually really simple, don't get discouaged. Texture files have mini texture tiles of 16 rows of 16. if you start numbering them from the top left to the bottom right, these are the numbers that are expected to be returned. The code depends on the way you set up your texture file, and which side needs which texture, but in the method: getTextureFromSideAndMetadata(int i, int j) the two variables are, as expected side and metadata, in that order. From this info, you can figure out which texture index you need to return. The actual texture file is retrieved by the renderer by your getTextureFile() method you need to override. -
Well, without using shaders(advanced, not all cards support it), you would increase the stack and slice count, but spheres are incredibly intensive. 'sphere.draw(0.5F, 32, 32);' the last 2 control the detail, first, how many slices, and the other is stacks. Basically, vertical and horizontal quality. As quality of the sphere increases, performance decreases non-linearly. Basically meaning after a certain point, the quality gain is not worth the cost(which is why modern cards now have tesselation acceleration, so they can get high quality rounded and high detail objects). You could play with the stack and slice count to find what you like best. By increasing the slice to stack ratio, the sphere sports a grid shading as opposed to what you see in the picture TroubleDad posted. Like this: sphere.draw(0.5F, 48, 24); That would give you similar performance, but the shading might be more attractive to you. Also, using GLU_FLAT instead of GLU_SMOOTH not only can increase performance in spheres, it might give it a better appearance, and hide the sharp edges. You can't simply remove 1 side of a cube and open it up, you have to connect it, so you have to do this: _ _ || || || || ||______|| -------------- Also, you cold draw a sphere within a sphere, by making the larger sphere transparent, and the inner sphere have a smaller radius. sphere.draw(0.5F, 128,64); with sphere.setNormals(GLU.GLU_FLAT); gives me a near perfect sphere, and even with 20 of them in view, I cannot see any noticeable fps drop on my modern system(but this will vary greatly on older systems). Here is an Image https://dl.dropbox.com/u/74770478/2012-12-09_18.04.24.png[/img]
-
I don't know what that code is suppose to be doing, but 'i' tells you the meta, and 'j' tells you which face you need to return. You could do something like this: //Where does this blocks textures start int OffSet = 0; return OffSet + (i * 6) + j; That could work if there is a different texture for every side and meta possibility, in order. Otherwise you will need some logic to determine the situational texture to use.
-
If your mod is simply a texture pack mixer, and the user is required to go to the makers website, this would violate no eula, as your content is strictly your own, which is the texture pack combining. As long as you claim no credit for the texture packs themselves, and do not distribute the texture packs themselves, this is completely legal, and I feel is also ethical. Application would be rather easy as well, java has everything you need in the built in libraries, so basically all you would need to do is create a file format to store the texture pack combination information. Minecraft has a GZIP library built in so you can piece everything together rather easily. I really like this idea, I appreciate people finding clever solutions to make everyone happy, even if it is not something I actually desire personally.
-
This is how you do it, btw, the image will be upside down, but that's easy to fix in any image viewing software. Oh, and do this after the world is loaded with the sun up for the best lighting. When I did this, I bound it to a key, the screen will flash. You will probably have to adjust the rotation, this one is most probably wrong, this was off the top of my head glPushAttrib(GL_ALL_ATTRIB_BITS); glPushMatrix(); glClearColor(0.0f, 0.0f, 0.0f, 0.0f); glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT); IntBuffer glFrameBuffer = BufferUtils.createByteBuffer(Minecraft.getMinecraft().displayWidth * Minecraft.getMinecraft().displayHeight * 4).asIntBuffer(); BufferedImage bufferedImage = new BufferedImage(Minecraft.getMinecraft().displayWidth, Minecraft.getMinecraft().displayHeight, BufferedImage.TYPE_INT_ARGB); glEnable(GL_LIGHTING); glTranslatef(220f, 150f, 0f); glScalef(100f, 100f, 100f); glRotatef(90, 0.0F, 1.0F, -1.0F); ForgeHooksClient.bindTexture(Block.stone.getTextureFile(), 0); Minecraft.getMinecraft().renderGlobal.globalRenderBlocks.useInventoryTint = true; Minecraft.getMinecraft().renderGlobal.globalRenderBlocks.renderBlockAsItem(Block.stone, Color.WHITE.getRGB(), 1.5F); glPixelStorei(GL_PACK_ALIGNMENT, 1); glPixelStorei(GL_UNPACK_ALIGNMENT, 1); glReadPixels(0, 0, Minecraft.getMinecraft().displayWidth, Minecraft.getMinecraft().displayHeight, GL12.GL_BGRA, GL12.GL_UNSIGNED_INT_8_8_8_8_REV, glFrameBuffer); int array[] = new int[Minecraft.getMinecraft().displayWidth * Minecraft.getMinecraft().displayHeight]; glFrameBuffer.get(array); bufferedImage.setRGB(0, 0, Minecraft.getMinecraft().displayWidth, Minecraft.getMinecraft().displayHeight, array, 0, Minecraft.getMinecraft().displayWidth); File file = new File("C:/BlockPics"); file.mkdirs(); file = new File("C:/BlockPics/" + Block.stone.getBlockName() + ".png"); try { file.createNewFile(); ImageIO.write(bufferedImage, "png", file); } catch (IOException ex) { FMLLog.getLogger().log(Level.SEVERE, "Error Saving File!", ex); } glPopMatrix(); glPopAttrib();
-
No, that is preventing the mod from loading. The issue is you did not create a new instance of your block before registering it.
-
I set the texture to a 1x1 White Texture so I can set the color before calling it, and it works brilliantly. I did notice however, how damn out of place spheres are in minecraft. I'd like to see it sounds cool Very well. It is not a mod, I as just playing with the idea, but this is how it looks. I made it so it generates a random color when placed so I can get a large color variation without much effort. Here is the screenshot. that is to cool do you mind if i do things with this to? I Posted the code as a tutorial, do what you want.
-
Looking good. Are you going to do anything with it? I didn't realize giving people this information would get as much attention, I guess it is something people have actually thought about. One of you should code some rudimentary physics, and make a soccer ball / bouncy ball
-
Done Downloading Error (Not meta-inf)
rich1051414 replied to The_Snowman's topic in Support & Bug Reports
There is no universal for 1.2.5, that started in 1.3.2 when the client and server combined. This is the version you need. -
Done Downloading Error (Not meta-inf)
rich1051414 replied to The_Snowman's topic in Support & Bug Reports
Universal does NOT mean any version, it means server AND client. go Here for 1.2.5 -
instead of setting the damage to 0, set the ItemStack to null.
-
How can I make the machine still working in a long distance?
rich1051414 replied to Wanghh's topic in General Discussion
Chunk loaders is how it is traditionally done. This is normal behavior of minecraft, and the reason chunk loaders were created. If that is too much for what you need to do, the only other option is to map your tile entities(coordinate based key) and tick them all on a server tick(burn time value), and have the tile entities check that map for it's burn time. This would only keep the burn time ticking, but would have no impact on anything else however. Also the map would need to be saved or burn time would be lost on every reload. Chunk loading would actually be an easier to implement way of doing this, but the way described above would also work without sacrificing as much performance as chunk loading does if you only want it to keep 'cooking'. Edit: An easier way would be to make the burntime tick counter realtime aware. You could have it's burntime be based on realtime instead of ticks. You could set a start time, and it's current burn percent is based on the current time. -
Here, I will help you. I tried it out and I see what your problem was, you weren't seeing it because you hadn't set up yet(Probably it was drawing on a transparent section of the currently bound texture). I noticed when rendering spheres that performance dropped a lot, but I found instancing the spheres instead of redrawing them at every render call helped immensely, this is how: First have your proxies both have a method like this: public int sphereID() {} The common should just return 0, but in your Client have it return a static varible named something like public static int sphereID; Now, at the bottom of your Clients RenderingRegistry, add this(This is the important bit): //GLU.Sphere Sphere sphere = new Sphere(); //GLU_POINT will render it as dots. //GLU_LINE will render as wireframe //GLU_SILHOUETTE will render as ?shadowed? wireframe //GLU_FILL as a solid. sphere.setDrawStyle(GLU.GLU_FILL); //GLU_SMOOTH will try to smoothly apply lighting //GLU_FLAT will have a solid brightness per face, and will not shade. //GLU_NONE will be completely solid, and probably will have no depth to it's appearance. sphere.setNormals(GLU.GLU_SMOOTH); //GLU_INSIDE will render as if you are inside the sphere, making it appear inside out.(Similar to how ender portals are rendered) sphere.setOrientation(GLU.GLU_OUTSIDE); //Simple 1x1 red texture to serve as the spheres skin, the only pixel in this image is red. BufferedImage bi = new BufferedImage(1, 1, BufferedImage.TYPE_INT_ARGB_PRE); bi.setRGB(0, 0, Color.red.getRGB()); //Bind our texture to a string. ForgeHooksClient.textures.put("1x1RED", Minecraft.getMinecraft().renderEngine.allocateAndSetupTexture(bi)); //sphereID is returned from our sphereID() method sphereID = GL11.glGenLists(1); //Create a new list to hold our sphere data. GL11.glNewList(sphereID, GL11.GL_COMPILE); //Offset the sphere by it's radius so it will be centered GL11.glTranslatef((float) 0.50F, (float) 0.50F, (float) 0.50F); //Call our string that we mapped to our texture ForgeHooksClient.bindTexture("1x1RED", 0); //The drawing the sphere is automattically doing is getting added to our list. Careful, the last 2 variables //control the detail, but have a massive impact on performance. 32x32 is a good balance on my machine. sphere.draw(0.5F, 32, 32); //Drawing done, unbind our texture ForgeHooksClient.unbindTexture(); //Tell LWJGL that we are done creating our list. GL11.glEndList(); Now, in our rending class, when you need to render the sphere, do this: GL11.glPushMatrix(); //Default parameters in TileEntitySpecialRenderer's renderTileEntityAt GL11.glTranslatef((float) var2, (float) var4, (float) var6); GL11.glCallList(YourModClass.proxy.sphereID()); GL11.glPopMatrix();
-
Thanks for your reply! If you mean doing it like Mojang does it with the Dragon-Egg, by just adding many small boxes in a roundish shape, no. I mean making a perfect sphere without any edges Well to use lwjgl's built in modeling helpers, look into GLU, more specifically quadrics. It includes sphere building methods. To draw a circle, just do a bit of math: for (int t = 0; t < 2 Pi; t+= stepsize) GL11.glVertex2d(Math.sin(t),Math.cos(t));
-
I believe you need to return false from overriding renderAsSolidCube or isSolidBlock something like that >isBlockSolidOnSide<. This tells the renderer you can see through it.
-
Ok I have encountered a bit of problem that is leaving me scratching my head a bit. I am rendering a living entity onto the HUD, and I have it working great except for one small problem. If there is an image on the same X as my rendered object, it becomes affected by lighting, and if the sky is the backdrop, the sky changes colors slightly. I have tried disabling all the common features after my render, but nothing seems to be fixing the issue.(Yes, I did try doing a GL11.glDisable(GL11.GL_LIGHTING), this does not work, it still causes images to be effected by lighting. I will point out that I am using the entities current renderer to do my rendering, I just translate, rotate and scale it into place, and I have been setting up my environment the normal way with entityRenderer.setupOverlayRendering(Although it makes no difference it seems whether I do this or not). Here is my relavent rendering code: RenderLiving ren = (RenderLiving) RenderManager.instance.getEntityClassRenderObject(el.getClass()); GL11.glTranslatef(locX, locY, 0); GL11.glRotatef(180, 0, 0, 1); GL11.glScaled(25, 25, 25); ren.doRender(el, 1, 1, 1, 0, 0); Anyone got a clue? Edit: I solved it with a GL11.glPushAttrib(GL11.GL_ALL_ATTRIB_BITS); So it's all good now (That pushes the current settings stack, so when you pop the stack, they are restored)
-
No, research Tile entities. This is all you need. No need to be rude about it, especially when you had no input on it in the first place. Who was being rude? I was trying to help you by putting you in the right direction. If you research what tile entities are, you will see it is exactly what you need.
-
How will the burntime transfer from server to client?
rich1051414 replied to Wanghh's topic in General Discussion
Datawatchers. Look into datawatchers, and everytime the burn time changes(server side) update the datawatcher for those who are listening(the clients) I assume he uses a block as furnace with a TileEntity. There isn't a DataWatcher available. What you can do is the build-in methods for sending/receiving packets in the TileEntity. Just look at the vanilla TileEntity class for the methods onDataPacket and getDescriptionPacket and override them in your TileEntity class. Here's an example code: @Override public void onDataPacket(INetworkManager net, Packet132TileEntityData pkt) { this.burnTime = par1Compound.getInteger("BurnTime"); } @Override public Packet getDescriptionPacket() { NBTTagCompound nbt = new NBTTagCompound(); nbt.setInteger("BurnTime", this.burnTime); return new Packet132TileEntityData(this.xCoord, this.yCoord, this.zCoord, 0, nbt); } Yes you are right. My bad, I have been doing too much work with entities lately and not enough tile entities. San Andreas is correct And that is the same method I use for various information storage related blocks I made that he showed above. -
How will the burntime transfer from server to client?
rich1051414 replied to Wanghh's topic in General Discussion
Datawatchers. Look into datawatchers, and everytime the burn time changes(server side) update the datawatcher for those who are listening(the clients) -
No, research Tile entities. This is all you need.
-
Blocks are assigned a specific ID apon creation when the game starts, and many mappings rely on the first mapping being currect and never changing for the game to function. You would have to unassign all mod blocks, and reassign them with new configs, but these actions happen upon loading of the mod, at startup. What one could do is design a system to stream the configs from the server upon connection if it fails a blockID check, and then force a restart when synced, but this would require quite the deep mod. I would support a function like this to be added to forge, but it might not happen because such a feature may cause undesired consequences, like breaking players single player maps without really realizing it(since the process was made so easy).
-
Server Admin Option for Turning off Chunkloader for offline users
rich1051414 replied to darkthought's topic in Suggestions
This still is not qualifying for a forge feature, but possibly a dedicated mod feature. One could do this in a mod quite easily, when a player places a chunkloader, map the player to those coordinates, when he logs out, replace it with a unremovable(except by ops) sign with the user and chunkloader that would have been there, and replace it when the user logs back in. Would not be very difficult to accomplish, and would not be hard for the author to keep it updated with the current various chunk loaders(or add in a config for the admins to add to). If the chunkloader has a null player who placed it(like some kind of deployer) then the mod could simply break the chunk loader. -
Well mods could disable an ore that is already added to the ore dictionary, and then use that block for use in ore generation instead of their own, this could help bring everything together, but everyone would have to cooperate to make that happen.
-
block id issues while building .minecraft folder
rich1051414 replied to infinus5's topic in General Discussion
Block ID's cannot surpass the ID of 4095. Usually Item ID's are assigned beyond 4096 and as high as 31999. If you cannot for the life of you get everything behaving, you could use ID Resolver, but be warned, this could break mods that do not address the instance's blockID's and instead use the block and Item ID's the config returned. It can also make server play extremely annoying, as well as breaking your maps everytime you add or remove a mod.