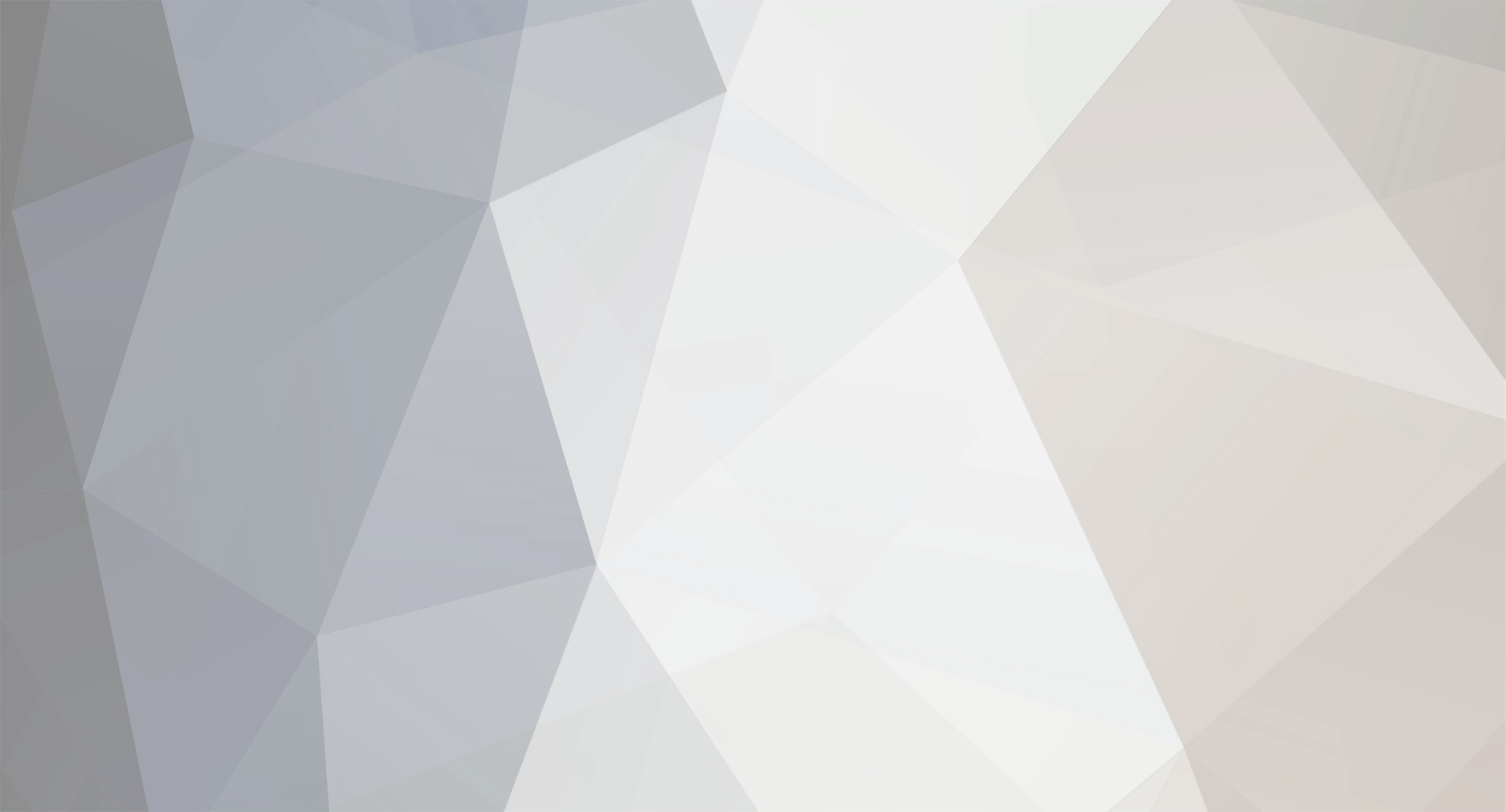
gcewing
Forge Modder-
Posts
145 -
Joined
-
Last visited
Everything posted by gcewing
-
You may also want to look into implementing ISidedInventory. This will allow Buildcraft pipes, Redpower tubes, etc. to access different slots depending on which side they connect to.
-
As often as you need to keep the two sides sufficiently synchronised for your purposes. The vanilla furnace sends progress updates every tick while the furnace is burning. However, it cheats a bit and only does this while a GUI is open. It gets away with that because the only reason the client needs to know about the internal state of the furnace is for displaying the GUI. If it's the same for your machine, you can do likewise. If you need to keep the sides synchronised at other times, you may want to be more selective, and send updates only for important events that the client needs to know about, such as when it finishes working on an item. The details will depend on your machine.
-
Depending on how the block is implemented, this may be difficult or impossible. With some crafting blocks, such as the vanilla crafting table, the crafting is actually done by the GUI. The block itself doesn't even have an inventory (that's why any items in the grid fall on the ground when you close it). You might be able to fake things by creating a Container of the appropriate type and driving it as though a player were interacting with it. The code would need to be specific to that one type of crafting block, though. I don't think there's any general way to do this.
-
Help with syncing client and server [unsolved]
gcewing replied to Floppygunk's topic in General Discussion
After changing the tile entity's state, you need to call the block's markBlockForUpdate method -- this ensures that the state is sent to the client and the block is re-rendered. You should also call the tile entity's OnInventoryChanged method, otherwise the state might not get saved to disk. -
You need to add dx and dz to the player's current position, not just set them. However, that probably isn't the right thing to do either, as it will attempt to teleport the player to the new position, regardless of whether there are any obstacles in the way, and also regardless of whether there is an empty space at the new position. If you teleport a player into a solid mass of blocks, he will get stuck and suffocate. To move the player while taking collisions into account, use the moveEntity method: player.moveEntity(dx, 0, dz); If you really want to teleport the player, the right way is to call setPositionAndUpdate: player.setPositionAndUpdate(player.posX + dx, player.posY, player.posZ + dz); This will ensure that the new position is communicated correctly to the client side.
-
[Solved] Updating TileEntitySpecialRenderer
gcewing replied to GiannivanMarion's topic in Modder Support
You need to call markBlockForUpdate for the block on the server side whenever the tile entity changes in a way that the client needs to know about. You also need to call OnInventoryChanged of the tile entity to ensure that the changed state gets saved to disk. -
You should study the furnace and crafting table to see how they do things. Essentially you'll need a TileEntity with an inventory having a slot for each input and one for the output, and an associated Container and GuiScreen.
-
You need a constructor with this signature: EntityZombieSpawner(World world) I think what happens is that the server sends a packet to the client telling it to spawn its own local copy of the entity, and the client tries to instantiate it by calling the above constructor. The client doesn't need to know about the throwing entity, so it should be all right to leave it null in that case, i.e. public EntityZombieSpawner(World world) { super(world); }
-
I don't think so. What are you trying to do? Maybe there's another way to approach the problem.
-
I don't usually say anything about my projects until I'm ready to release something, but I can't resist showing a bit of what I've got so far. Stargates, SG-1 style:
-
How to programm a custom bucket with Minecraft Forge?
gcewing replied to Netglex's topic in Modder Support
You should certainly start by taking a look at ItemBucket and seeing how it does things. Whether you should subclass it or not depends on how much you want to customise it. If all you want to do is make it look like it's made of gold, you probably don't need a subclass. Just make an instance of ItemBucket and use setTextureFile and setIconCoords to give it your own icon. You'll also want to set its name and install a language translation for that name so that it appears as "Gold Bucket" or whatever in tooltips. You'll actually need to create two instances, one for a full bucket and one for an empty bucket, with different item IDs. Look at the two "new ItemBucket" calls in Item.java to see how you need to set them up. -
Setting bounding boxes on a per-block basis...
gcewing replied to voidzm's topic in General Discussion
To clarify, there are two different notions of bounding boxes. One of them is kept in a set of static variables in the Block class, and the game calls the block's setBoundingBoxBasedOnState method immediately before doing anything that uses it. Because it's static, if you don't update it at the right times you get weird interactions between blocks. This bounding box is used for drawing the wireframe around the block you're looking at. The other kind of bounding boxes are used for collision detection with entities; this is what getCollisionBoundingBoxFromPool is for. It's also possible for a block to have multiple collision boxes by overriding addCollidingBlockToList instead and adding more than one block to the list; stairs do this, for example. -
Questions About Mod Design and Compatiblity
gcewing replied to neovista's topic in General Discussion
Including the other mod's API files in your mod is not the best idea, because it means that when the other mod is present, there are two copies of the API files around. If they're identical, that's not a problem, but if the API changes in any way, the presence of outdated API files in your mod can break the other mod. A better way is to isolate everything that references the other mod's API into a separate group of classes that are not referenced directly anywhere from your main code. Then at run time, test for the presence of the other mod, and if it's there, use reflection to dynamically load and instantiate a class that creates and registers all the blocks, items, etc. that use the API. And then ship your jar file without any of the other mod's API files in it. If you do things that way, your mod has a good chance of surviving updates to the other mod's API, as long as the changes consist only of adding new interfaces and methods, and not changing any existing method signatures. If you haven't used reflection before, read up on Class.forName(), getConstructor() and invoke(). -
If I remember rightly, if you do double a = Math.toRadians(player.rotationYaw); double dx = -Math.sin(a); double dz = Math.cos(a); then (dx, dz) is a unit vector pointing in the direction the player is facing in the X-Z plane. Multiply that by the distance you want to move.
-
anyone know how the vanilla grass block works?
gcewing replied to robustus's topic in General Discussion
It'll be in RenderBlocks somewhere. Stock up on aspirin before looking in there though, as it's a tremendously tedious and obscure piece of code... There are two different branches to the rendering code, depending on whether ambient occlusion is enabled (I think this is the "Fancy Lighting" option in the video settings). In renderStandardBlockWithAmbientOcclusion() there are passages of code like this: this.colorRedTopLeft = this.colorRedBottomLeft = this.colorRedBottomRight = this.colorRedTopRight = (var17 ? par5 : 1.0F) * 0.6F; // a bunch more colour-setting code here this.renderNorthFace(par1Block, (double)par2, (double)par3, (double)par4, var27); if (Tessellator.instance.defaultTexture && fancyGrass && var27 == 3 && this.overrideBlockTexture < 0) { this.colorRedTopLeft *= par5; // a bunch more colour-setting code here this.renderNorthFace(par1Block, (double)par2, (double)par3, (double)par4, 38); } which is what appears to be rendering the face a second time with different lighting when a grass block is being rendered (id == 3). The other branch is in renderStandardBlockWithColorMultiplier(): var8.setBrightness(this.customMinZ > 0.0D ? var26 : par1Block.getMixedBrightnessForBlock(this.blockAccess, par2, par3, par4 - 1)); var8.setColorOpaque_F(var18, var21, var24); var28 = par1Block.getBlockTexture(this.blockAccess, par2, par3, par4, 2); this.renderEastFace(par1Block, (double)par2, (double)par3, (double)par4, var28); if (Tessellator.instance.defaultTexture && fancyGrass && var28 == 3 && this.overrideBlockTexture < 0) { var8.setColorOpaque_F(var18 * par5, var21 * par6, var24 * par7); this.renderEastFace(par1Block, (double)par2, (double)par3, (double)par4, 38); } Since all this is hard-coded to recognise the block id of vanilla grass, even if you copy all of the code in BlockGrass into your block class, it won't render the same way. Where to go from here depends on how much you want to customise the appearance of your block. If you want it to look exactly like a vanilla grass block, I think you should be able to get that by installing a custom block renderer that calls renderBlockByRenderType() and passes it the vanilla grass block object instead of your own block. If you want to customise your block more than that, you may need to figure out what that code is doing with the lighting and do something similar in your own block renderer. -
I have a class declared like this: public class BaseBlockRenderer<BLOCK extends BaseBlock<TE>, TE extends TileEntity> implements ISimpleBlockRenderingHandler { BLOCK block; TE te; ... where BaseBlock is declared as public class BaseBlock<TE extends TileEntity> extends BlockContainer ... This works, but it's a bit annoying that you have to specify the tile entity class explicitly when instantiating BaseBlockRenderer. Is there any way to arrange things so that the compiler can infer the TE parameter from the supplied block type? I.e. so that given class MyBlock extends BaseBlock<MyTileEntity> you can just write BaseBlockRenderer<MyBlock> instead of BaseBlockRenderer<MyBlock, MyTileEntity> , and still be able to declare things of type TE inside BaseBlockRenderer. I've tried the following things, none of which work: public class BaseBlockRenderer<BLOCK extends BaseBlock<TE extends TileEntity>> --- syntax error public class BaseBlockRenderer<BLOCK extends BaseBlock<TE>> --- can't find name TE <TE extends TileEntity> public class BaseBlockRenderer<BLOCK extends BaseBlock<TE>> --- syntax error public <TE extends TileEntity> class BaseBlockRenderer<BLOCK extends BaseBlock<TE>> --- syntax error public class <TE extends TileEntity> BaseBlockRenderer<BLOCK extends BaseBlock<TE>> --- syntax error
-
It all depends on Eloraam. As soon as she releases an API for Redpower blutricity, I'll get onto it. No immediate plans, but I'll consider it. As for power, is there an agreed rate of conversion between EU and MJ?
-
Thanks, this makes a lot of sense in terms of improving efficiency. I'll incorporate it into the next version. There does appear to be a bug in IC2, though. It seems like it should work with a smaller buffer, even if it's inefficient. Maybe the IC2 developers should be told about it?
-
Error in My Furnace Recipes dont know how to change to fix
gcewing replied to jamesscape2's topic in General Discussion
You'll need to combine your three ints into a single object to use as a key in your HashMaps. A List of Integer instances should work, I think. -
It seems that world.getWorldInfo().getWorldName() only works on the server side. For the client, it's always set to "MpServer". Does anyone know of a way for the client to find the real name of the world?
-
Sorry about that! I'll try to do something about it in the next release.
-
I'm not getting any such messages. What version of IC2 are you using, exactly? Are there any special circumstances under which it happens?
-
I'd like to change the way mob spawning is done, so that instead of mobs just appearing randomly in the vicinity of the player, they emerge from particular structures found in caves, etc. Similar to the way mob spawners currently work, except that they would have a much bigger activation range. First, does anyone know of an existing mod that does anything like this? Second, any ideas on how to override the existing mob spawning code? I will need to somehow disable the current spawning behaviour for some (but probably not all) of the hostile mobs. Forge doesn't seem to provide much in the way of hooks for this, and I'd really like to avoid modifying base classes if at all possible.
-
Iron tank problem should be fixed now.
-
What kind of structures does this affect, so I can test it?