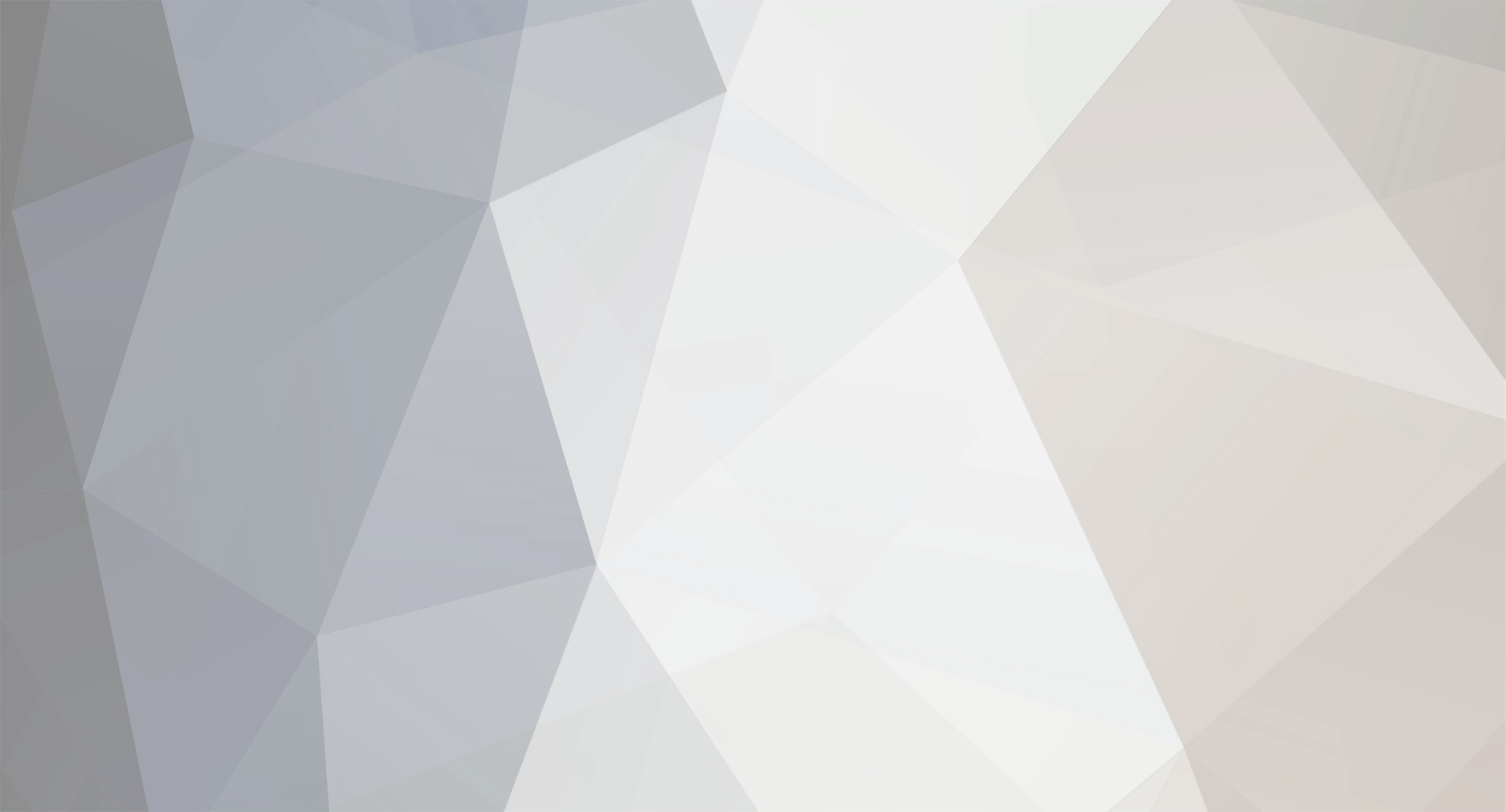
Failender
Forge Modder-
Posts
1091 -
Joined
-
Last visited
Everything posted by Failender
-
[1.8] PlayerEvent.HarvestCheck not fired when mining with tools
Failender replied to Failender's topic in Modder Support
For everyone interested, here's my solution @SubscribeEvent public void canHarvest(HarvestDropsEvent event) { if(event.state.getBlock().getUnlocalizedName().equals("tile.oreIron")) { ItemStack holding = event.harvester.inventory.getStackInSlot(event.harvester.inventory.currentItem); if(holding!=null && holding.getItem() instanceof ItemPickaxe) { if(holding.getItem().getHarvestLevel(holding, "pickaxe") <3) { event.drops.clear(); } } else { event.drops.clear(); } } } @SubscribeEvent public void breakSpeed(BreakSpeed speed) { if(speed.state.getBlock().getUnlocalizedName().equals("tile.oreIron")) { ItemStack holding = speed.entityPlayer.inventory.getStackInSlot(speed.entityPlayer.inventory.currentItem); if(holding!=null && holding.getItem() instanceof ItemPickaxe) { if(holding.getItem().getHarvestLevel(holding, "pickaxe") <3) { speed.newSpeed = speed.originalSpeed/5; } } else { speed.newSpeed = speed.originalSpeed/5; } } } -
It breaks and gives the ore. And I have no idea why
-
[SOLVED] [1.7.10] Get all blocks touching each other
Failender replied to WARDOGSK93's topic in Modder Support
True that. set is way faster then an arraylist could ever be -
[SOLVED] [1.7.10] Get all blocks touching each other
Failender replied to WARDOGSK93's topic in Modder Support
I did something like this once, my solution was to use an arraylist and check with contains if the block is already included in my list, if not add it and check its neighbors.. this can get quite expensive really fast, so its only good if you are checking small amounts of blocks.. -
[1.8] PlayerEvent.HarvestCheck not fired when mining with tools
Failender replied to Failender's topic in Modder Support
Yeah I realized that.. Any other way to realize what I am triing to do? Making iron ore only harvestable with a tool that has miningLevel 3? -
[SOLVED] Issue setting blocks in world using World.setBlockState
Failender replied to Arkathorn's topic in Modder Support
What was causing your problem is the fact that you were setting the blocks on client side, so the player was unable to interact with them because they were not existing -
So I wanted to implement more tools, and making the way to iron a bit harder. Unfortunately Blocks.iron_ore.setHarvestLevel("pickaxe", 3) seemed to do nothing. So I tried using PlayerEvent.HarvestCheck to check if the block is iron and if it is check the harvestLevel. Problem is that HarvestCheck seems to not get fired when the player is harvesting with tools.. So I am out of ideas how to realize that iron is harder to mine. Any experiences? Greetz Failender
-
onItemRightClick behaving weird [ISSUE UPDATED]
Failender replied to zedlander1000's topic in Modder Support
ur welcome -
onItemRightClick behaving weird [ISSUE UPDATED]
Failender replied to zedlander1000's topic in Modder Support
not really. since you are triing to teleport the player calling methods from the player, and I am using methods from the NetHandlerPlayServer, holded by the player (I forgot to mention that I casted the player to EntityPlayerMP) -
onItemRightClick behaving weird [ISSUE UPDATED]
Failender replied to zedlander1000's topic in Modder Support
Which version? Because this works for me in 1.8 teleporter.playerNetServerHandler.setPlayerLocation (player.posX, player.posY, player.posZ, player.rotationYaw, player.rotationPitch); Where teleporter is the player to get teleported, and player is the player that he gets teleported to -
package de.failender.basewars; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.Mod.Instance; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.event.FMLServerStartingEvent; import net.minecraftforge.fml.common.network.NetworkRegistry; import net.minecraftforge.fml.common.network.simpleimpl.SimpleNetworkWrapper; import net.minecraftforge.fml.common.registry.GameRegistry; import de.failender.basewars.items.Modules; import de.failender.basewars.network.NetworkHandler; import de.failender.basewars.proxies.CommonProxy; import de.failender.basewars.tileentities.TileEntityBase; import de.failender.basewars.util.BaseHandler; import de.failender.basewars.util.BaseUtils; import de.failender.basewars.util.GuiHandler; @Mod(version=BaseWars.VERSION, name=BaseWars.NAME , modid = BaseWars.MODID) public class BaseWars { public static final String NAME ="Base Wars"; public static final String VERSION ="0.1"; public static final String MODID = "basewars"; public static final short BASEGUIID = 0; public static final short FURNACESID=2; public static final short BASEFURNACEID=1; public static CreativeTabs baseWarTab = new CreativeTabs("basewartab") { @Override public Item getTabIconItem() { return Item.getItemFromBlock(BaseWarBlocks.base); } }; @SidedProxy(clientSide="de.failender.basewars.proxies.ClientProxy", serverSide="de.failender.basewars.proxies.CommonProxy") public static CommonProxy proxy; @Instance public static BaseWars instance; @EventHandler public void preInit(FMLPreInitializationEvent event) { NetworkHandler.initialize(); } @EventHandler public void Init(FMLInitializationEvent event) { BaseWarBlocks.register(); BaseWarItems.register(); Modules.finishStartup(); GameRegistry.registerTileEntity(TileEntityBase.class, "tileentitybase"); BaseUtils.RemoveRecipe(new ItemStack(Items.stone_pickaxe)); GameRegistry.addShapedRecipe(new ItemStack(Items.stone_pickaxe), "TTT"," S "," S ", 'S', Items.stick, 'T', Blocks.stone); Blocks.iron_ore.setHarvestLevel("pickaxe", 3); System.out.println(Blocks.iron_ore.getHarvestLevel(Blocks.iron_ore.getDefaultState())); proxy.registerRenderer(); NetworkRegistry.INSTANCE.registerGuiHandler(instance, new GuiHandler()); MinecraftForge.EVENT_BUS.register(new BaseHandler()); } @EventHandler public void postInit(FMLPostInitializationEvent event) { } }
-
Hello everyone, my problem right now is that I simply want to change the tool u need to harvest iron ore. So normally you need a stone pickaxe to mine iron and you are good to go. I want to add some more tools, and decided to set the harvestLevel of iron to 3. Which means that normally you should not be able to mine it with stone. Bad news: I can still mine it with stone. Am I missing something totally retarded? Part of my Init method, and yes it is beeing called, since a syso on getHarvestTool gives me pickaxe and getHarvestLevel gets me 3. Blocks.iron_ore.setHarvestLevel("pickaxe", 3);
-
[1.7.10] Rendering .obj models as Armor HELP
Failender replied to BaXMultigaming's topic in Modder Support
we are not here to do your coding. -
I think the best example for particles is the vanilla furnace, just take a look at the BlockFurnace from vanilla and you might find ur solution there
-
elix is right with that. if u think a bit about what static means in java ull know
-
I do this because like a machine that can access several furnaces. Like an overview of all your furnaces. That why I want to send the data to client so it can display it in the gui
-
I am triing to make a custom furnace, which is like the vanilla furnace, just with some additional properties. Since most of the stuff is like vanilla furnaces I created a TE extending TileEntityFurnace, a Block extended BlockFurnace and fixxed my .json files. I am saving the locations of placed furnaces in a TileEntity, called TileEntityBase. At one point I need to sync the placed furnaces between Client and Server Side, so I am using a package. The problem is that it seems that my custom block is creating a TileEntityFurnace, so I am getting a ClassCastException here int i = 0; for(BlockPos location : message.furnaceLocations) { furnaces[i] = (TileEntityBaseFurnace) worldObj.getTileEntity(location); i++; } The locations are beeing send via the message, and these are the correct positions, I checked that. So it seems that at some point minecraft seems to kill my custom TileEntity and replace it with a TileEntityFurnace. But the only place where I can see Minecraft creating a TE is at @Override public TileEntity createNewTileEntity(World worldIn, int meta) { return new TileEntityBaseFurnace(); } Am I missing something?
-
show your assets folder structure please
-
First of all, if you run this on a server this will crash it. Registering Renderer should ONLY happen on client side, thats why you should use proxies to do so. Show your folder structure in assets with the files please
-
[1.8] Getting a worldObj in TileEntity.readFromNBT
Failender replied to Failender's topic in Modder Support
First of all, thank you for the information larsgerrits. Second I just realized that I am totally dumb, since tileEntites have a worldObj inside them -
[1.8] Metadata limited to 4 bits - possible alternatives
Failender replied to Reygok's topic in Modder Support
I dont know that much about setting textures in 1.8 via code, except of basic metadata with 16 variants, but one way might to save which texture you want to use in a tileEntity. Of course this only a good idea if your block isnt common, because TE's are quite expensive. One thing you might do is create 12 Block with 12 states. Maybe you can categorize your block a bit more, like chisel mod -
Hello everyone, I am triing to safe another TileEntity inside another. I do this because I need a reference to "drain energy" from it. I am doing it this way because I am building a mod which based on each player having his own base, which is storing all the stuff like energy. That means that each "machine" needs a reference to the base to drain energy from it. So I thought about setting the TileEntity and saving it. Problem is if I want to read it I need a worldObj to get the TileEntity from at a saved BlockPos. To sum things up: I need to get a worldObj on client and server side. Client side should be easy, use Minecraft.getMinecraft().thePlayer. For Server I have no idea. Also I need a way to find out if I am on Server or Client Side, because otherway it will crash when I try to access Minecraft.getMinecraft. Of course one way would be a try catch around Minecraft.getMinecraft , catching the ClassNotFoundException, but that feels shitty..
-
Well. You use that method and set your own variable to true? Of course it only works for custom blocks and you have to use nbt (except of if you are using a hashmap or sth full of booleans)
-
Use onBlockPlacedBy(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase placer, ItemStack stack) {} and check if placer is an instace of EntityPlayer
-
[1.7.10][SOLVED Finally]Tile Entity Questions
Failender replied to BuddingDev's topic in Modder Support
Read and write nbt is only for saving data, like when the game is shutting down. It gets called by Minecraft ,no need to do it manually. What exactly do you mean with acting strange? TE's execute on Server and client side